Next Lesson: Lesson 22: While Loops in Python
We’ve learned about functional programming in the previous lessons. Now, let’s discuss Object-Oriented Programming (Classes in Python).
Functional Programming and Object-Oriented Programming
Before we get to our lesson, I just want to make some things clear. Functional Programming is, as its name hints, about using functions and we’ve talked about this type of programming in Python on Lesson 9.
But in this lesson, we are going to talk about Object-oriented Programming. This type of programming stresses on objects. What is an object? An object is simply a collection of data (variables) and methods (functions) that act on those data.
We both need these two types of programming in Python. And I hope you have understood the whole flow for the Functional Programming because Object-oriented Programming can include this to itself.
Alright! Enough talk, let’s code! Create a new file: ex20.py. Of course, type this in:
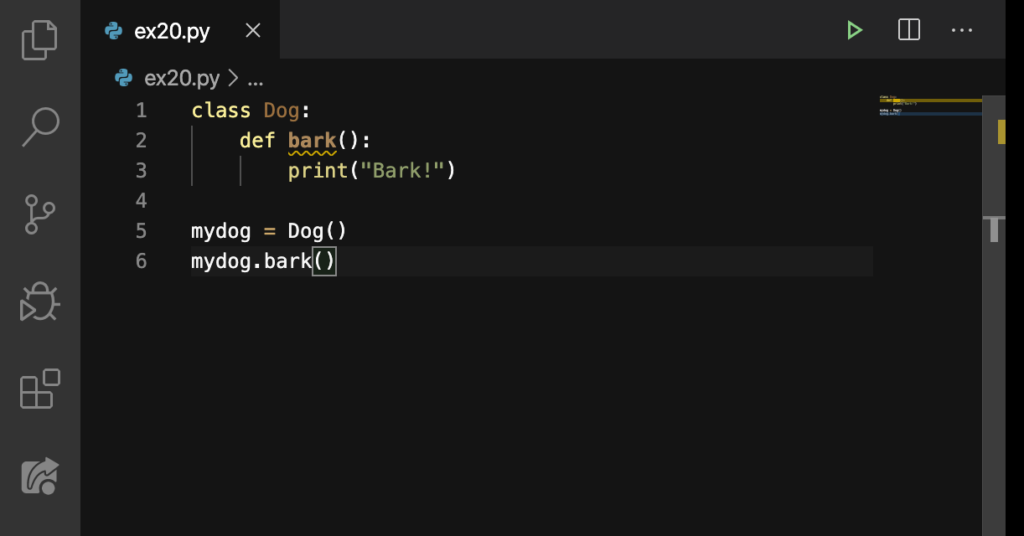
To make a class, just type class and then give your class a name. On our case, we have Dog as our class. Starting your classname in an uppercase is not required, but it is suggested. Finally, we don’t want to forget about the colon (:).
What can we put inside a class? Well, a function would be very nice to put inside a class. And then we’ve defined a function by saying def; this function will help Dog “Bark!” So now, our Dog has a function or method bark.
Additionally, we can create an object from this class. To do that, we set our object Dog() to a variable mydog. Now, we have an object.Then we tell mydog to bark by doing what we did on Line 6.
Looks like we can now run this on our Terminal. But:
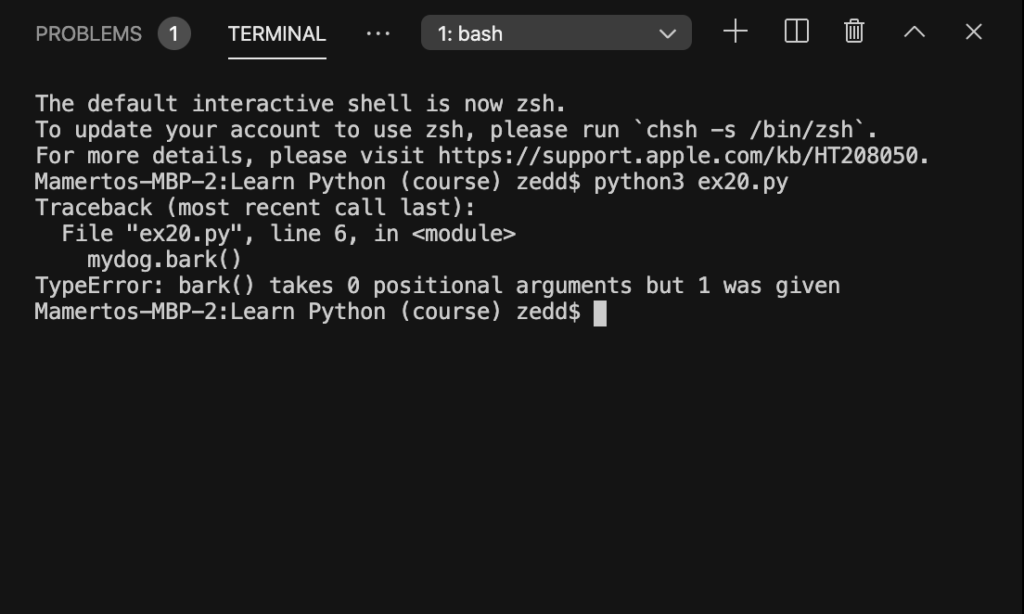
This will not work because we should have self as a parameter to our function. So let’s add it in:
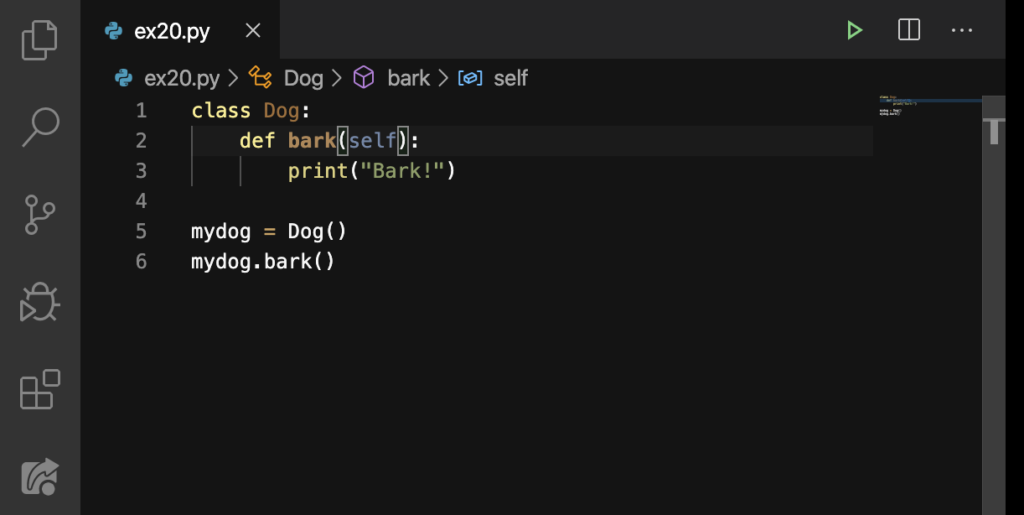
Note: The self is a parameter; the mydog is an object. That’s why we need that self.
Let’s run this:
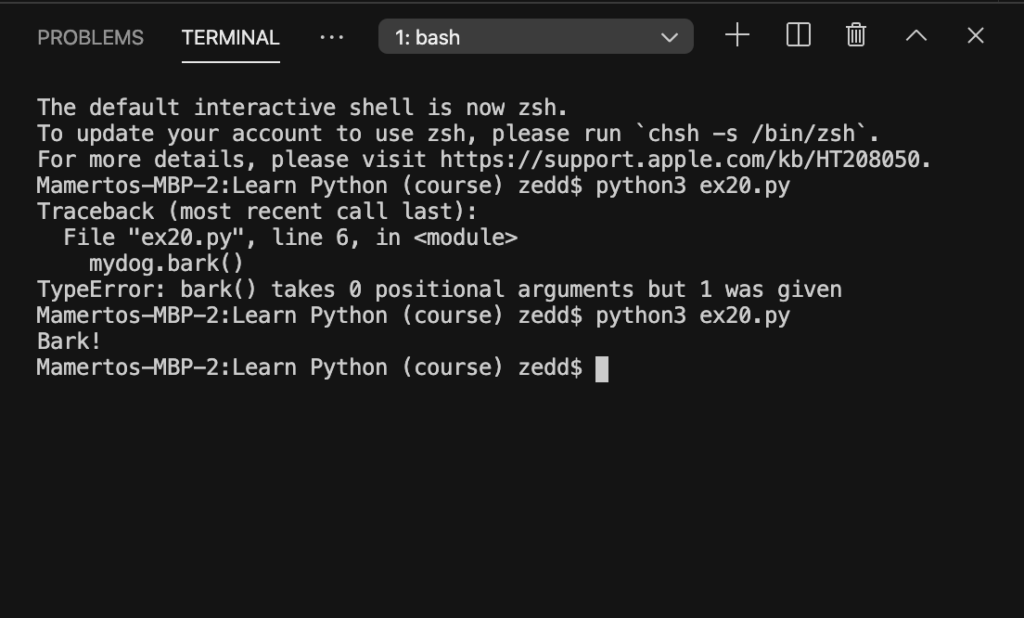
Yes! Our Dog finally barked! But let’s give our Dog more instance1An individual object of a certain class.. And we’ll give it something more than just the ability of barking. I know we’ve talked about many terminologies here so I’m giving you a reference to help you with that.
More instance variables
We can make instance variables2Variables that are defined inside a method and belong only to the current instance of a class. on the flight of coding. Let’s add some. Make sure that your code looks exactly like this:
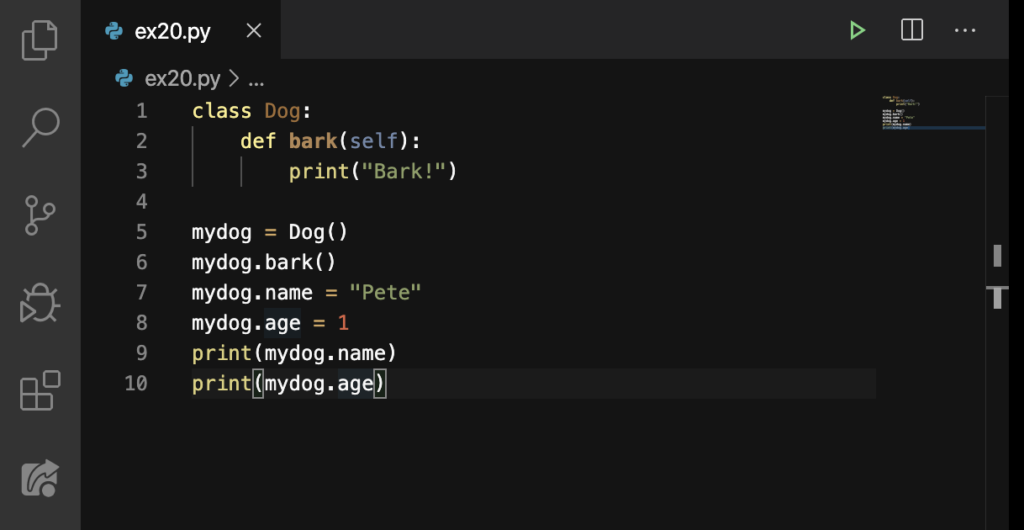
So, our Dog now has a name (Pete) and an age (that’s 1 year old). And we also want these printed on our Terminal. Let’s run it:
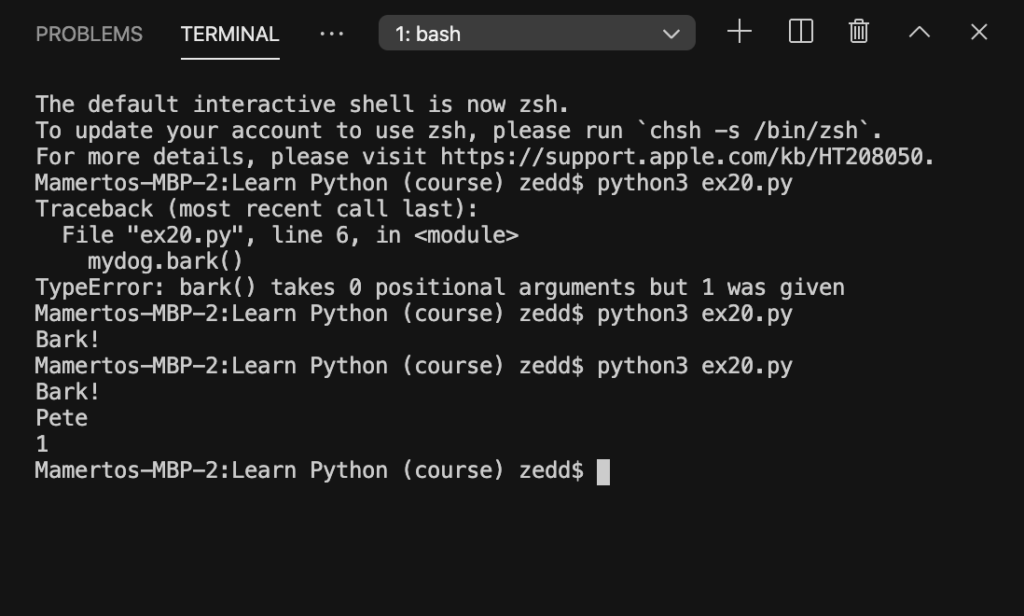
Epic!
Class variable
Let’s make a class variable and name it dogInfo:
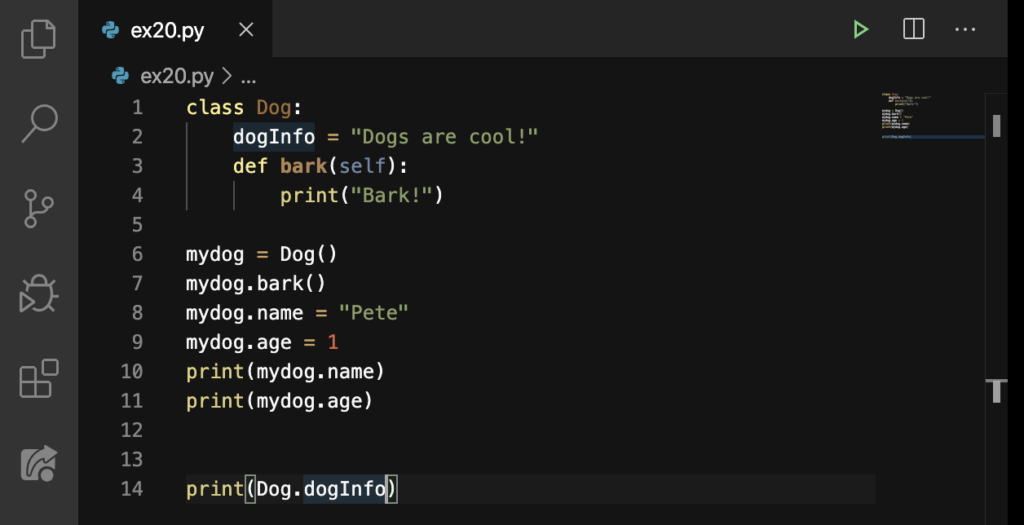
This class variable can be accessed anywhere–it’s not tied to a specific instance. So for example, after all this code and we could go down to Line 14 and say print. Of course, we needed to specify what we need to print out by saying the name of our class first (Dog), and then typing in the name of that variable after a dot.
Let’s check this out:
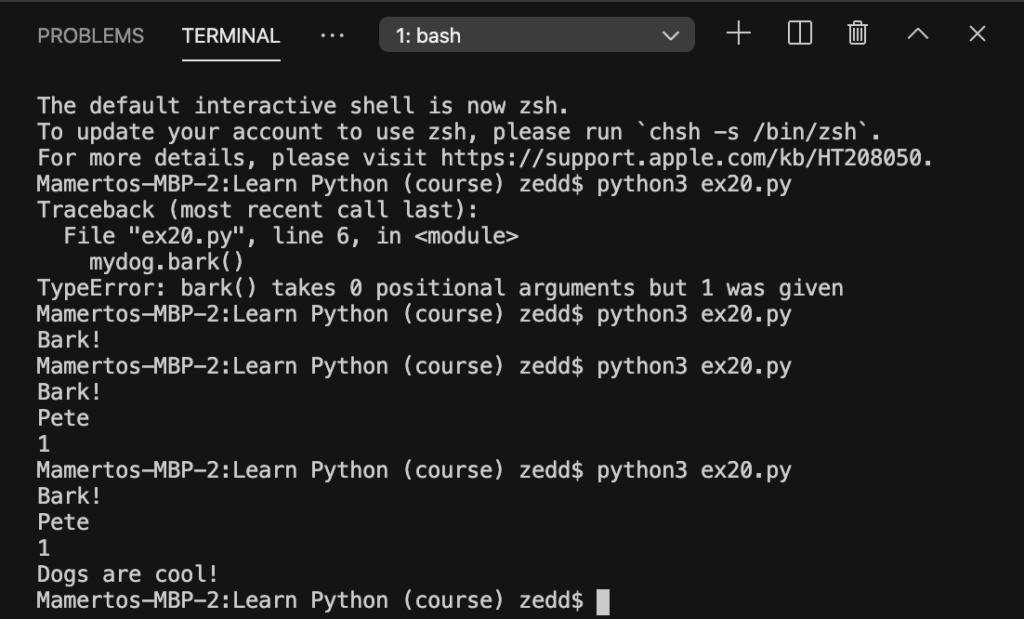
Cool. If you’d want to you could replace the value of our class variable here on Line 13 right before we print it out:
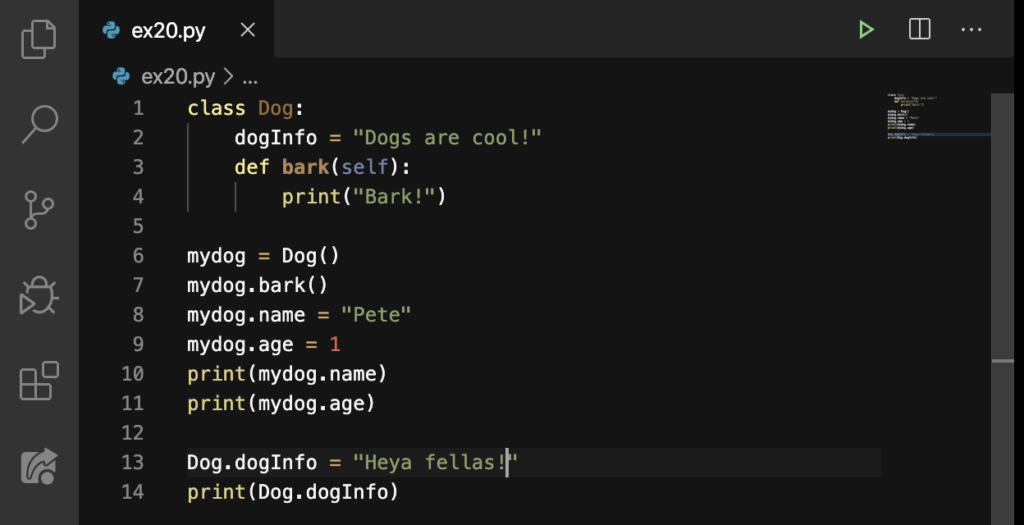
Will this work? Let’s see:
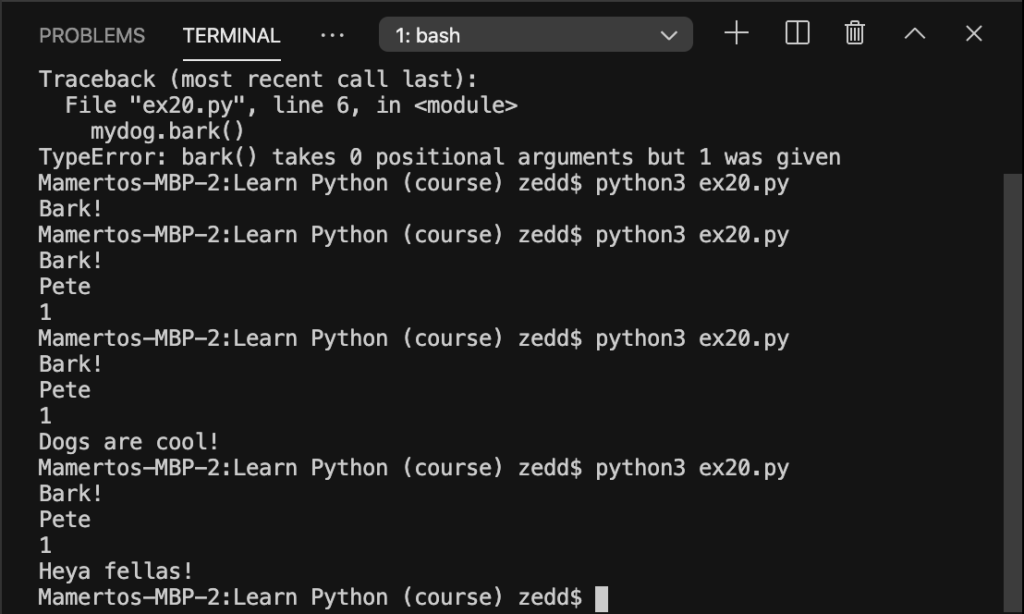
Nice.
Another parameter
Instead of having just self as our parameter, we can have a second parameter:

We added hotdog as the second parameter and had the ” I want a hotdog!” on Line 7 as its argument, which we’ve added on “Bark!”. Let’s print it out:
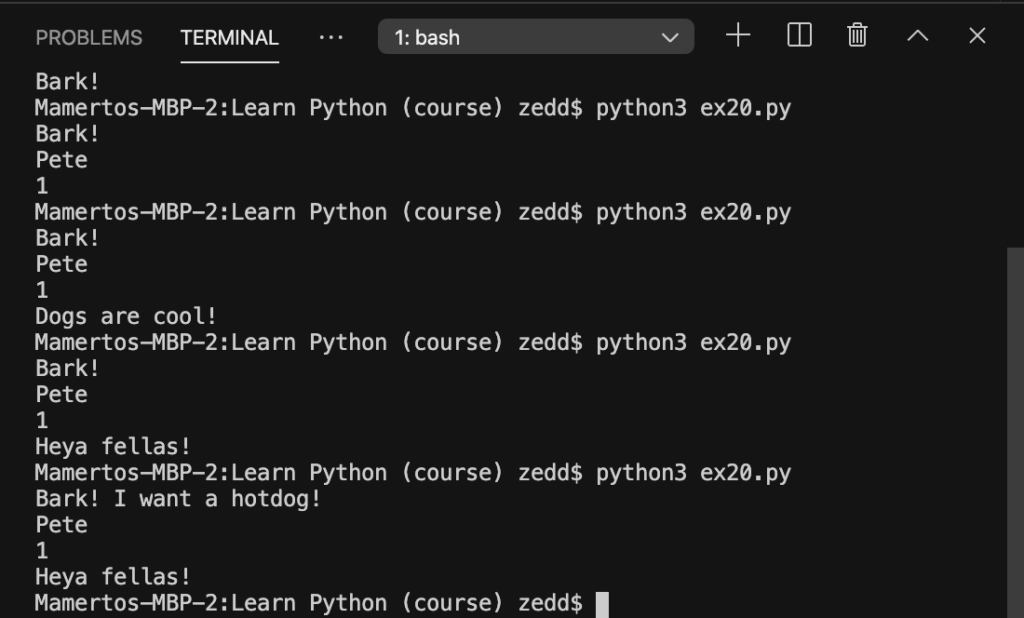
Want a hotdog? Go to foodpanda and go buy some. Once you buy some, don’t forget to share some with us.
__init__
All along, we were just being at the basics of classes in Python. But before we get into the discussion for this __init__, first make your code look like this:
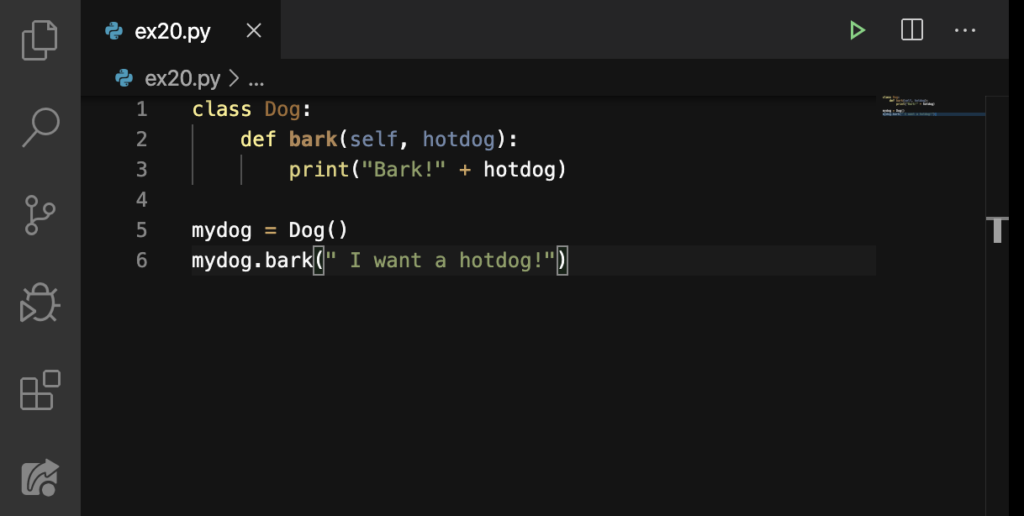
Neat! Let’s check to see if it still works:

Good. When making a class, we should give it some instances or characteristics. On our example, Dog, we can give it a name, an age, and a fur color like an ordinary dog.
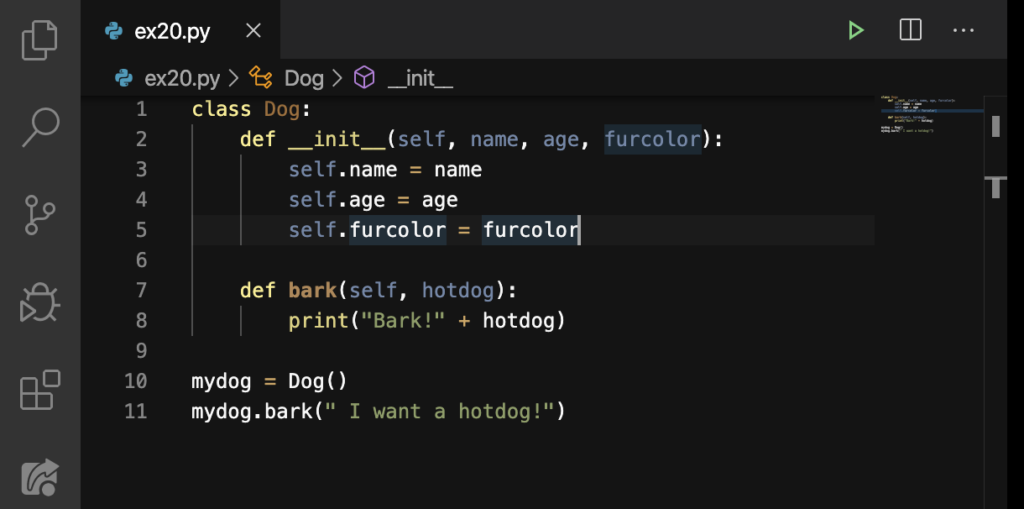
To do the one on Line 2 correctly, we should type in two underscores (__ [with no space in between]). Then the word init itself and finally, another two underscores. Inside this init function, we have self as our first parameter, and then followed by the other parameters.
We’ll discuss the other code lines later because there is something wrong with this code:

And our Terminal says that our __init__ function is missing 3 arguments for the parameters name, age, and furcolor. So let’s give these their own arguments:
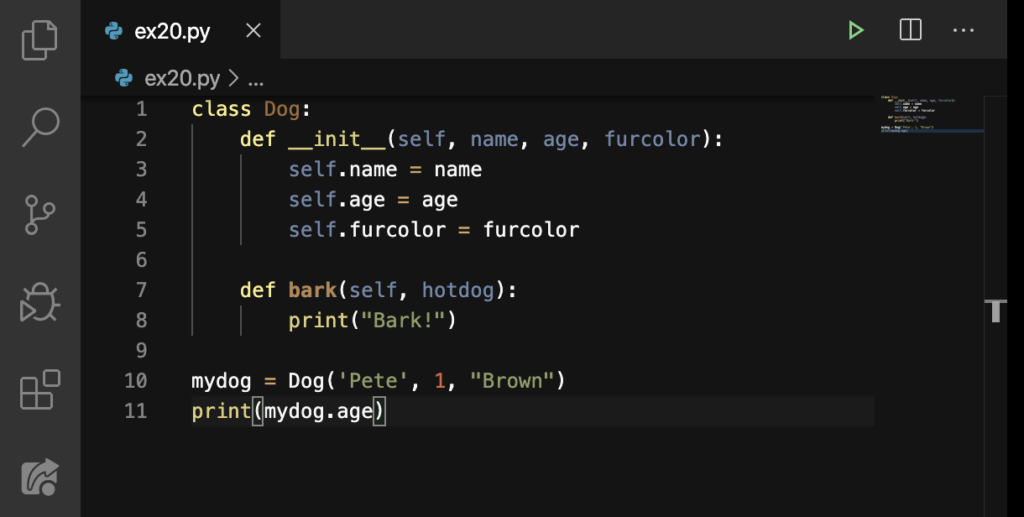
On Line 3, we assigned the value of the parameter name to name.
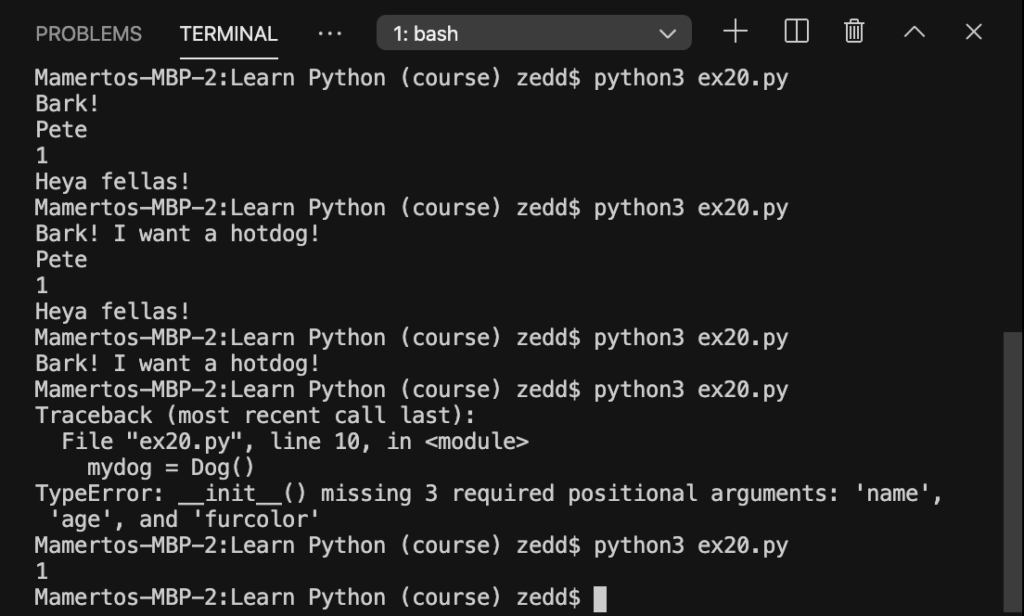
self
Just want to make it clear that self has nothing special for our code, we’ll have some changes:
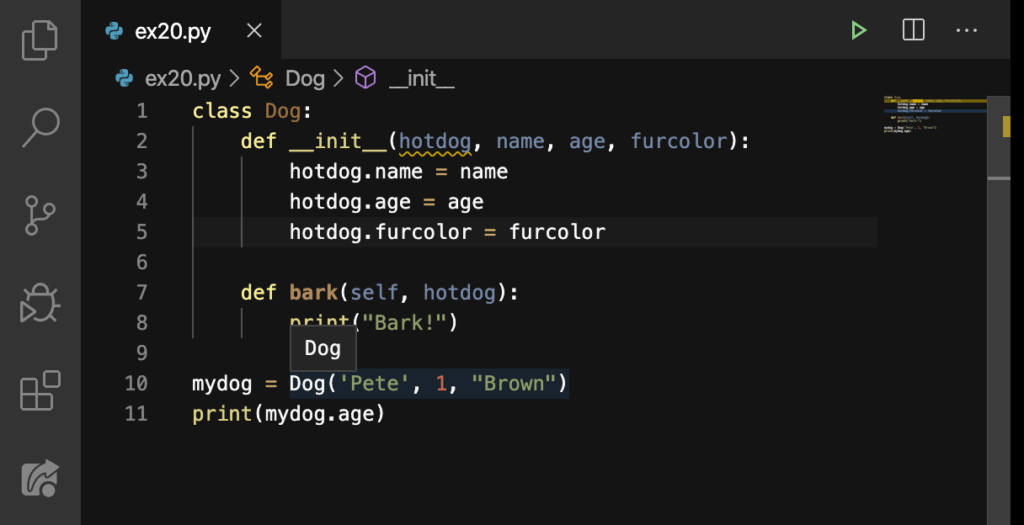
So here I’ve changed self into hotdog. However, you have to remember that once you’ve changed one self, you would also have to change all selfs to avoid error. Run:
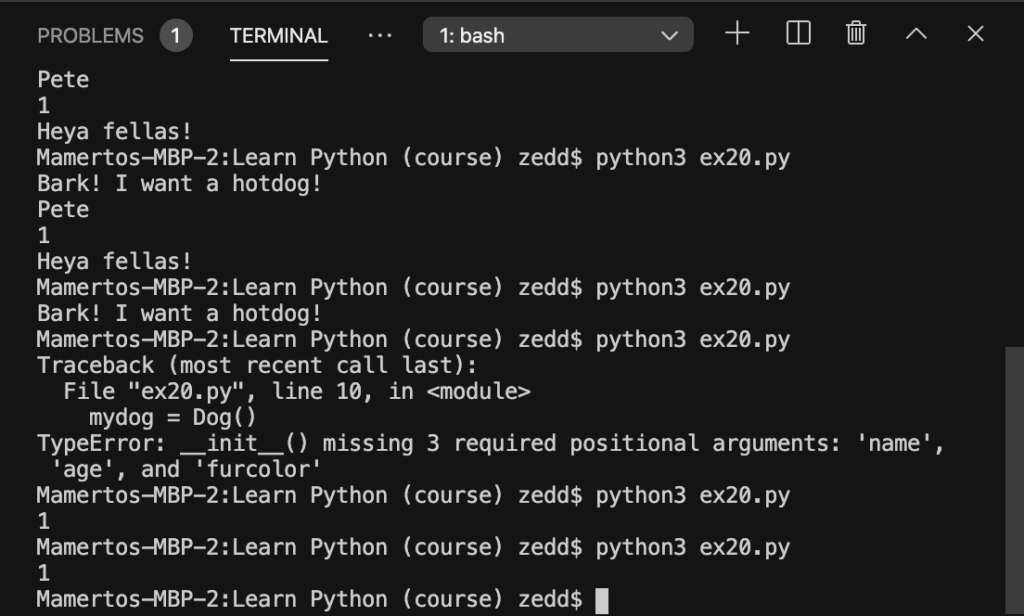
See? You can also change it to cheesedog.
default value
Just like what we’ve learned from Lesson 10, we can set default values to our parameters for classes in Python, just in case we forgot to set the arguments. Type this:
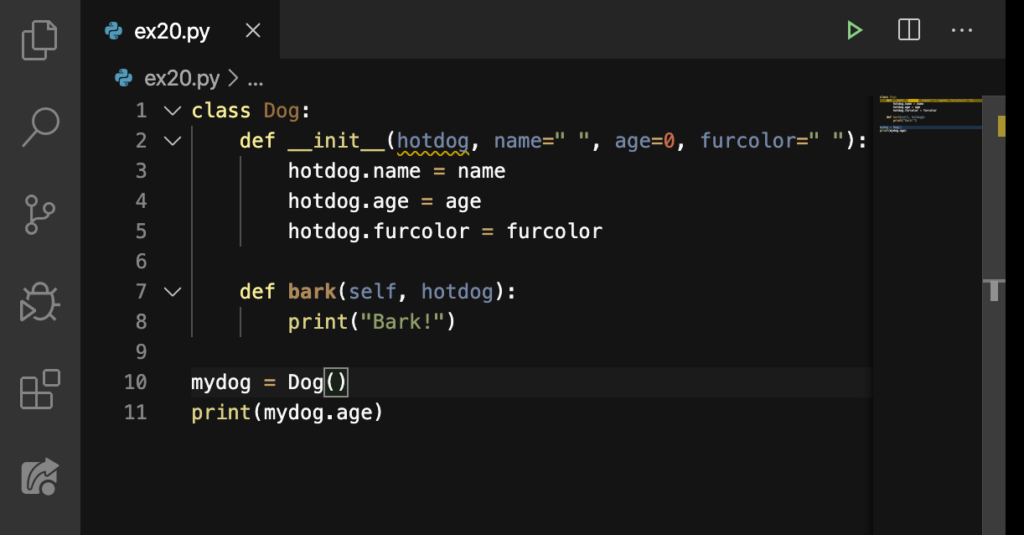
Notice that I’ve removed the arguments from Line 10. Let’s run this:
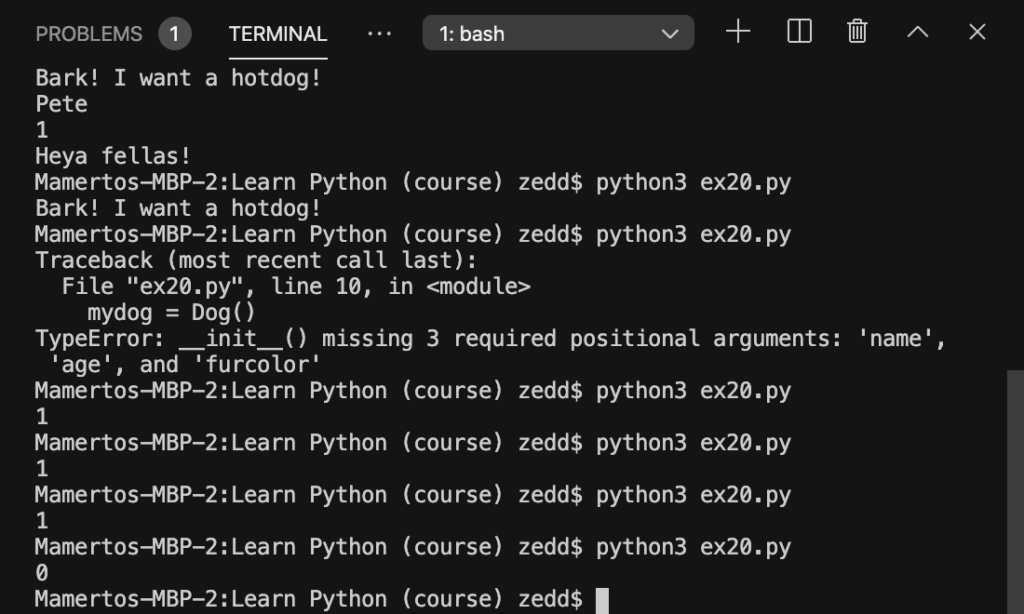
That’s it for classes in Python! I know that this lesson may have been a little tough for you. But if you have questions, just post them down on the Comments section below and we’ll talk about it. See you on the next lesson!