Previous Lesson: Lesson 17: Mutableness, Errors, and Methods in Dictionaries
Know how immutable tuples in Python are, and why they are unique compared to lists and dictionaries. I also made a cheatsheet for you in this lesson.
Create a new file named ex15.py and type this in:
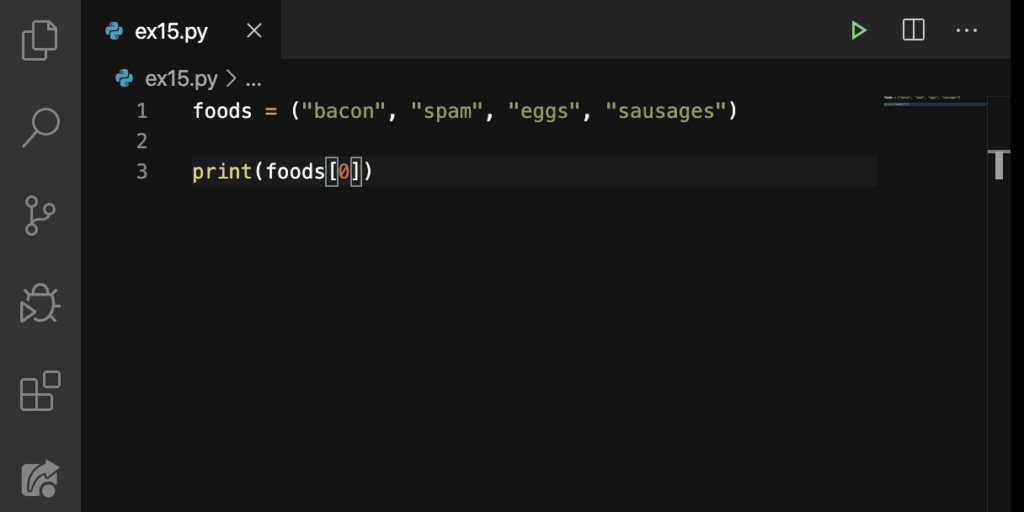
Like lists, we can index an element out of a tuple, too.
Note: We create lists using square brackets ([]); we create tuples using parentheses (()).
Let’s get that favorite food of mine:
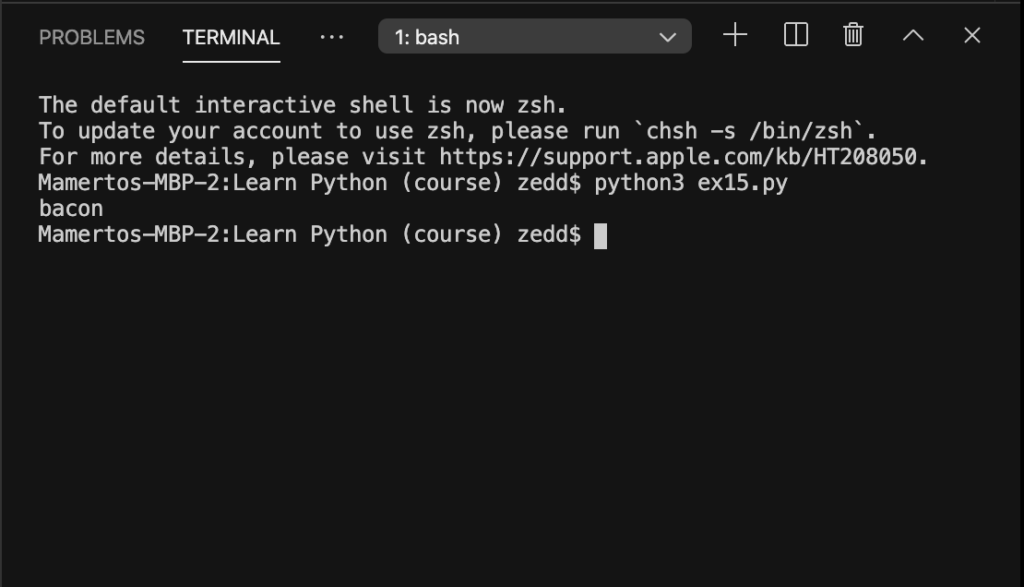
Yummy!
Reassigning an Element of a Tuple
Tuples in Python are very similar to lists. But unlike lists, tuples are immutable, or cannot be changed. We’ve learned from the previous lesson that lists and dictionaries are both mutable or can be changed. But in the case of tuples, they can’t be changed. Let’s make an attempt of changing this one:
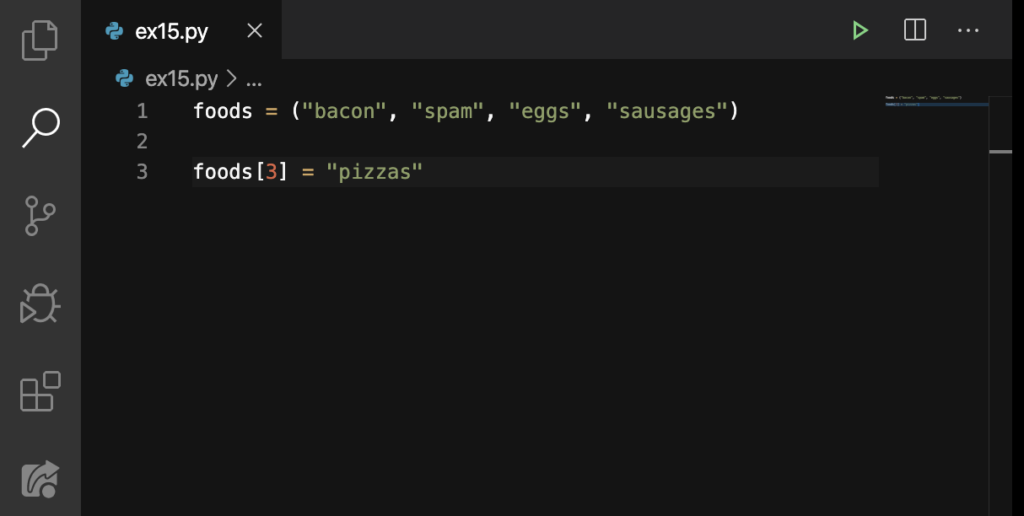
Run:
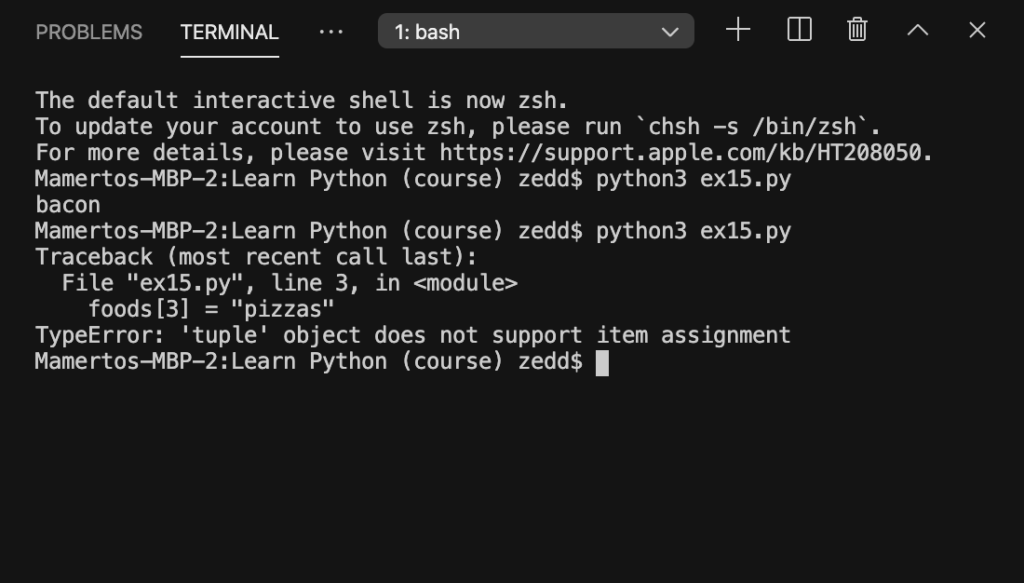
As what we can see in our Terminal, trying to reassign an element inside a tuple causes a TypeError. Therefore, it really is impossible to change this one. But I’ll tell you that tuples are awesome with this next one.
Tuples Without Parentheses!
Yes, they can be created without the parentheses (()). Remember, though, that when the type of an element inside the tuple is a string, we should still put quotation marks around them. For example:

Nonetheless, you may have notice this one too, that we don’t have to worry about putting quotation marks if we want an int or a Boolean as an element in our tuple. However, you can try and play around with this one for a while.
I’ll just get back to this code:
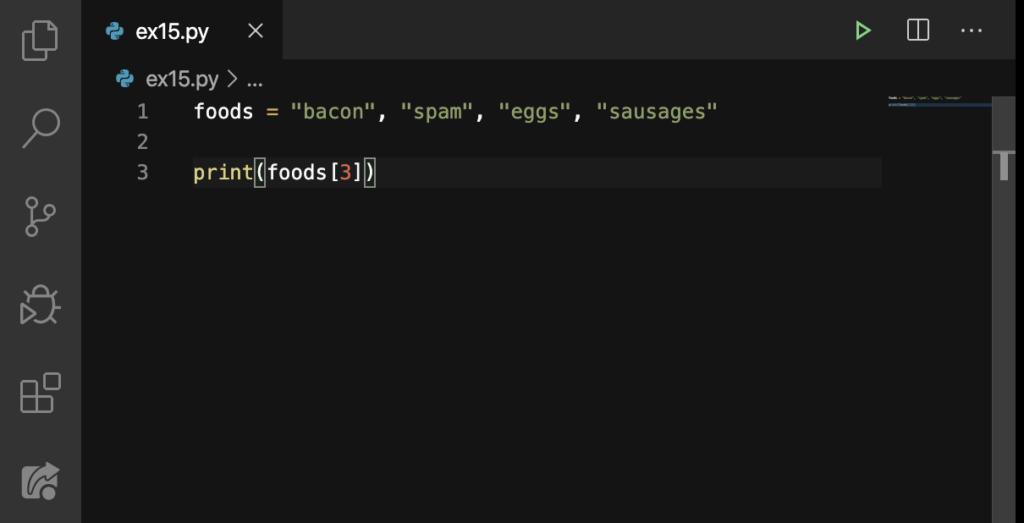
And then we’ll run this:
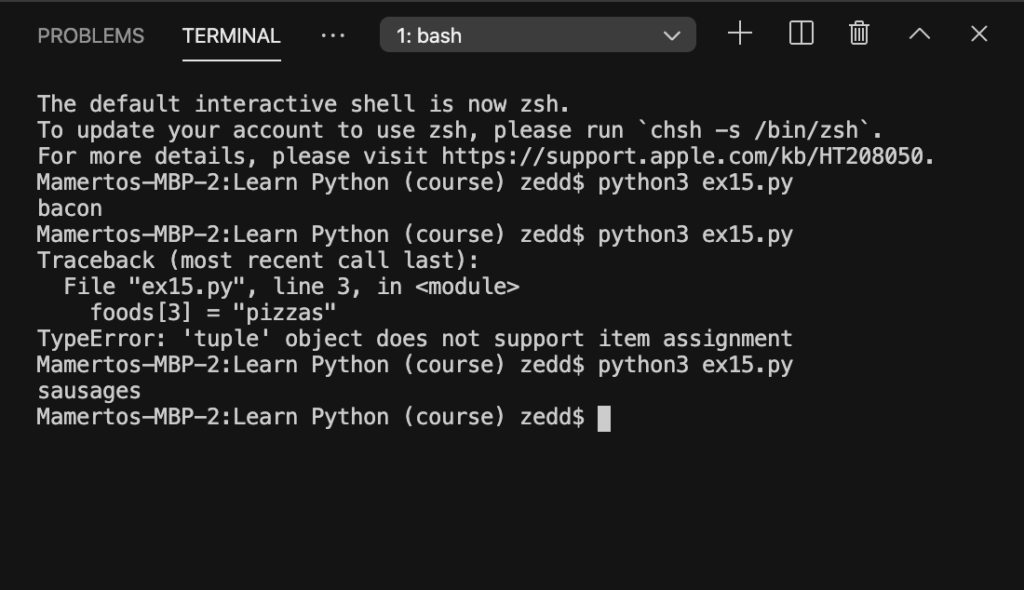
Alright! We’ve got the sausages. We can create as many tuples without parentheses as we want. Unless, if we want a nested tuple.
Nested Tuples
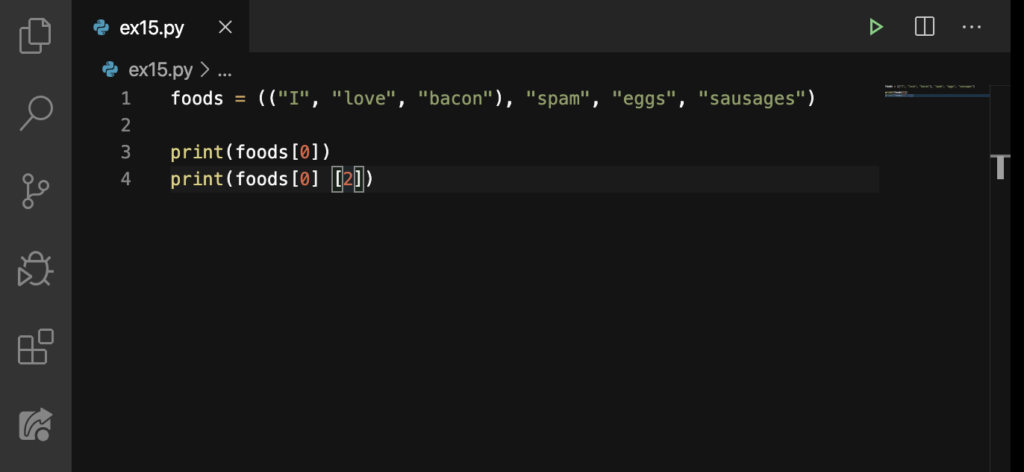
Like lists and dictionaries, tuples can be nested. We know what Line 3 does–it gets the first element of our tuple (which is also a tuple). And that is the (“I”, “love”, “bacon”).
Concerning how Line 4 works, I’m sure you’ll remember what we’ve discussed about accessing an element from a nested list back on Lesson 13 (Part I). And it works the same way.
Still, I’ll explain how the code works. We wanted to get the third element (by using [2]) from the first element (by using [0]) from our tuple foods. So that would be. . .

Yup, my favorite food again–bacon!
Tuples Inside a Dictionary
We’ve learned that only immutable objects can be used as keys on our dictionaries. And that includes a string, an integer, and a boolean. Additionally, we’ve learned from this lesson that tuples are immutable, too! So can you use them as keys for your dictionary? If yes, how will you do that? Let’s see:
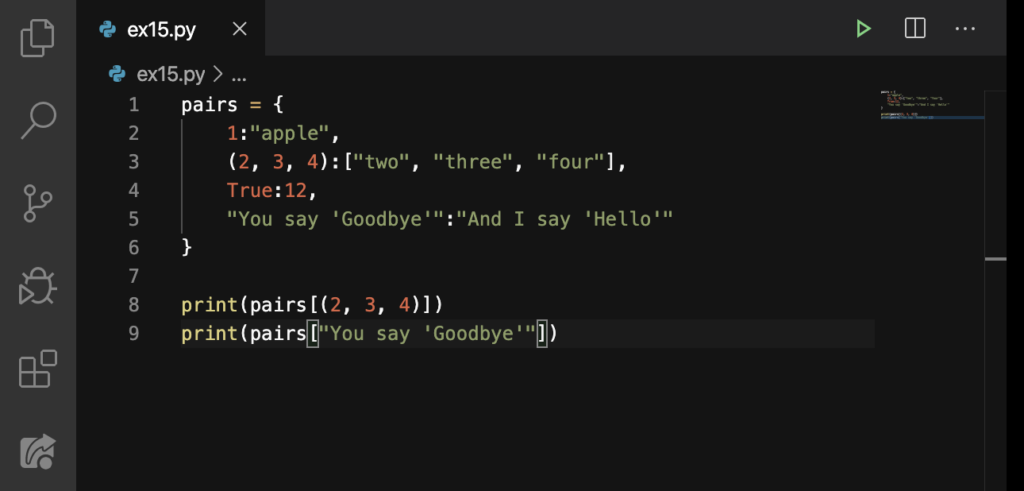
Note: Line 3 has our tuple as its key.
There should be no error for this one:
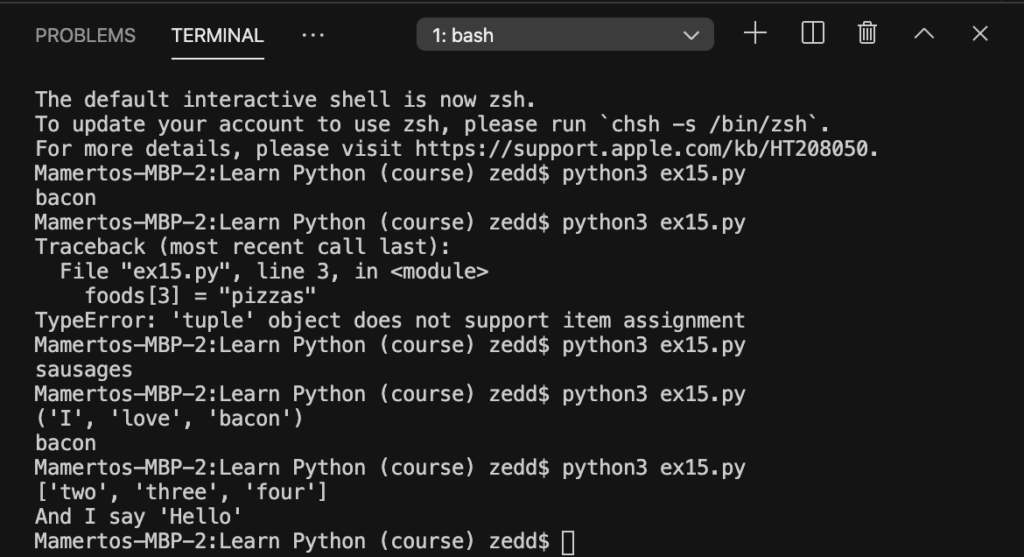
Good! So that’s it with tuples in Python! I hope you understood this lesson as you have understood the previous lessons! But, I know that you may be confused with all these lists, dictionaries, and tuples. So I wanna give you something.
A Cheatsheet!

Yeah! Go ahead and write this down on an index paper and always keep it with you. If you’re still confused with these, you’ll get used to it by practicing. Keep on practicing. And keep on playing around with our code where we used lists, dictionaries, and tuples. Doing so will help you get more familiar with each.
This is important to do before you move on to the next lesson. Why? Because on the next lesson, we’ll talk about something that we can add to our cheatsheet. See you there!