Previous Lesson: Lesson 16. Dictionaries in Python
What are “mutableness”, the errors, and the methods in dictionaries? How do we face the errors once we’ve encountered one? Learn all these in this lesson!
“Mutableness”
Alright. Before we discuss about what “mutableness”, the errors, and the methods in dictionaries in Python, I just want you to know that we are still gonna use ex14.py for this lesson. Additionally, I want you to clean your code, and make it look like this one here:

Finally, you’ll know what I meant when I said that “lists and dictionaries are mutable” is once you’ve run this on your Terminal:

See? Just as what the name of our dictionary suggests, this is a bad dictionary. So if we determine what kind of error we’re dealing with, it says TypeError:unhashable type: ‘list’. What’s that suppose to mean?
It only means that only immutable objects can be used as keys to dictionaries. Immutable objects are those that cannot be changed and lists and dictionaries are both mutable (which means, they can be changed). So, we can only use lists and dictionaries as values only, not as keys. Want to prove that they are mutable?
As we can recall what we have learned from lesson 13, we can use the .append method to add an element to the end of a list. We can also use .insert to insert an element anywhere inside a list. And we could also use del to delete an element from the list. Which means that we can do anything with lists. And not just that.
Have I told you that an element from a list can be changed entirely into anything that we want? Let’s try it. Type this all in exactly on your editor:

Let’s see if something really changes:

As you can see, we’ve changed the ‘4B’ from the pencils list into ‘3B’; ‘vinyl’ from the erasers list into ‘gum’; and ‘A4’ from the papers list into ‘letter’. This also works for dictionaries. This one looks a lot like the first one on top so type this one carefully:
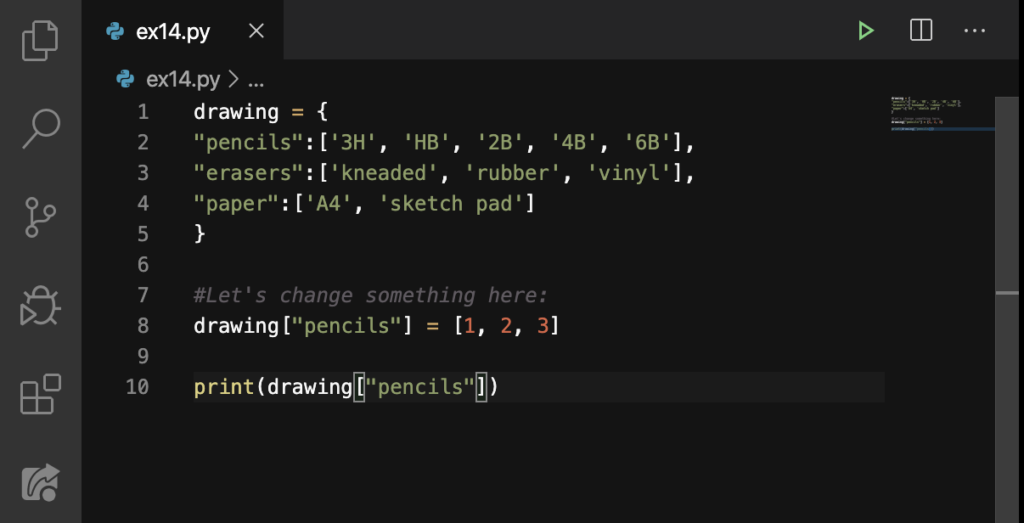
Let’s change the ‘3H’, ‘HB’ and whatsoever (they are called “lead grade swatches“) from the “pencils” key into [1, 2, 3]:

Nice work. We’ve talked about what TypeError means. But now, let’s talk about another error that we may find.
Key Error
Trying to index a key that’s isn’t part of a dictionary returns a KeyError. Let’s try by typing this in:
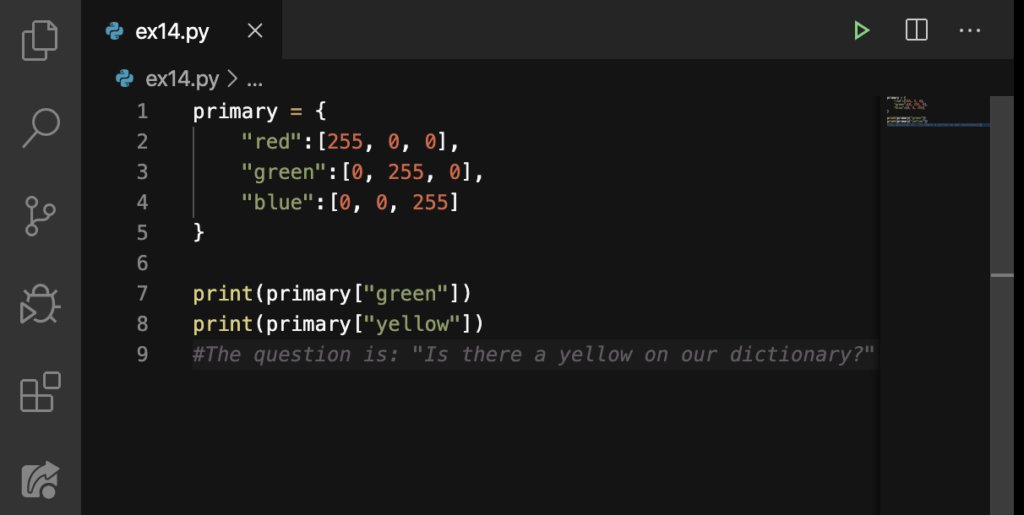
Now let’s run this:
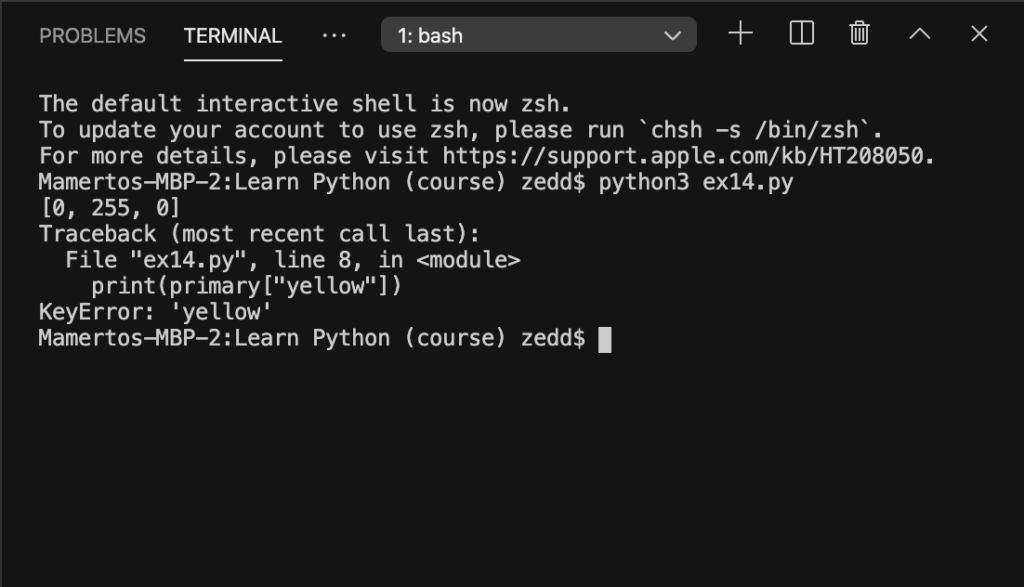
To face this kind of error, you can simply make “yellow” a key and then assign a value to it. Or, you could just simply get a different key that exists in your dictionary.
‘in’ and ‘not in’
This is also what we’ve learned from lesson 12. But this time, we’re applying it to dictionaries. Still, just to have a review, we can determine whether a key is in a dictionary using the in and not in. And we use these like this:
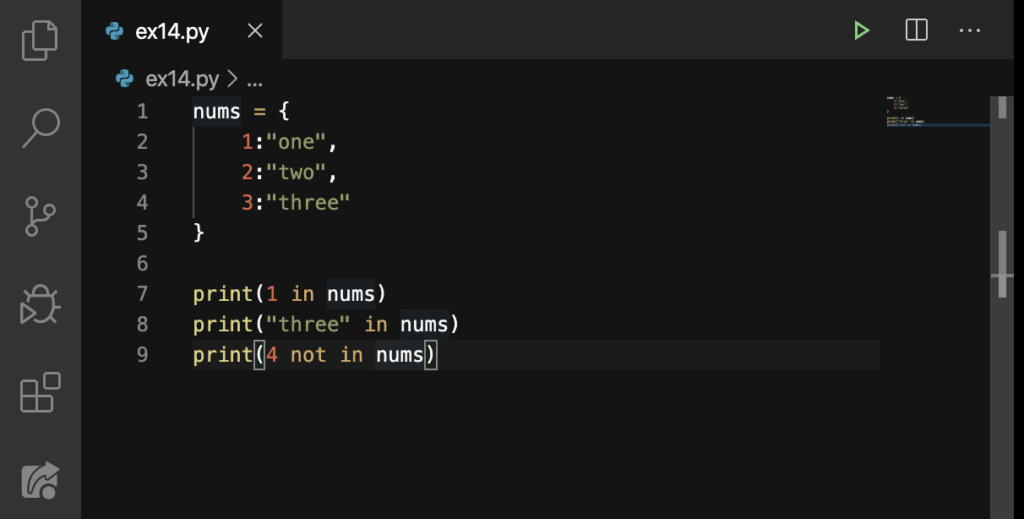
Run:

We got True because 1 is in our nums list. Then we got False because “three” is not in our nums list. And then finally, we got True because 4 is not really in our nums list.
The .get Method in Dictionaries
Because it has the dot (.), it is a method. What does it do? Well, it does the same thing as indexing. But then, it has something different that’s also amazing at the same time. If the key is not found in the dictionary, it returns another specified value instead. But if there is no specified value, the None get’s printed (it is the default). Type this in:

You may notice that instead of using square brackets ([]), we are using parentheses (()). This is important to remember when using methods and that includes .get. But how do we understand all these?
Do we have the “orange” key? Yes, we have. So [2, 3, 4] will get printed out. But do we have 7 as a key? No, we don’t have a 7. But since it doesn’t have a specified value, it will return None.
Line 10, is a little more intriguing. Do we have 12345 as a key on our dictionary? No, we don’t have that. But instead of simply returning a None, because we don’t have that key, “not in dictionary” will get printed out. Watch this:
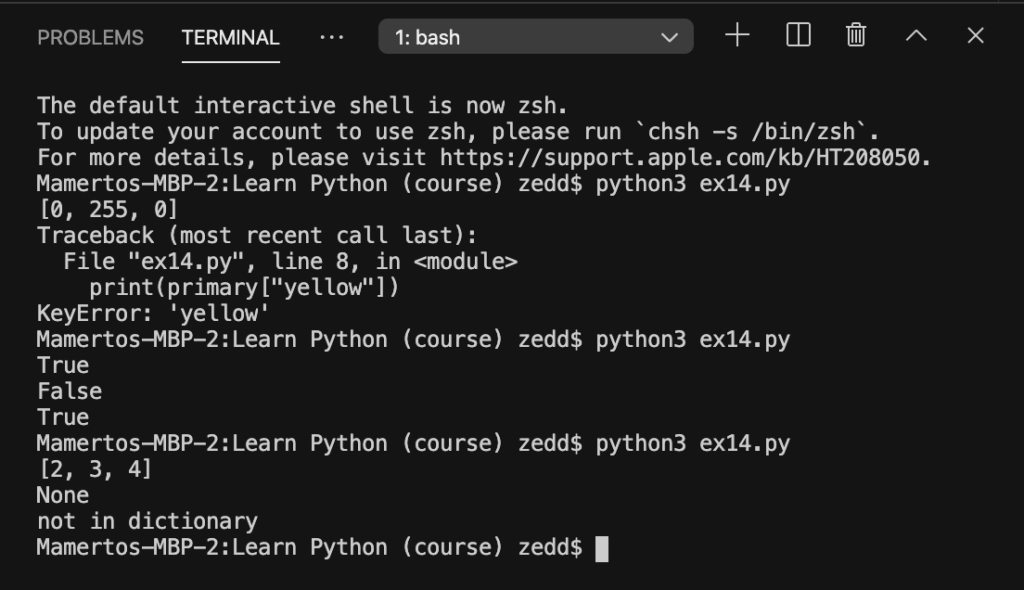
Yay! It worked! Let’s add some more to get the hang of it:

Hopefully, you know how this runs. If you do, share it on the Comments section below. If you don’t, you’re always welcome to ask down below, too and we’ll try our best to answer your question(s). Anyway, let’s still run this:

Good going! So that’s all about “mutableness”, errors, and methods in dictionaries! On the next lesson, we’ll talk about something that’s like a lists and dictionaries. But the main difference current topic (which are mutable) to our next one is its immutableness.