Previous Lesson: Lesson 13. (Part I) Accessing and Nesting on Lists in Python
Once you’ve finished this lesson, you’ll learn about and know how to use some of the different methods and functions on lists in Python. Let’s still have this in the file we’ve created and used in our previous lesson: ex11.py.
The len function
Want to know how many items we have in a list? We can use len. And as you might have guessed, this is a shortened wording for “length.”
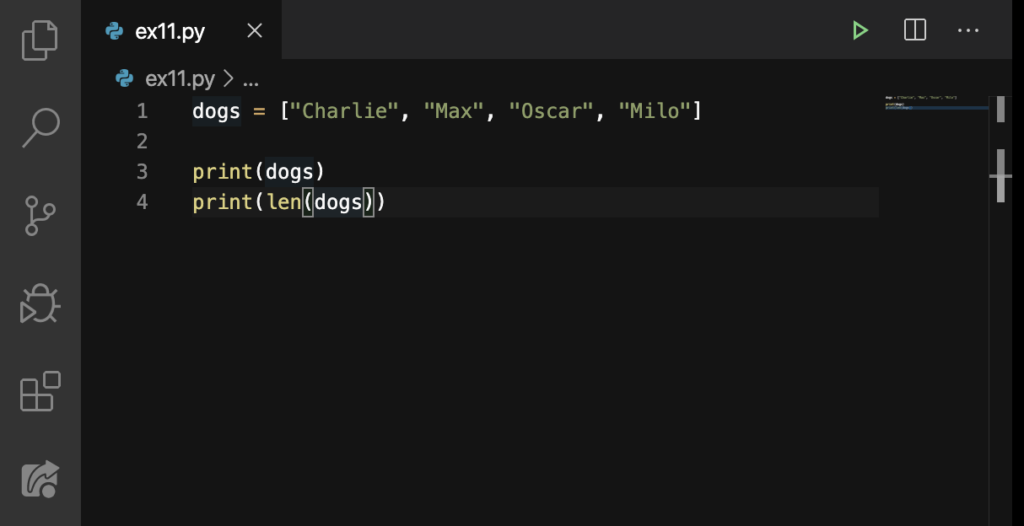
Let’s check:
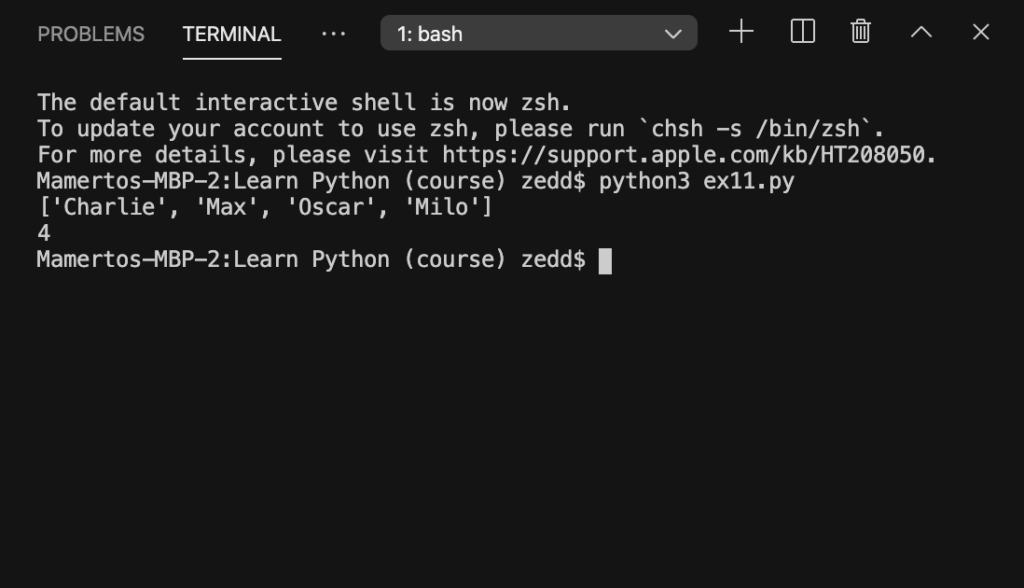
Great! It tells us that we have 5 items or elements inside our list. You may notice that len does not have a dot. That’s because it is a function, not a method. From now on, bear this in your mind: If it has a dot, it’s a method; if it doesn’t have a dot, it’s a function.
The .append method
So, is this a method, or a function? Right, .append has a dot, so that means it is a method. What’s this method for? As the word hints, append means to add an additional item or element to the end of an existing list. We apply this method by doing this:

So we’ve written down the name of our list, dogs. And then we attached the method .append. Afterwards, we’ve put the element we want to append to the list, which is “Jane”. So let’s see what this looks like:
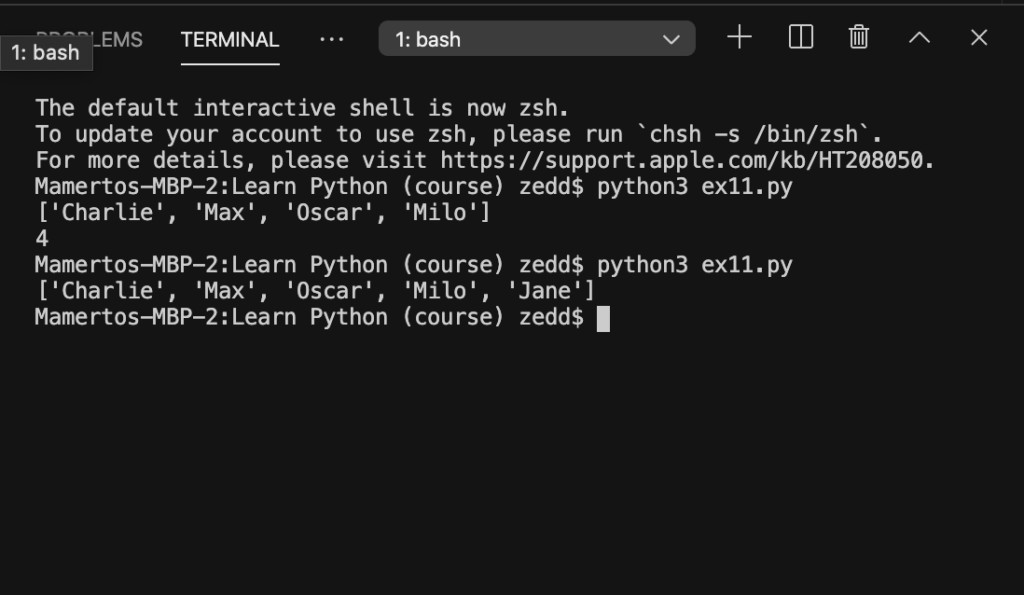
Nice work! But what if we want to insert a new element somewhere in the middle of our list?
The .insert method
It was fun doing things with our examples. But for now, we’ll try this method on a different example:
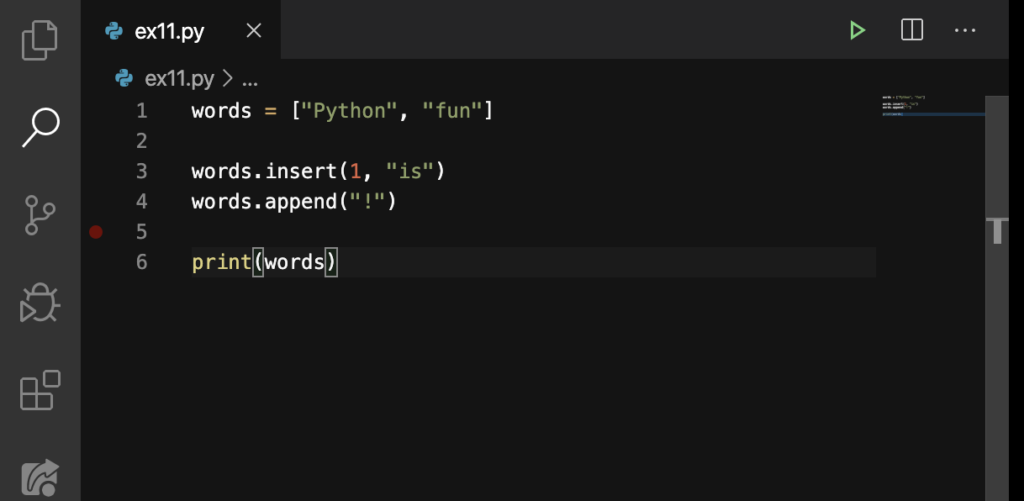
So we can understand what’s on our Line 1 and so is what we have done on Line 4 because we’ve just talked about how we use .append. Using the .insert is a little similar with .append. However, to use .insert, we first say the index (which is the 1) of where we would want to insert the “is” inside our list (which we’ve specified after the comma).
All in all, let’s just see how this looks like on our Terminal:
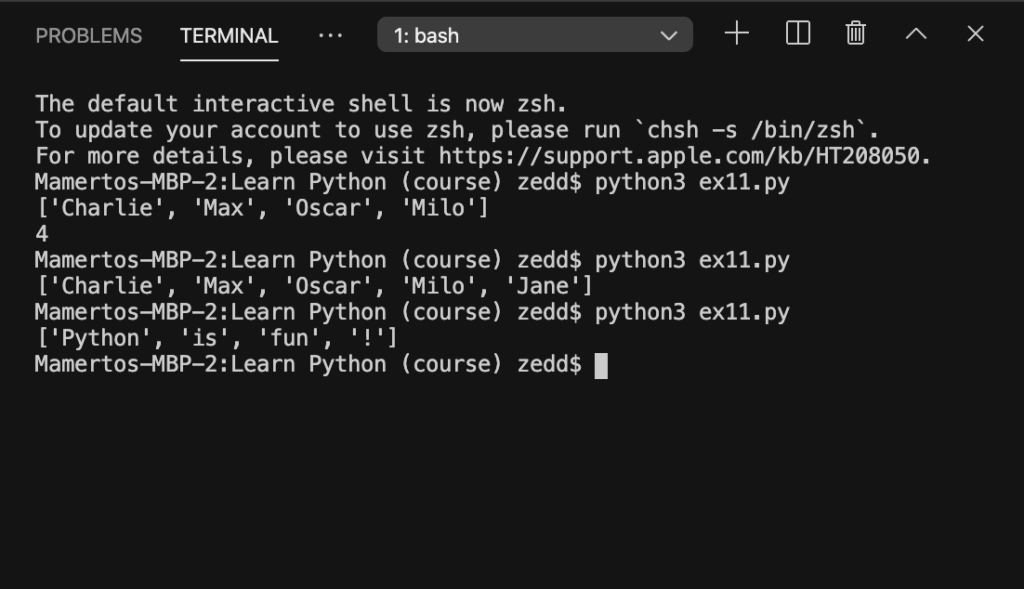
Alright! You might notice that we just keep on adding and including new elements into our list. But what if we wanted to delete an item from our list?
The del function
Just as it hints, it means, “delete”. This is a function so the way we implement it to our code is a little different with that of the previous methods. This is how we use it:
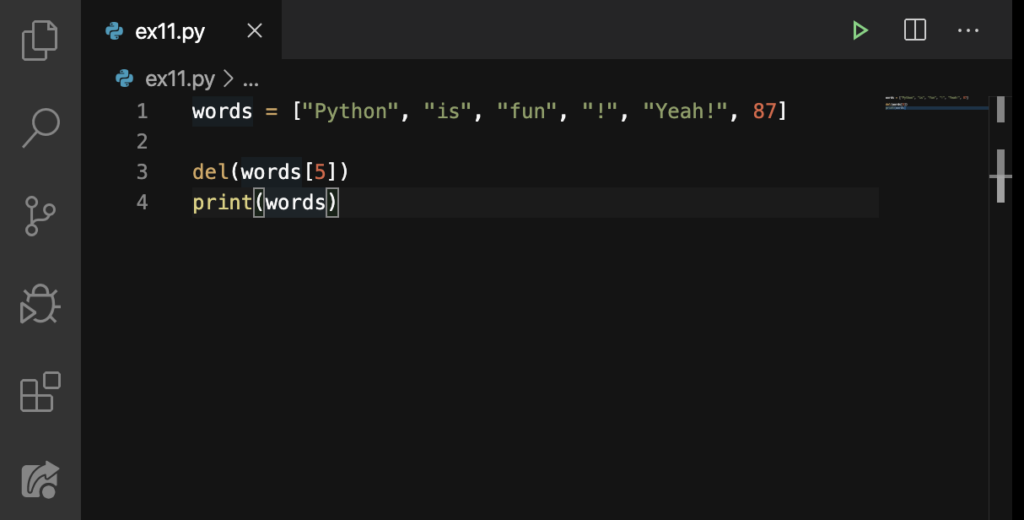
But if you would compare the way we used this function with the first list function we’ve learned (the len), it has something in common. Anyway, we just do this and then say the index of the element that we’d want to remove. In this case, we want to remove 87 from our list:
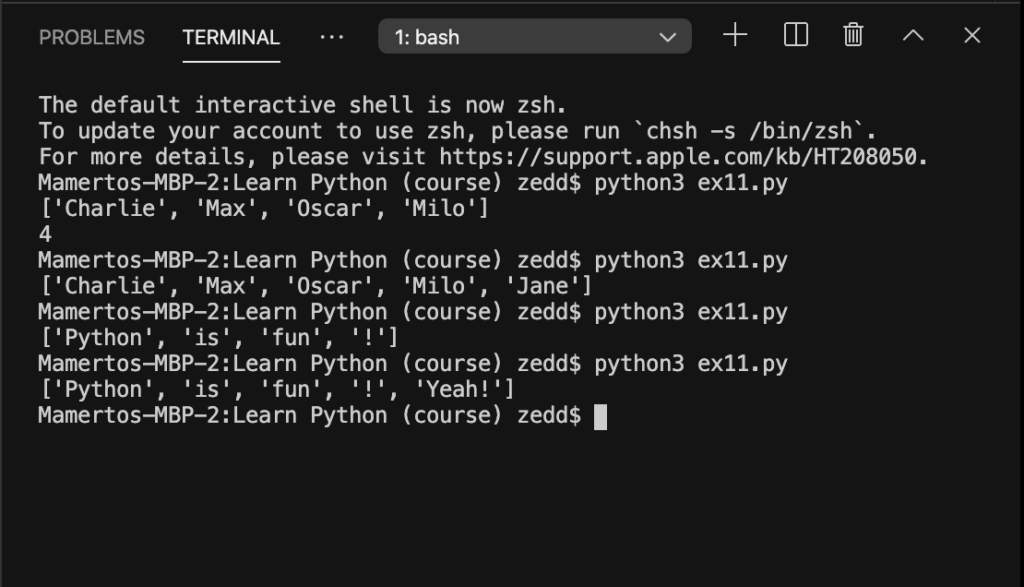
Good! What if we want to know the index of an element? Meaning, if we want to know what index an element has inside our list in order to do all these functions and methods that we’ve learned above (because we use the index to apply these), how are we gonna do it?
The .index method
Yup, we have this method. And this is how we use this:

We indicate the name of the list and then we implement the method. Afterwards, we enter the item or element we’re trying to find in a parentheses.
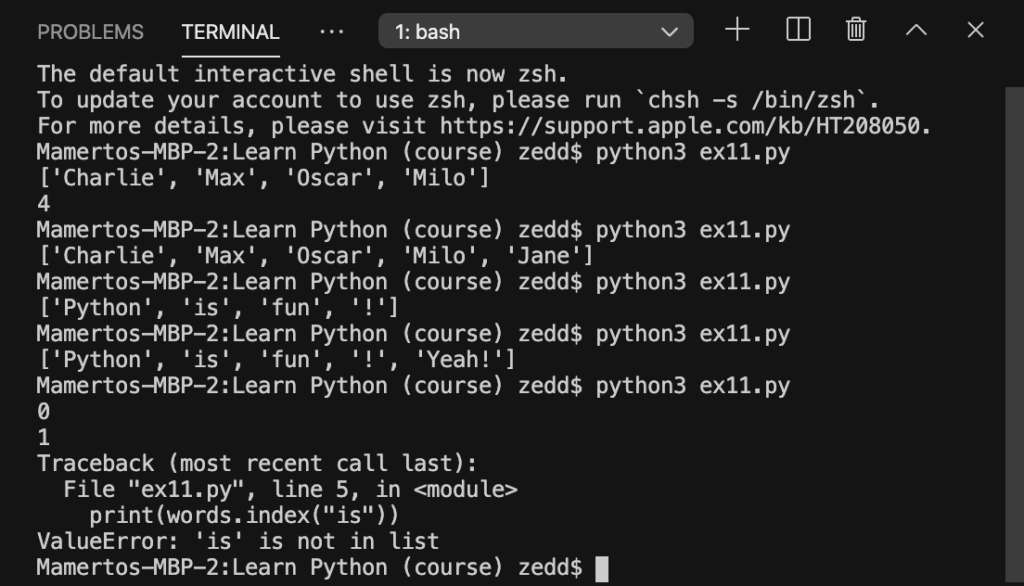
We can see the index of the first two elements and those indices are 0 and 1. However, if we’re trying to find an item that is not in our list, it will cause an error called ValueError. In addition, it will say the element that we’re trying to find is not in our list, saying: ‘is’ is not in list.
Bonus! The split() function
Type this in:
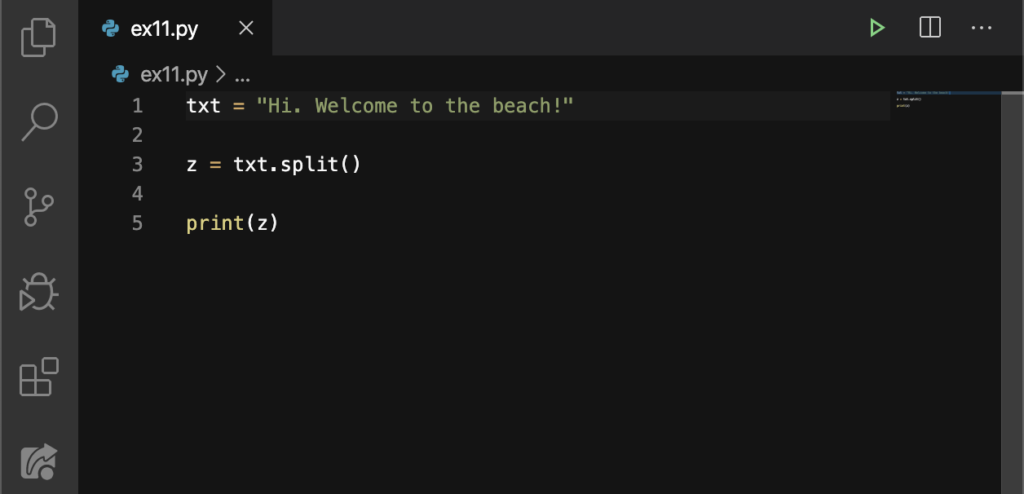
What do you think this split() does?
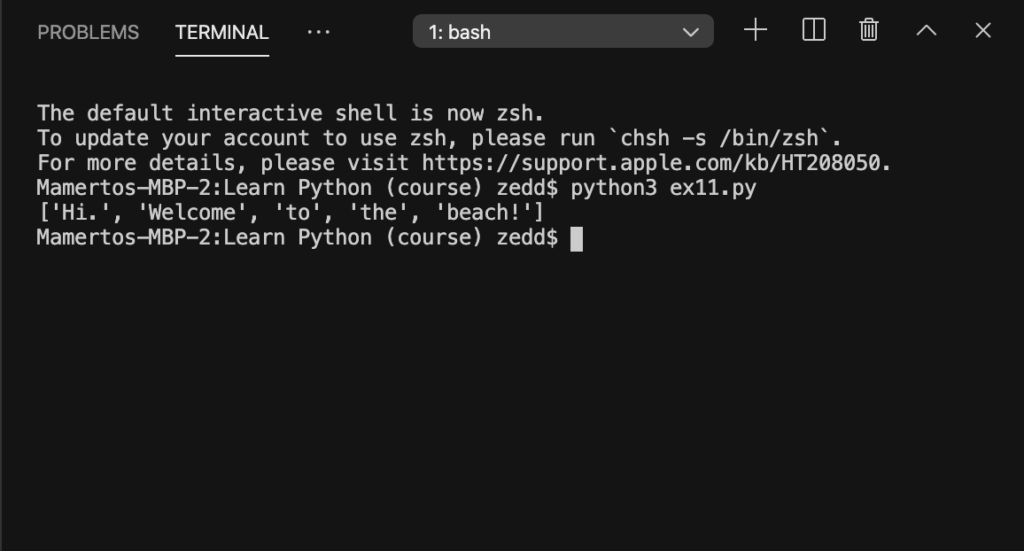
Excellent! Our text got splitted up and turned into a list. You can learn more about this method here.
That’s it! That’s all you need to know for now about the different methods and functions on lists in Python. And I know that we’ve tackled a lot that all of these might be a little difficult to absorb.
But, always remember that once you keep coding with all of these, you’ll get used to it. One more thing, if it has a dot, it is a method; if it doesn’t have a dot, it is a function.