Previous Lesson: Lesson 9: Templates in Django
In this lesson, we’ll improve our website’s homepage. We’re going to create a box where our user can put some text for the counting. And we’ll do that using HTML ‘forms’ for Django. Also, we want a button that will trigger the word-counting action.
Let’s start with our home.html:
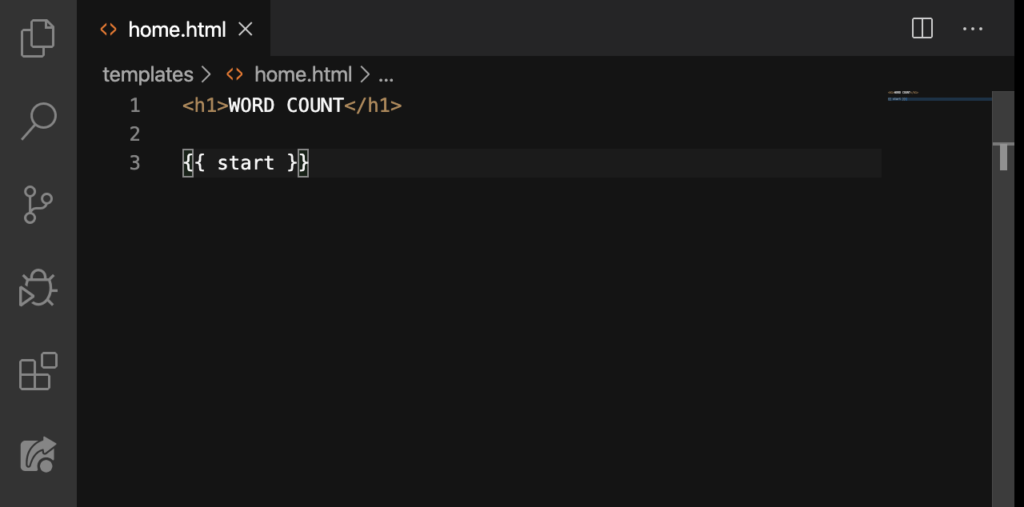
Let’s remove this:

DELETE:

Good. So if we removed that dictionary’s key here on this file, we’ll have to go to our views.py and delete this line, too:
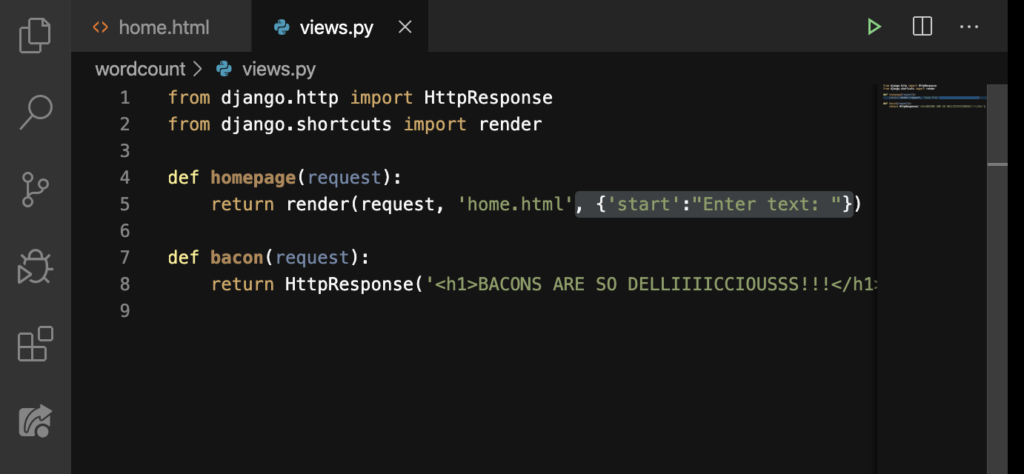
BACKSPACE:
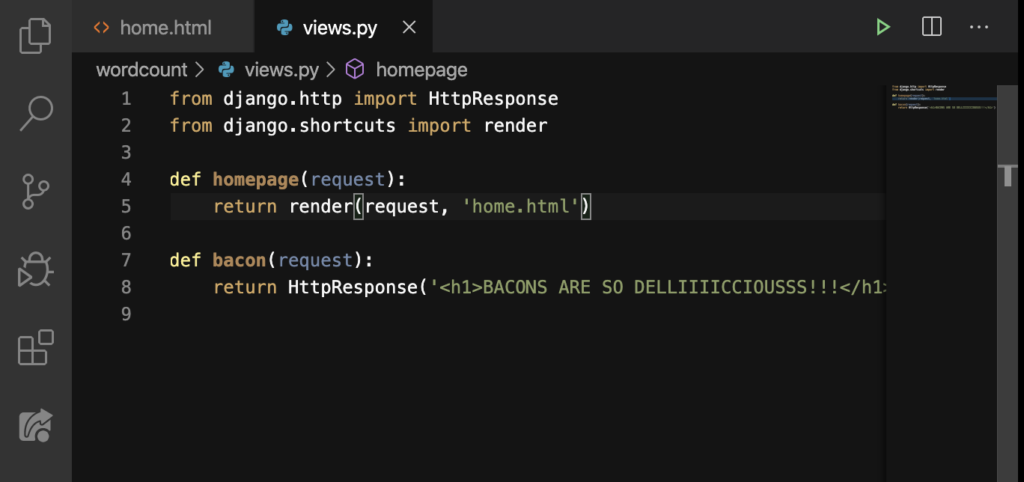
Good. Now how are we gonna let people enter in some text? In the HTML world, we use forms.
<form>
Forms is a place where people can enter some information. For example: first name, last name, email address; mostly like when you’re creating a new account fo yourself:
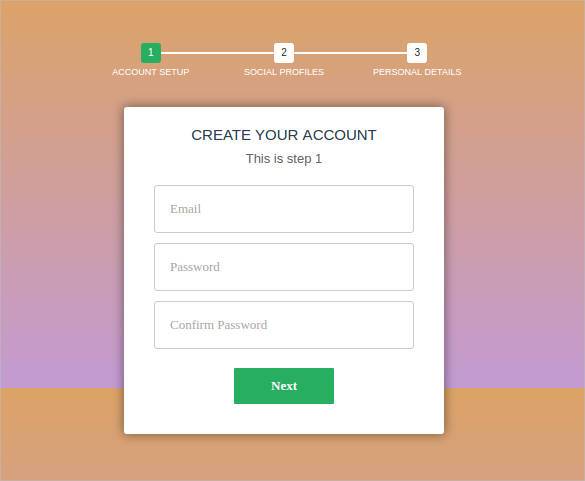
Of course, we’ll only have one box (or form) for the text. Let’s do it:
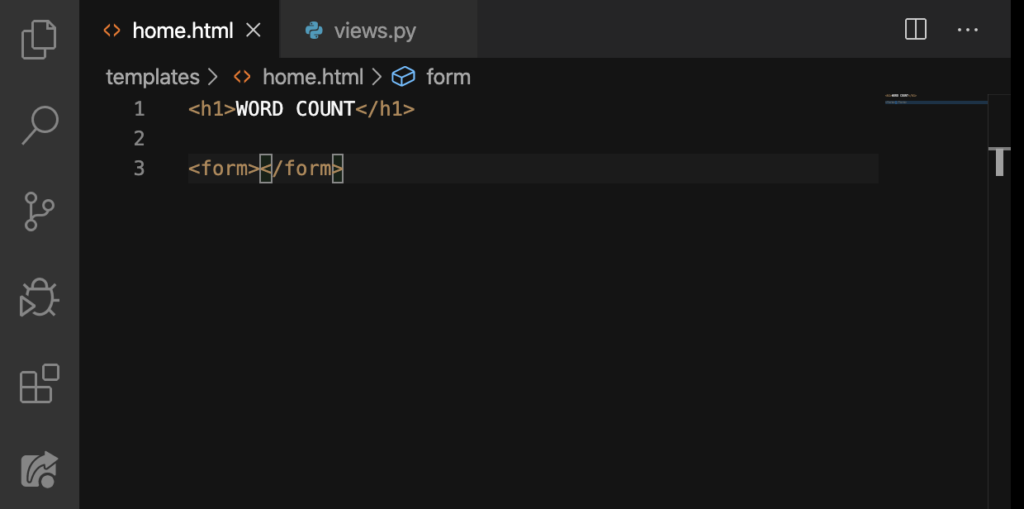
Inside the form tag, we’ll have action=””. But you’ll notice that as you type this, this shows up:
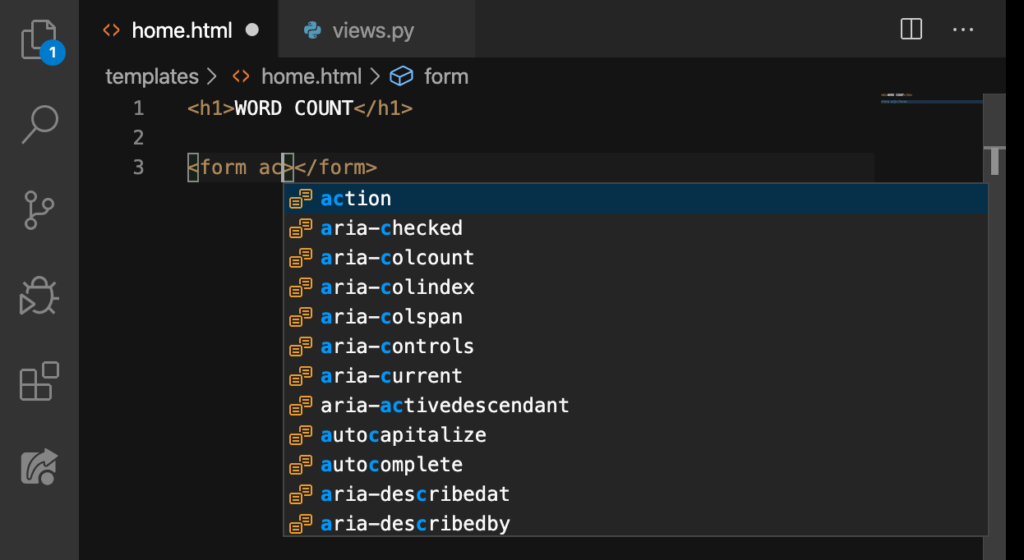
Once you’ve typed the ac (of action), press ENTER immediately:
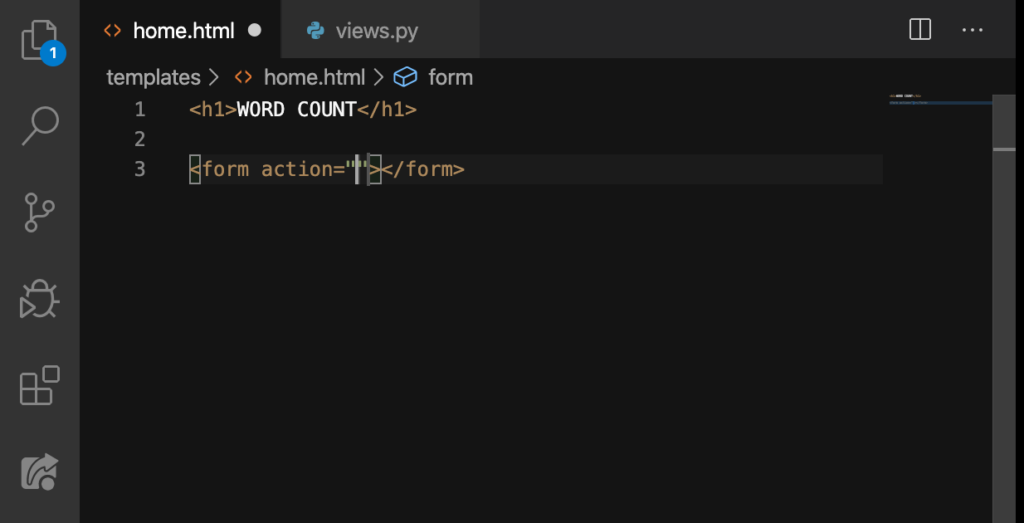
Between the form tags, press ENTER so that it will look like this:
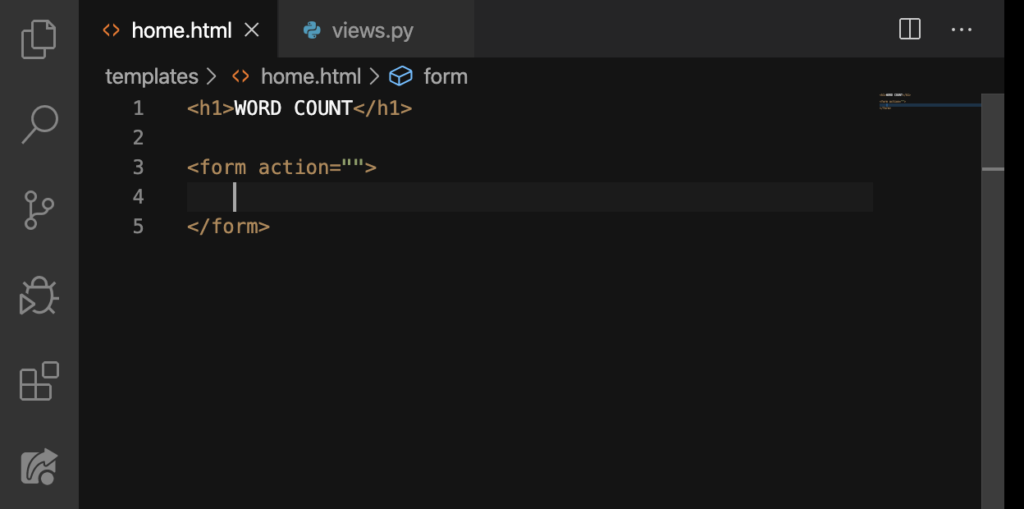
In those quotation marks, we’re gonna place the URL of our count page but for now, we’re gonna leave it blank; we’ll something first.
<textarea>
This is a big square box where someone can put a lot of information. You can also specify the size of this thing:
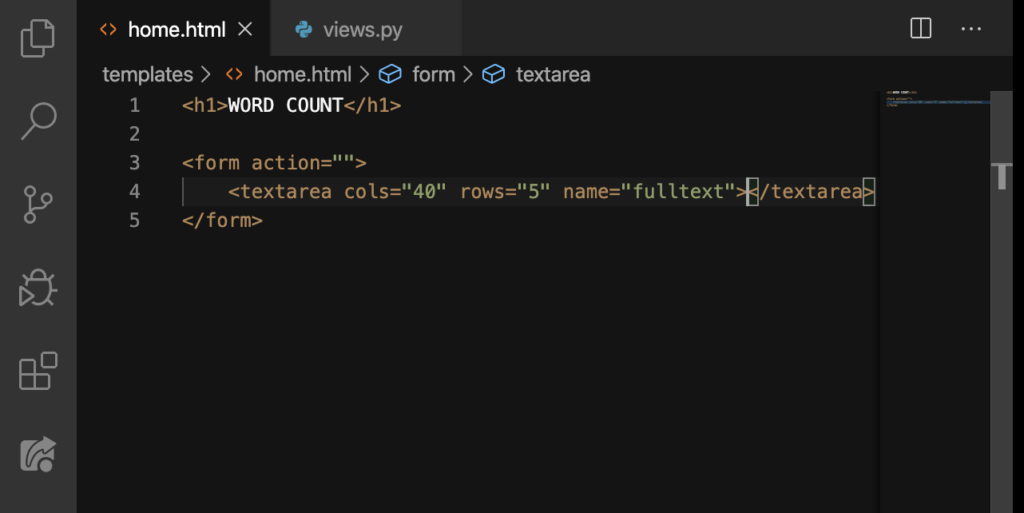
cols (columns) and rows specify the size of this thing. We also gave it a name. Finally, we’ll refresh our browser (make sure that your server is running):
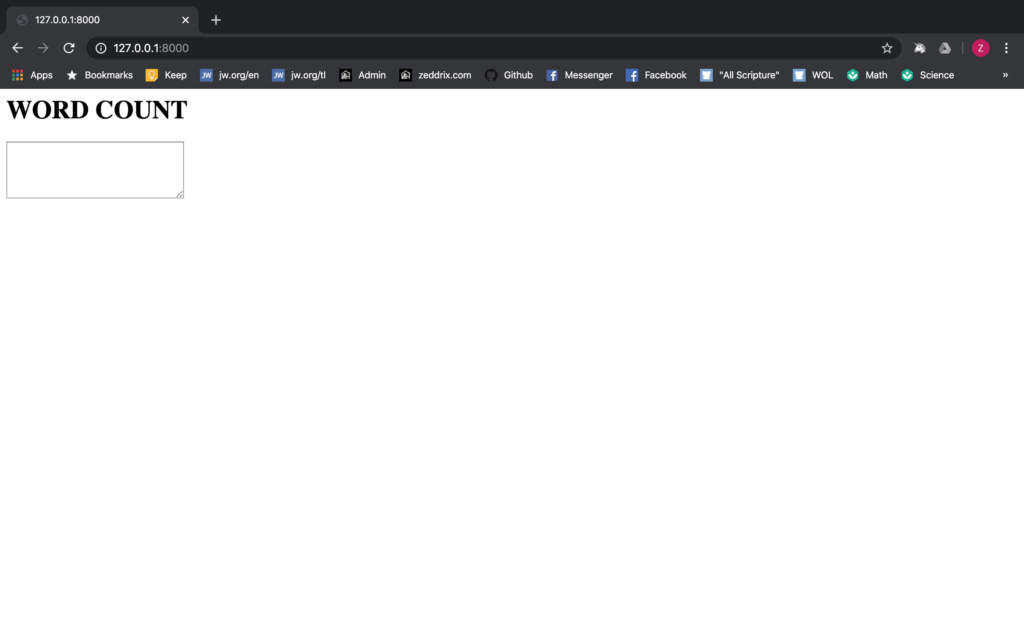
Yes! We now have this cute box here. I think it would be better if we make this box a little bigger (from 40 to 70; from 5 to 20):
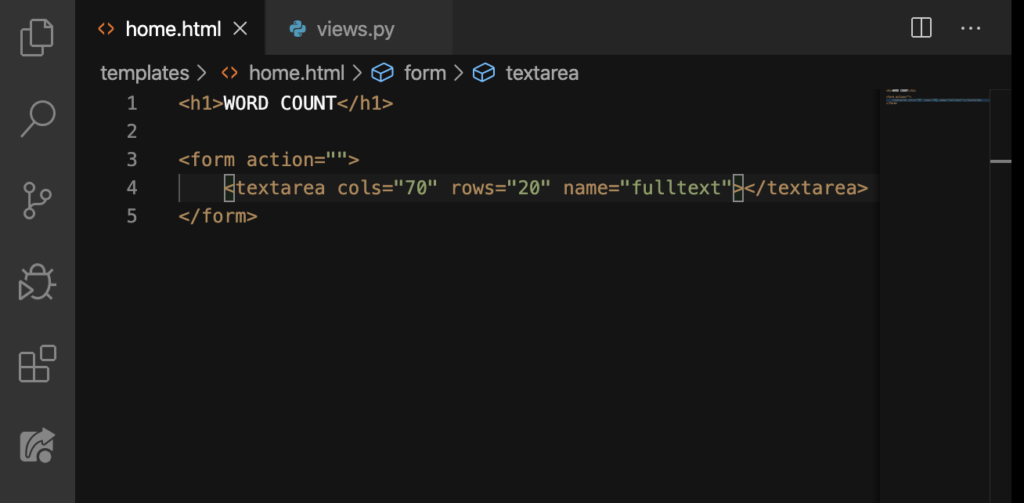
Save. Refresh:
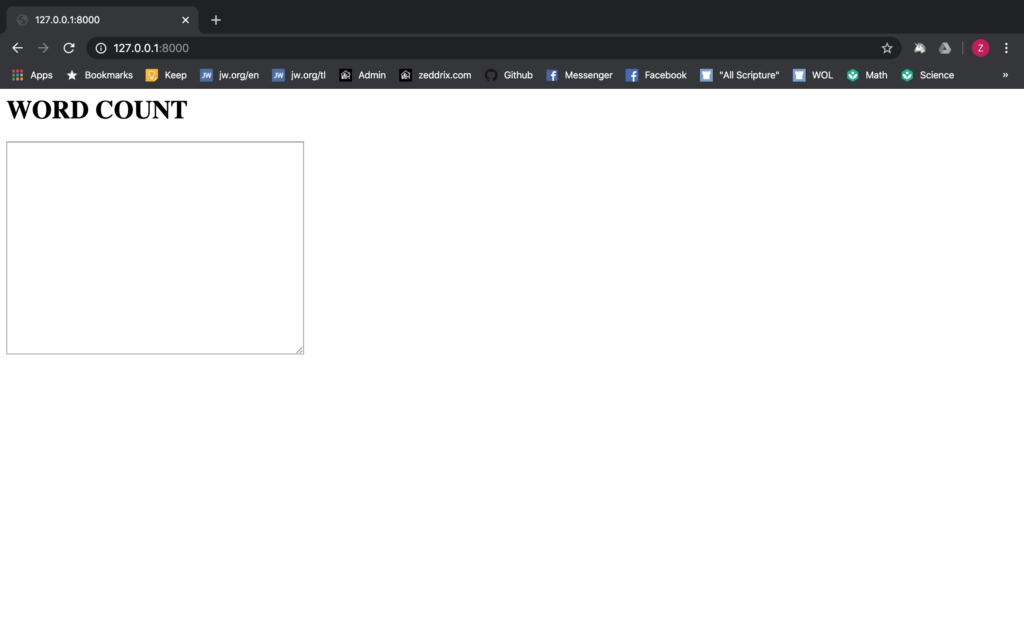
Good. Try entering something here:
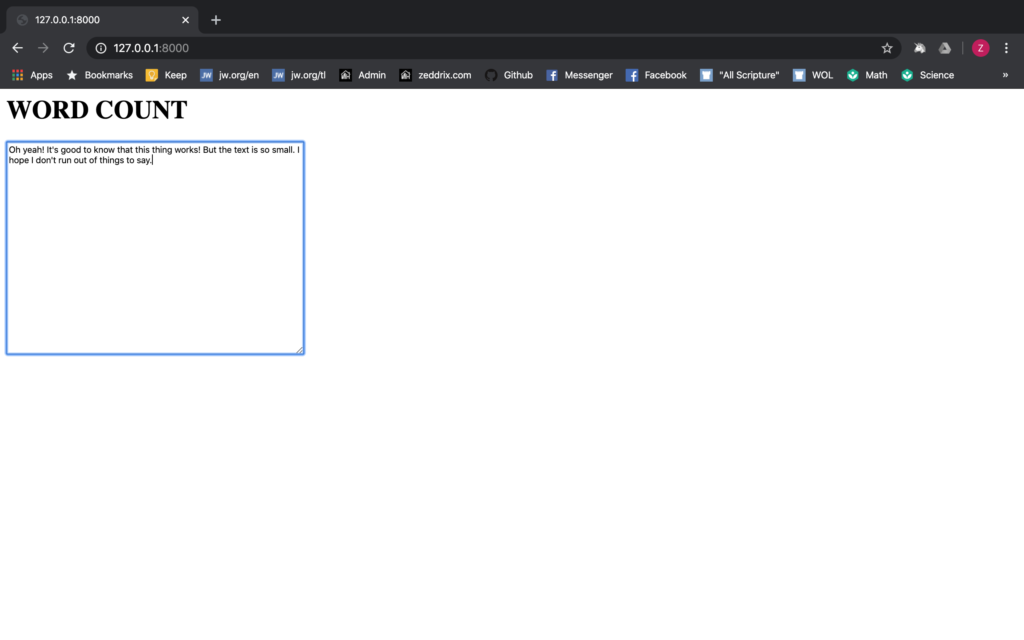
You done writing something here? I wouldn’t take much of my time thinking of what to say on this one because there is no button. And hitting ENTER on this form will be no good. So let’s put a button.
<input>
In order to have a button here, we’ll add an input tag like this:
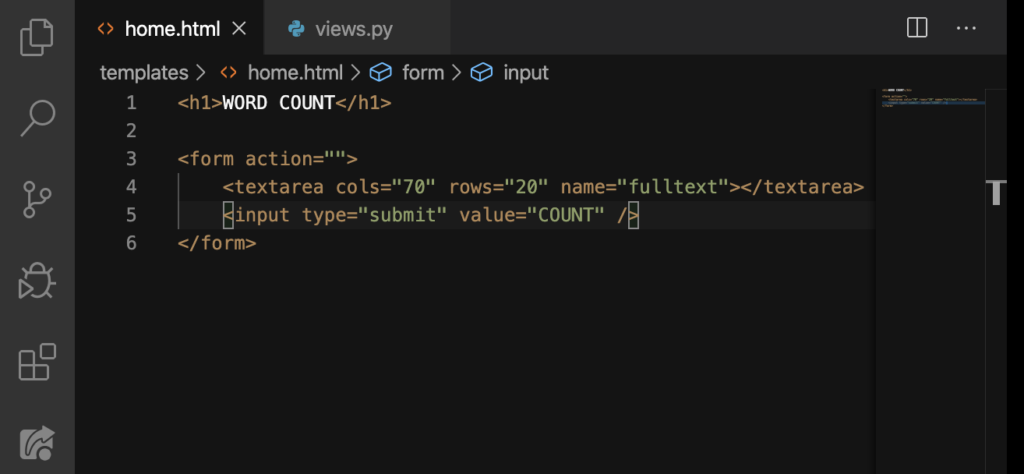
This input’s type is submit. As its type hints, once we’ve clicked on this button, it will submit our form. The value is the actual text that’s inside this button.
But, you may have notice that Visual Code didn’t automatically put in the closing tags when we tried to close this input tag. Why is that? That’s because we didn’t have to put anything inside two input tags. Let’s save this and go back to our browser:
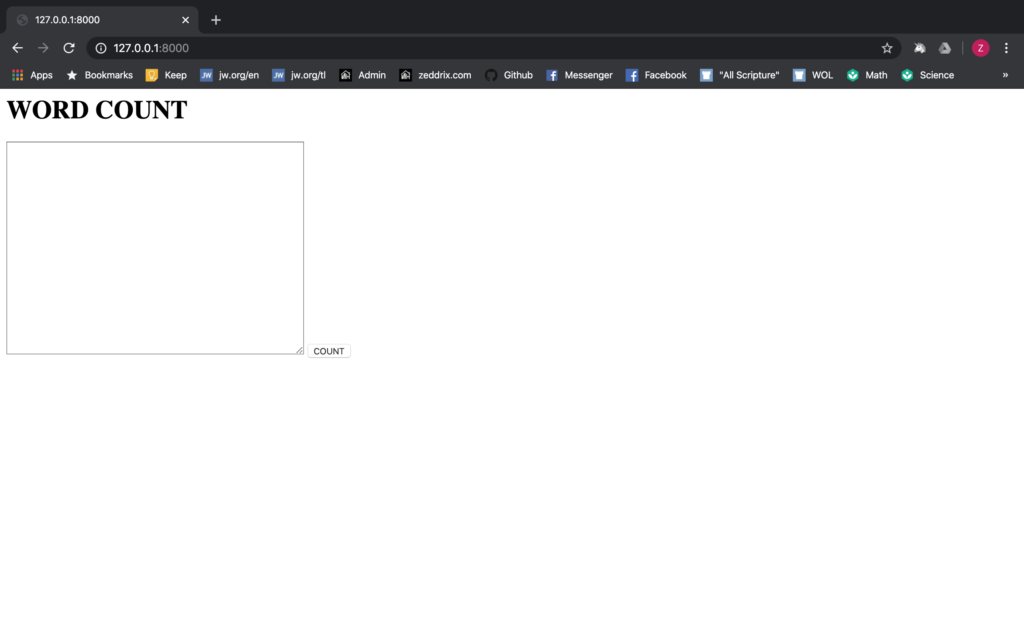
Nice. We now have a button! But I think it would be better if we would put the COUNT button under the form.
<br>
br means break. In Python terms, this is like using the /n (newline). We’ll just put this below our box (textarea) and above our button (input):
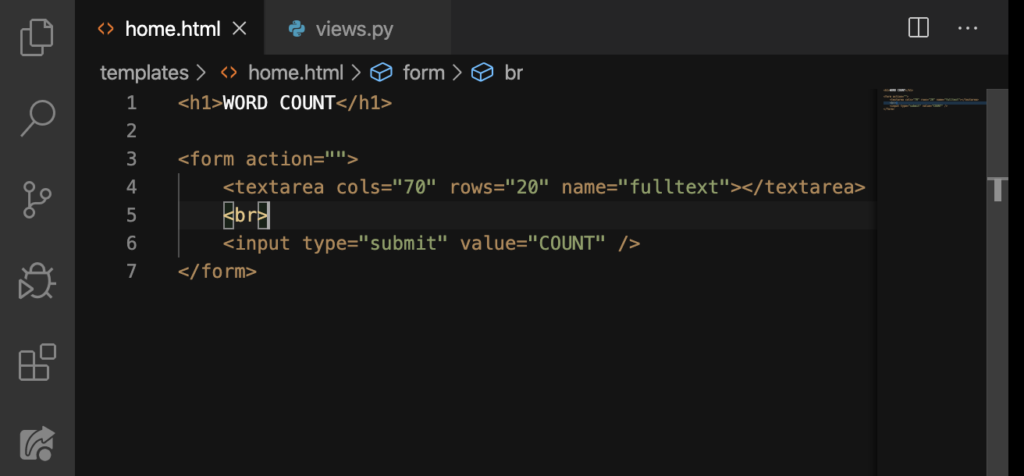
Reload our website:
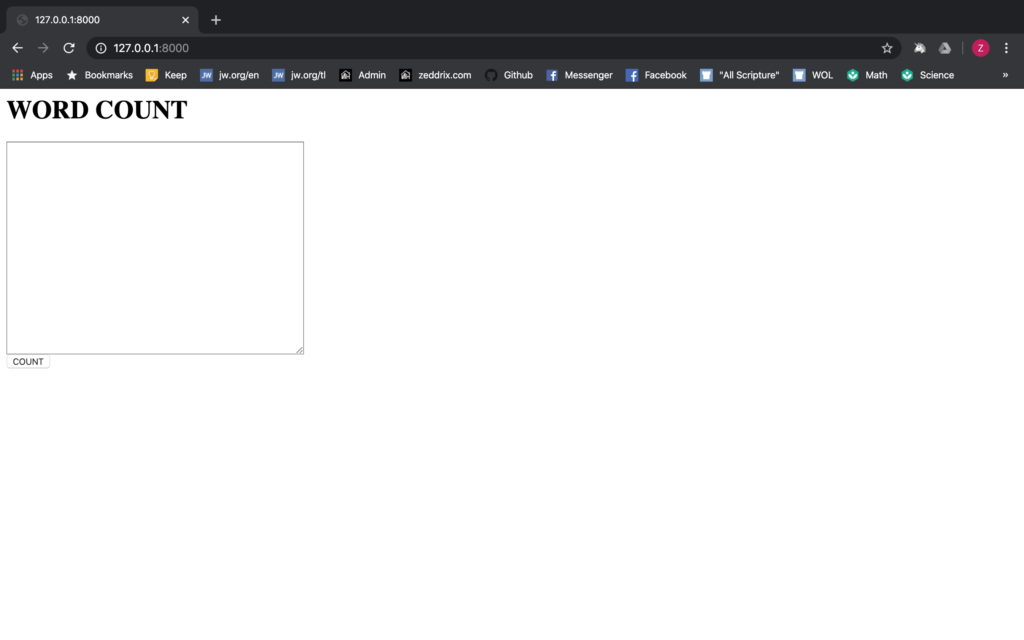
Now that’s better. If you want to have some more space, you can do more <br>. Therefore, you can be a lot more fancy with HTML. However, in our first site, we’ll not be taking care of the front-end fanciness.
Alternatively, we’ll be talking about Bootstrap on our second website. Moreover, it would make our website look professional without too much work. For the meantime, we’ll keep things simple because I want to teach you the basics in Django.
But I would really want to make everything here bigger. We can’t keep on readjusting the box because our COUNT button will still be smaller. So I’d say we just zoom-in:
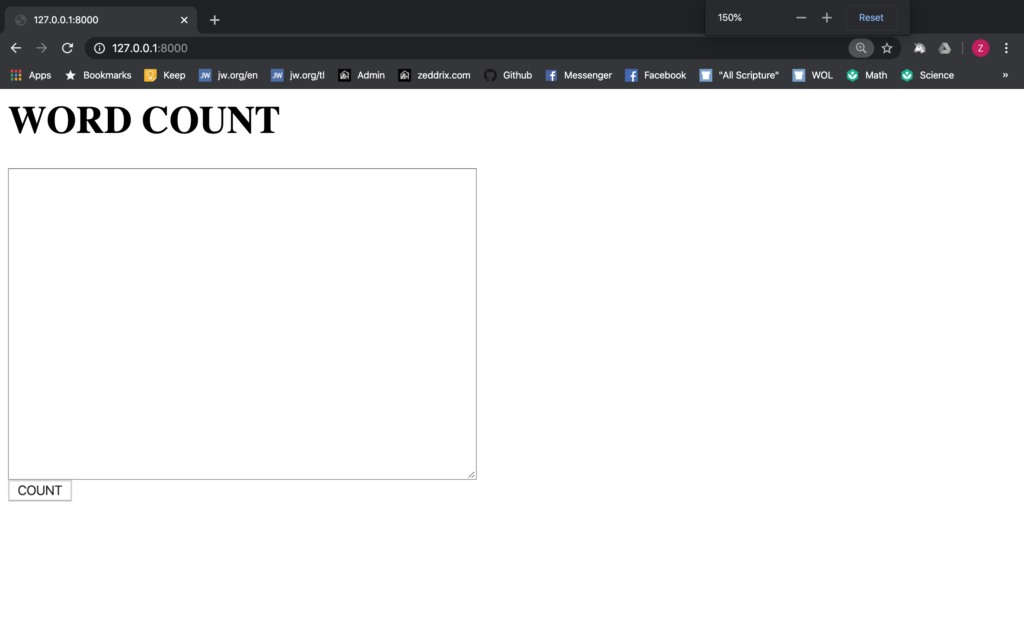
That’s better. Now let’s test this button out! First, enter in some text:
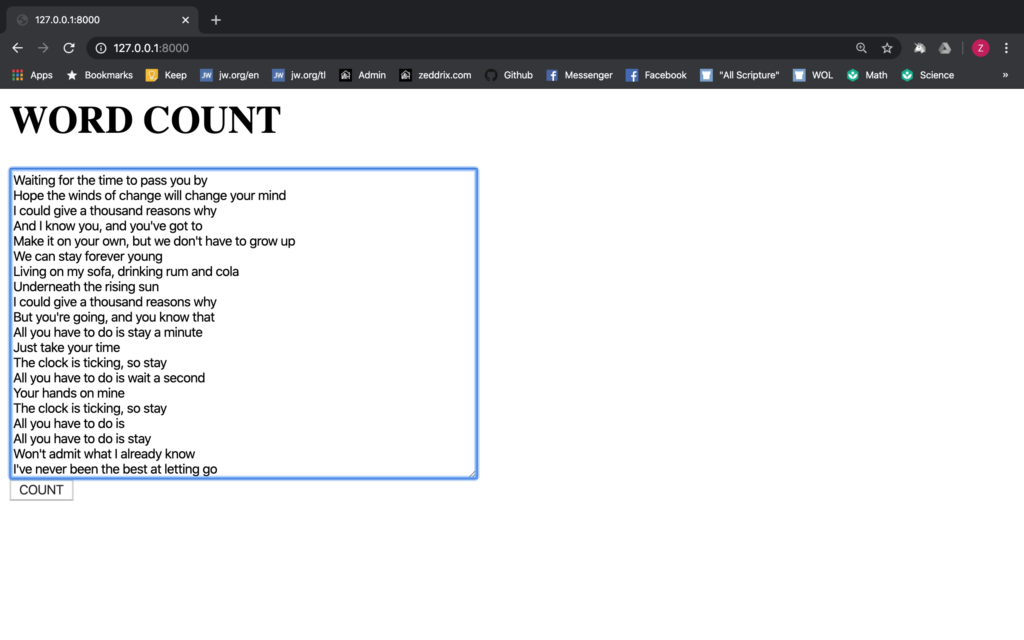
Then, click on the COUNT button:

“What?” Yup. It does nothing because we had to direct this to another page where the word counts are all displayed.
Count Page
Let’s first clear some things here on views.py:
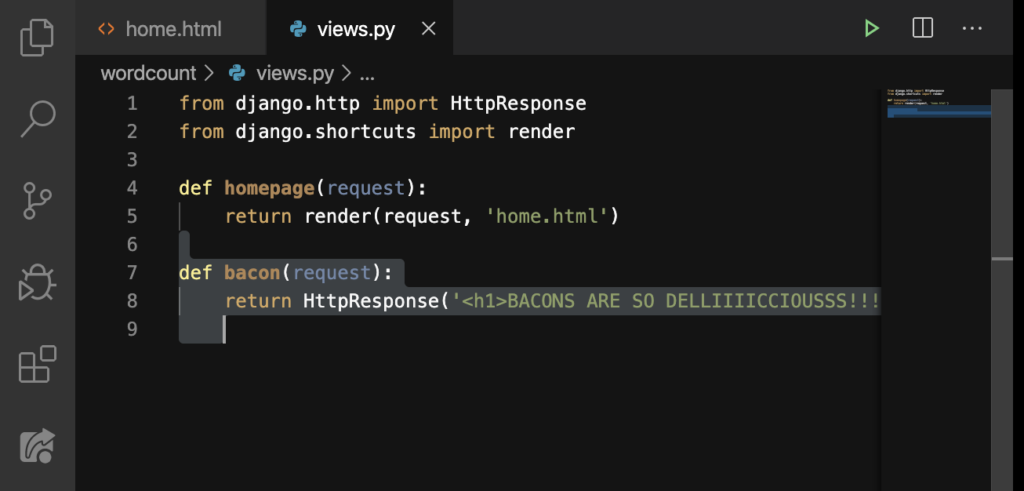
DELETE:

Good. Let’s also remove this one here on our urls.py file:
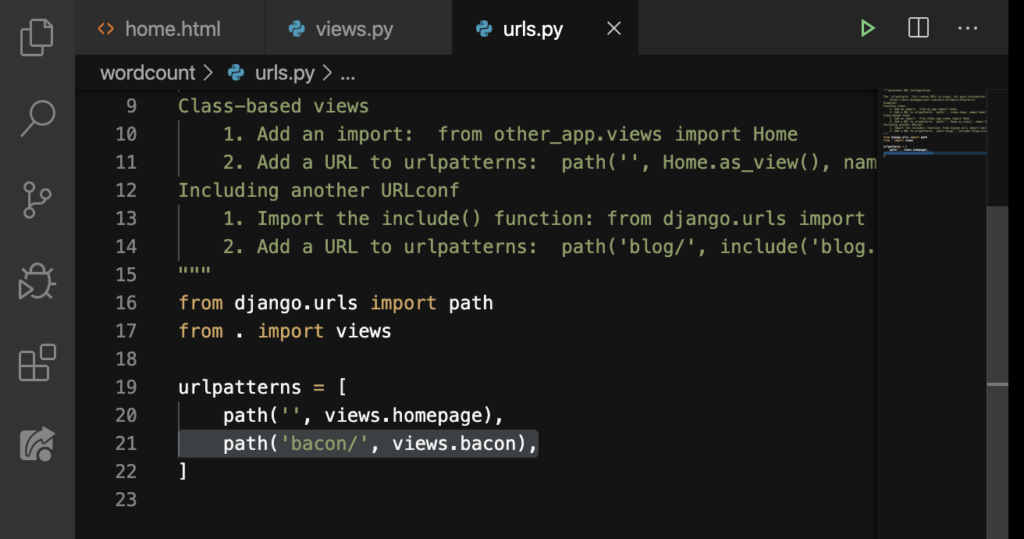
DELETE:
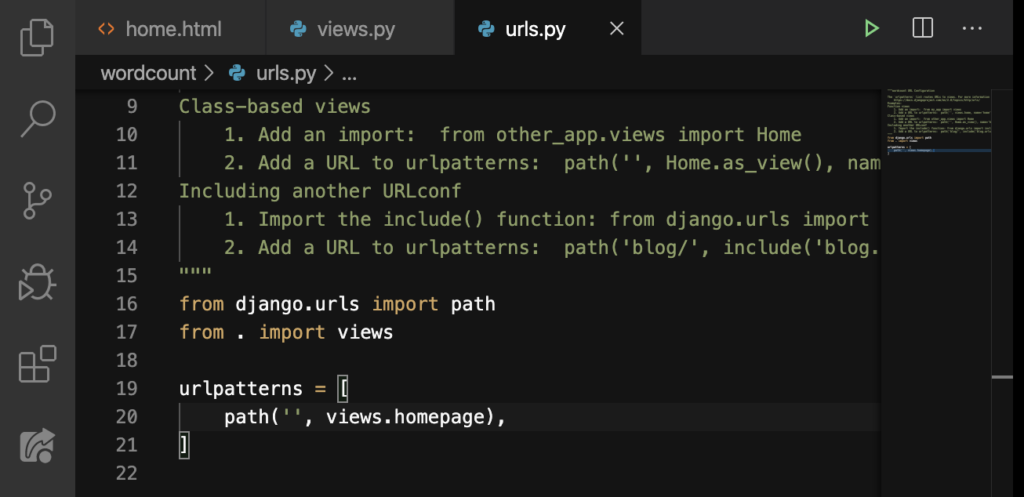
Perfect.
New path
Now let’s create a new path on Line 21:
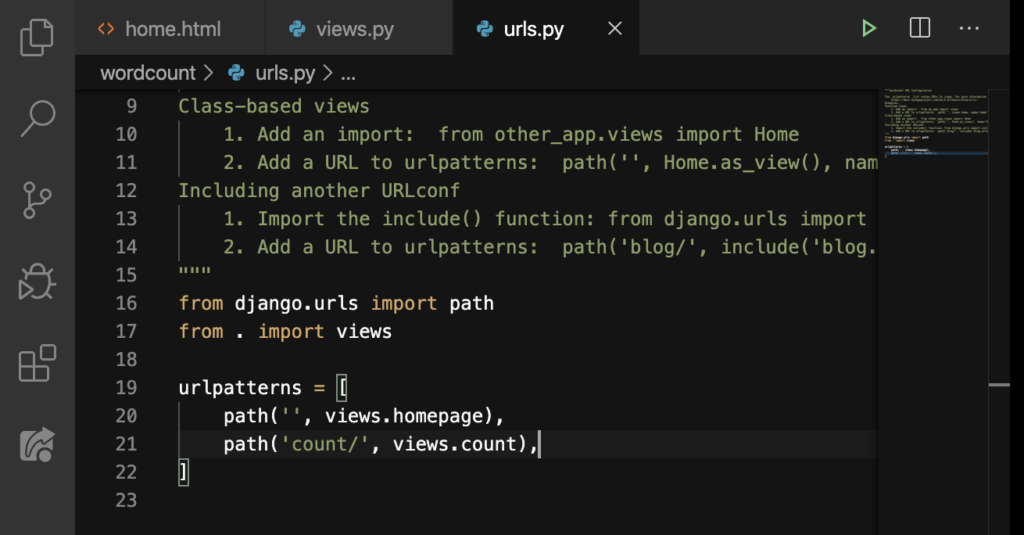
We want take them to our count function inside our views.py file. We don’t have that count function yet so we’ll make one.
def count()
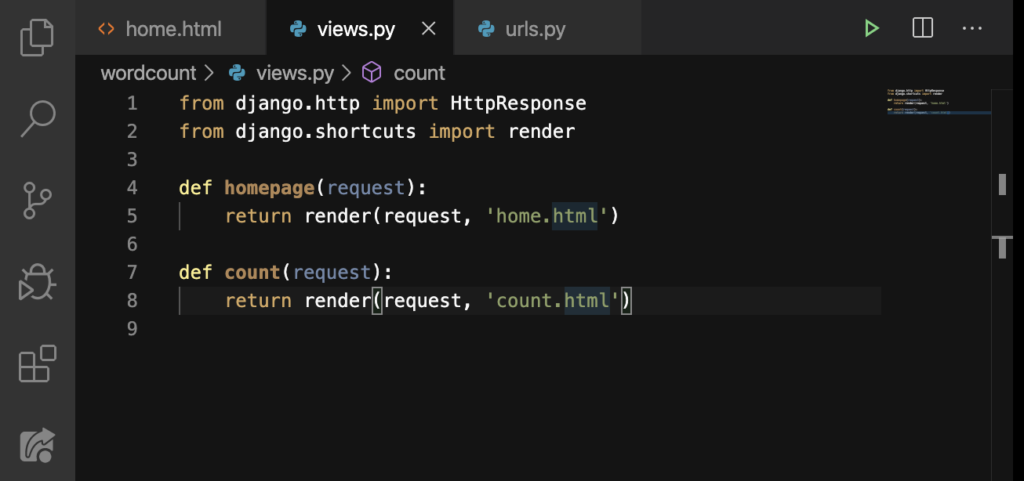
So, this will send them to the count.html file. We don’t have a count.html file yet so we’ll make one.
count.html
Let’s make one inside our templates folder:
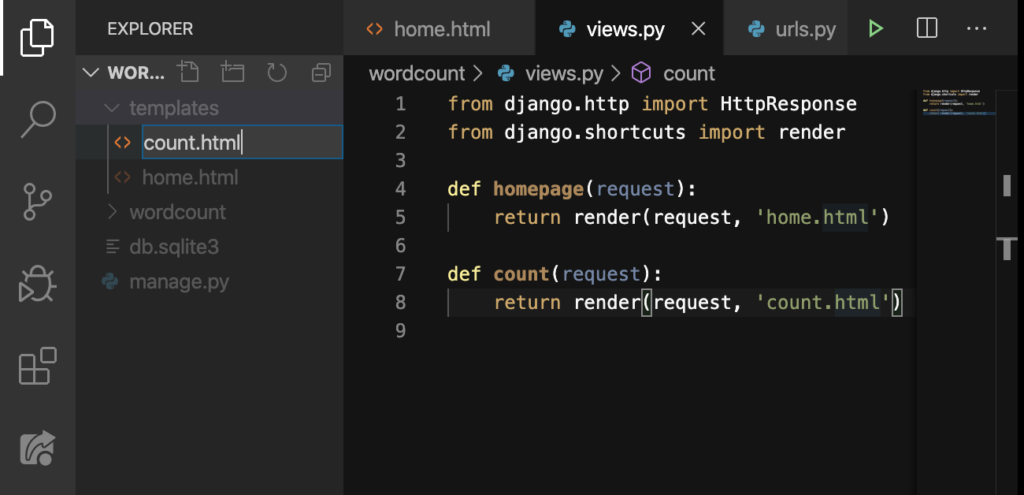
ENTER:

Just to make sure that everything works fine, let’s type this:
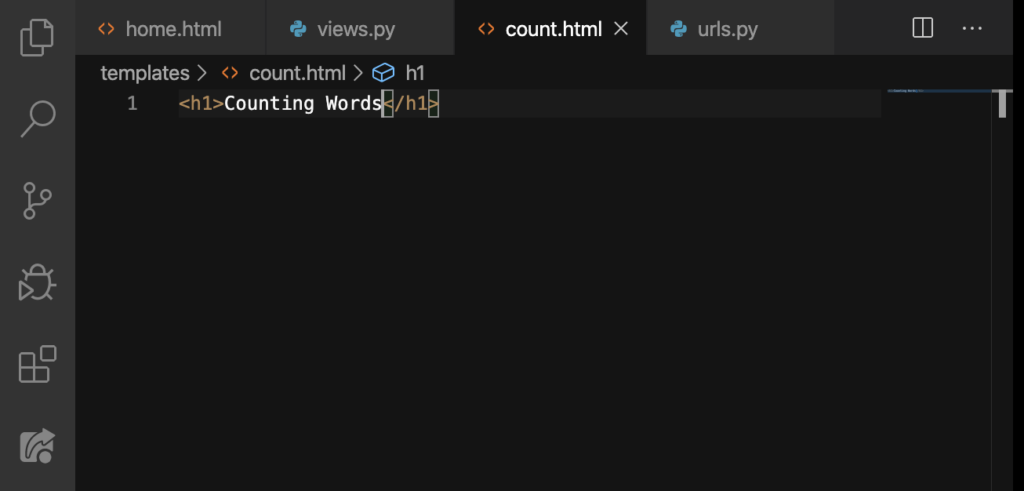
So, when we hit that COUNT button, this should take us to another page with the big Counting Words. Let’s try entering some text again:
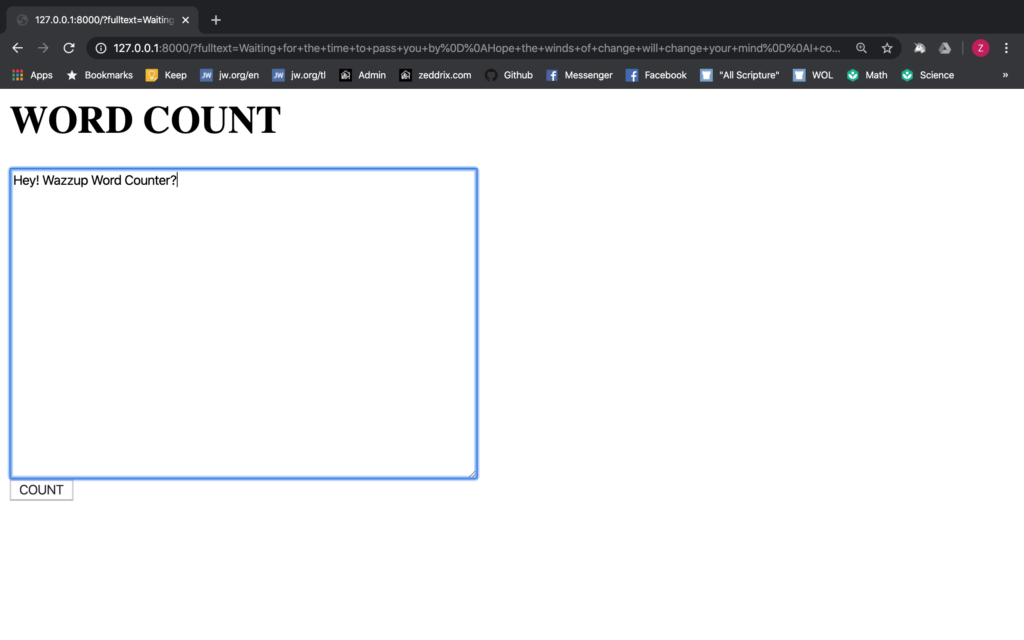
Hit the COUNT button:
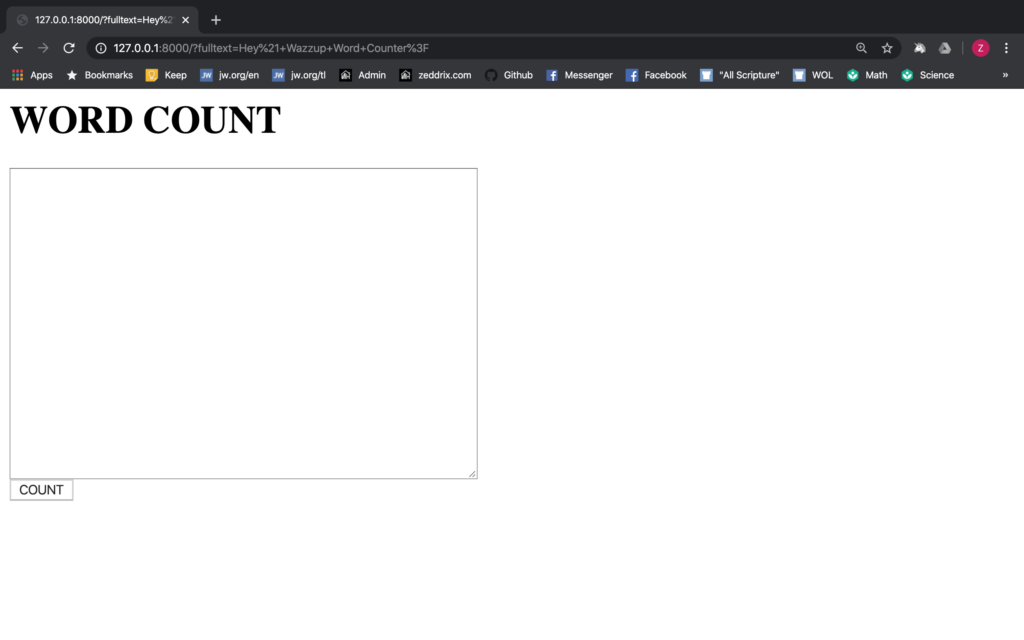
Okay nothing happens. Why is that? It’s because in our home.html, we’ve left our action blank:
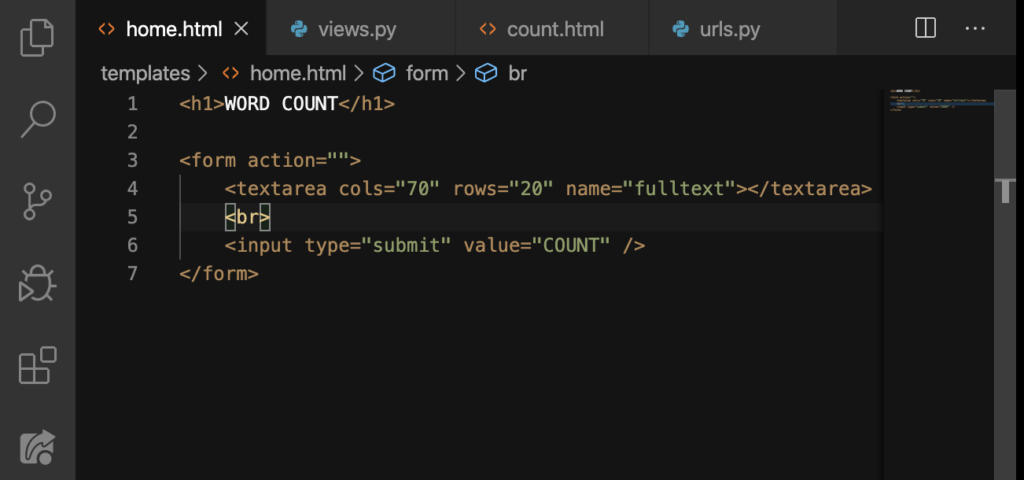
action=””
Let’s put count (name of our page) in between those quotation marks:
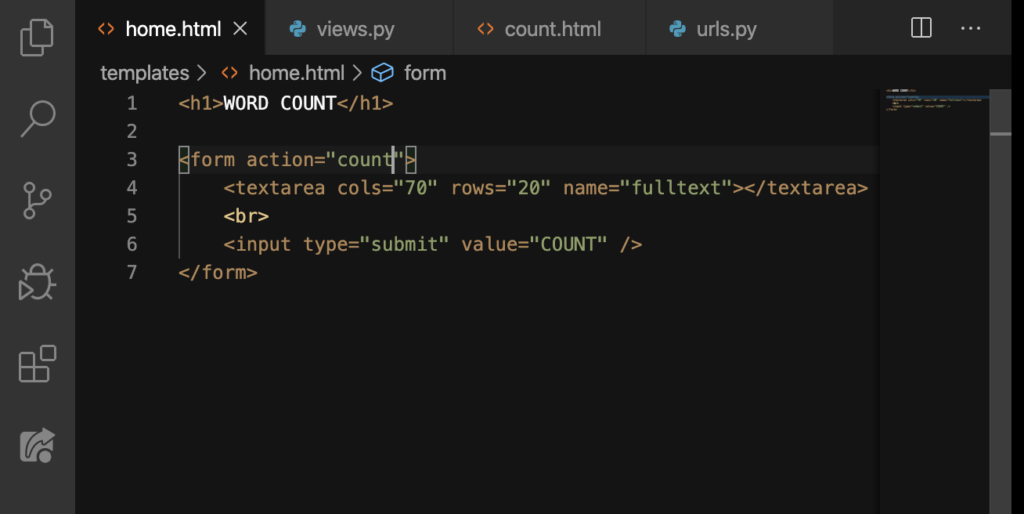
Done. Now let’s refresh our browser and enter in some text:
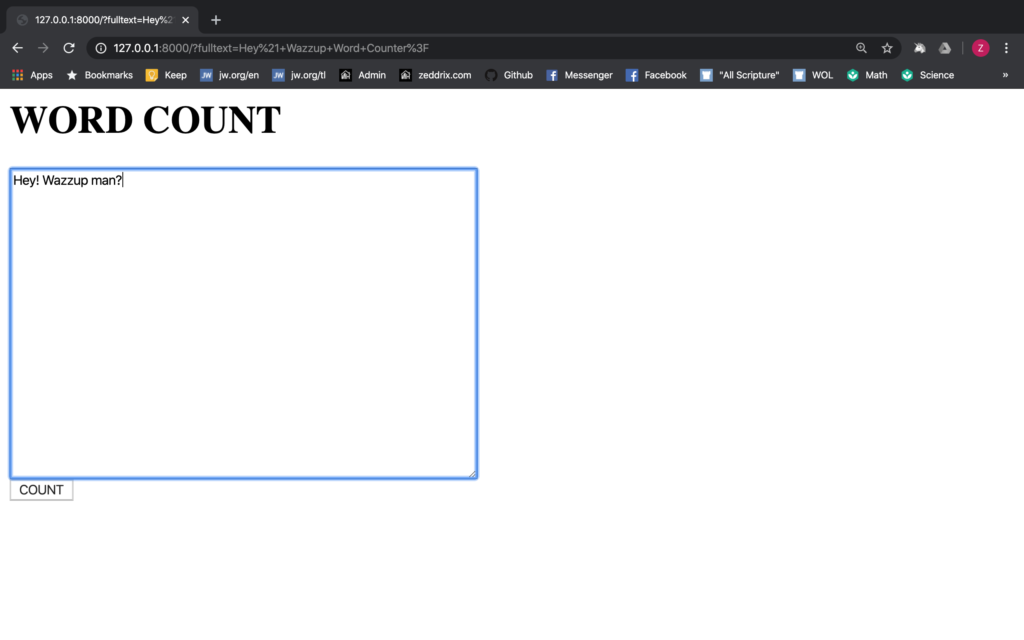
Hit the COUNT button:
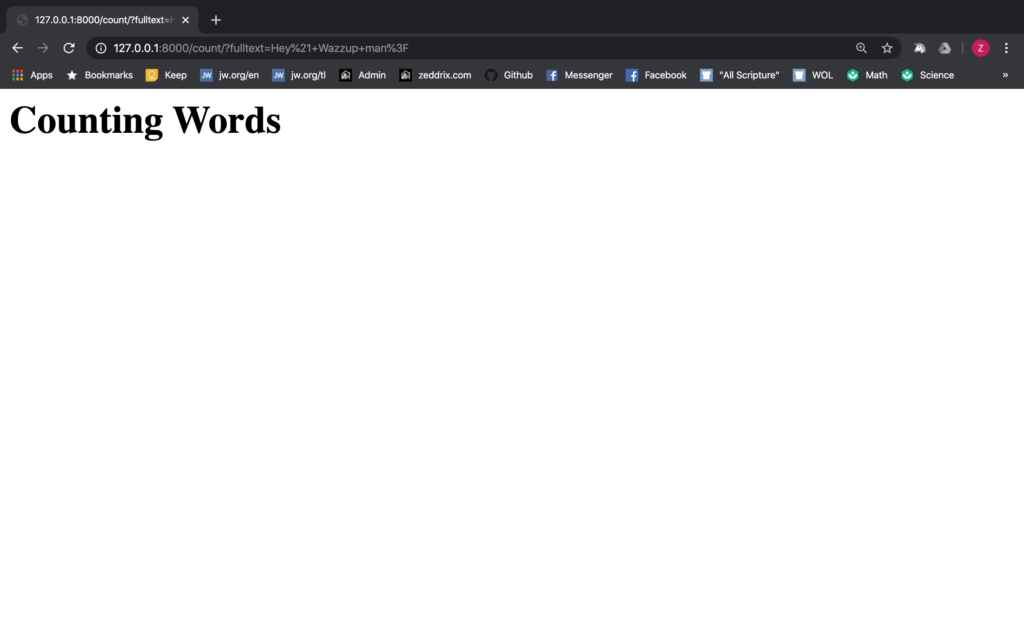
YES!!! It finally works! Forgive me if I was a little overreacting. But programmers always have that joyful moment once they’ve solved a problem with their code. That’s what I’m feeling right now. You may not be feeling that too but once you start developing some websites or games on your own, you’ll have that feeling.
Anyway, there is one issue here with regards to typographical error, or sometimes, we really need to make some changes. That’s why we’ll need a pliable URL.
A Pliable URL
What do I mean by “having a typographical error” and have some changes with our code? For example, we have to change the URL path count/ to counts/, or we’ve mistakenly added an s there after the counts:
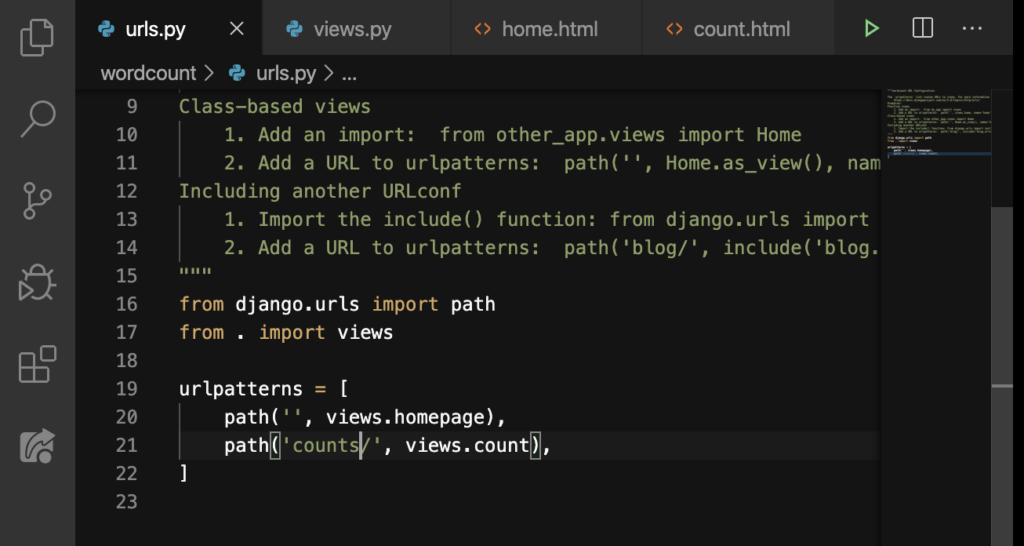
This would be a big problem because look after refreshing our browser:
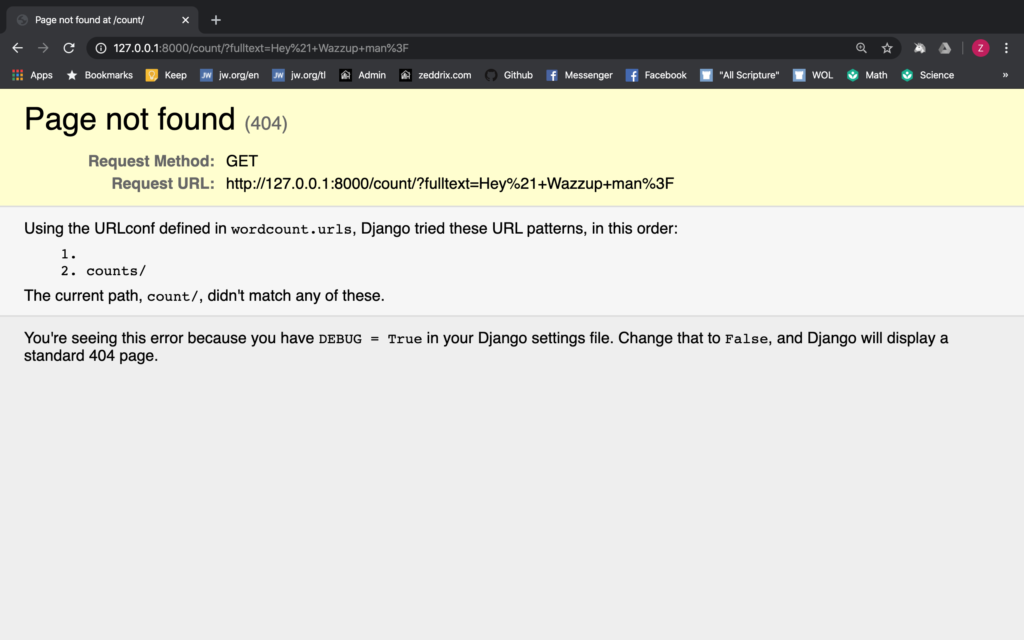
Yes, Django can’t find that path. Fortunately, we have something that handles this. But first, rename it back to count/:

Good. Now go to home.html and do what I did on Line 3:

The % allows us to enter in some URL. In this case, we entered ‘count’. Lastly, go back to our urls.py file and do what I did on Line 21:
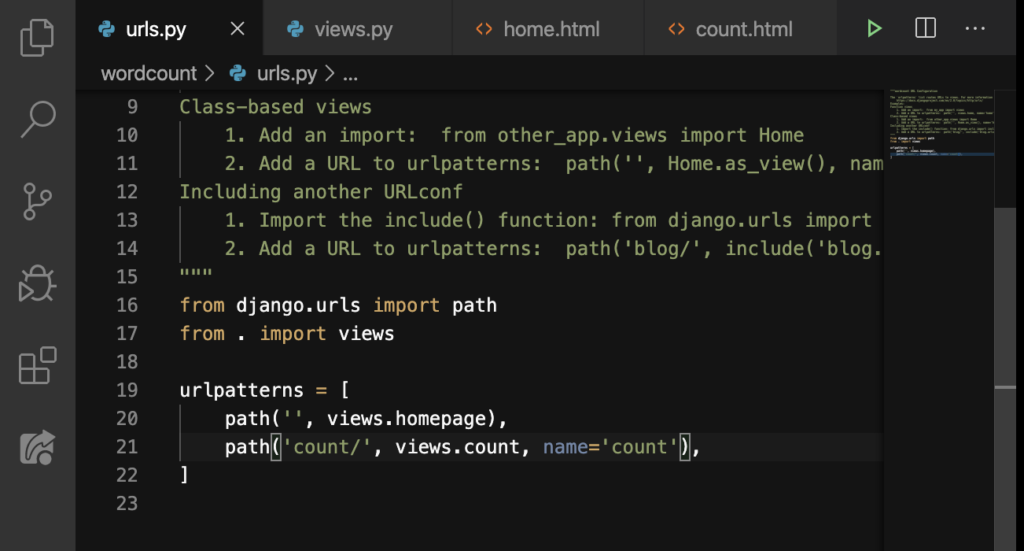
Note, however, that whatever we’ve assigned for the name=”” here, the URL that we used on the home.html should be exactly the same:
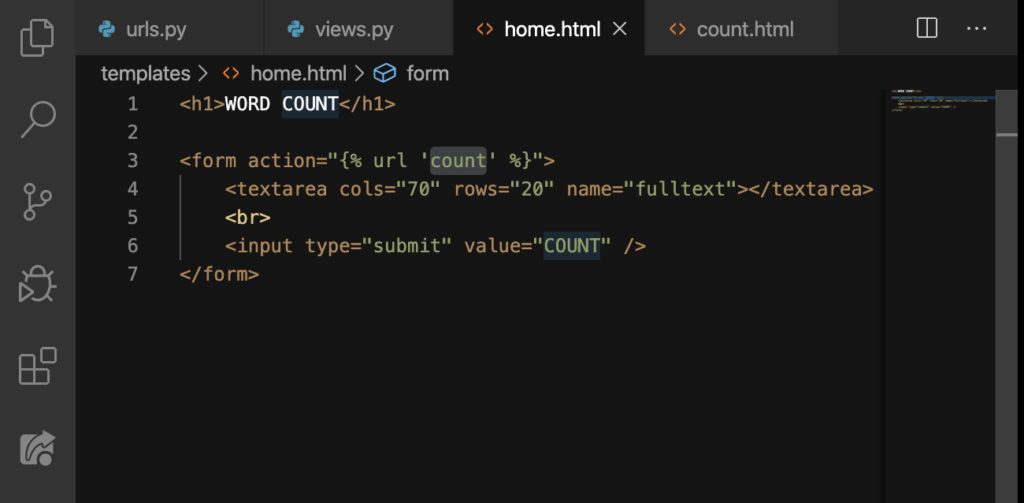
In short, if we use count on the name=””, we should use count on the URL. That’s all you need to consider and after that, you can change the path’s name with whatever you want. For instance, let’s change count/ to countthewords/:
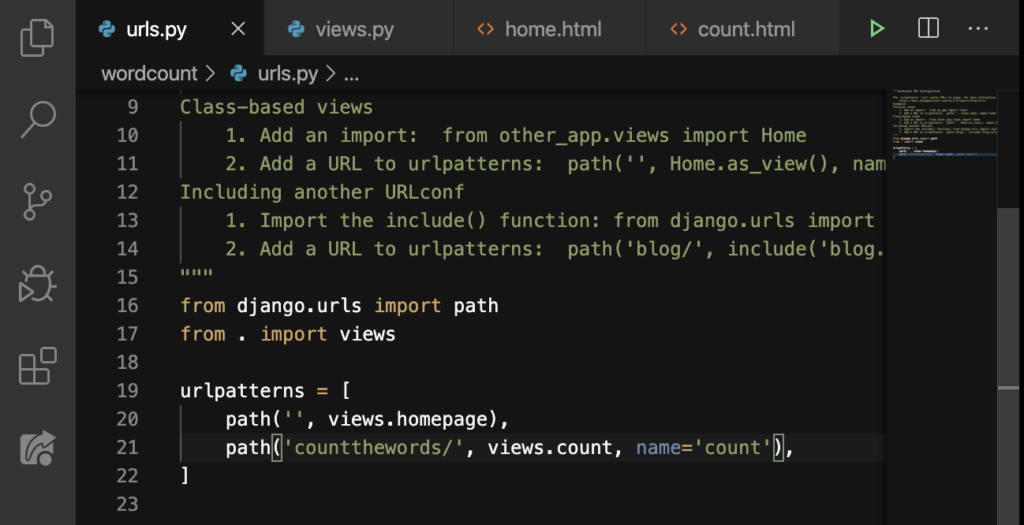
Let’s go back to our homepage, refresh, and then enter in some text:
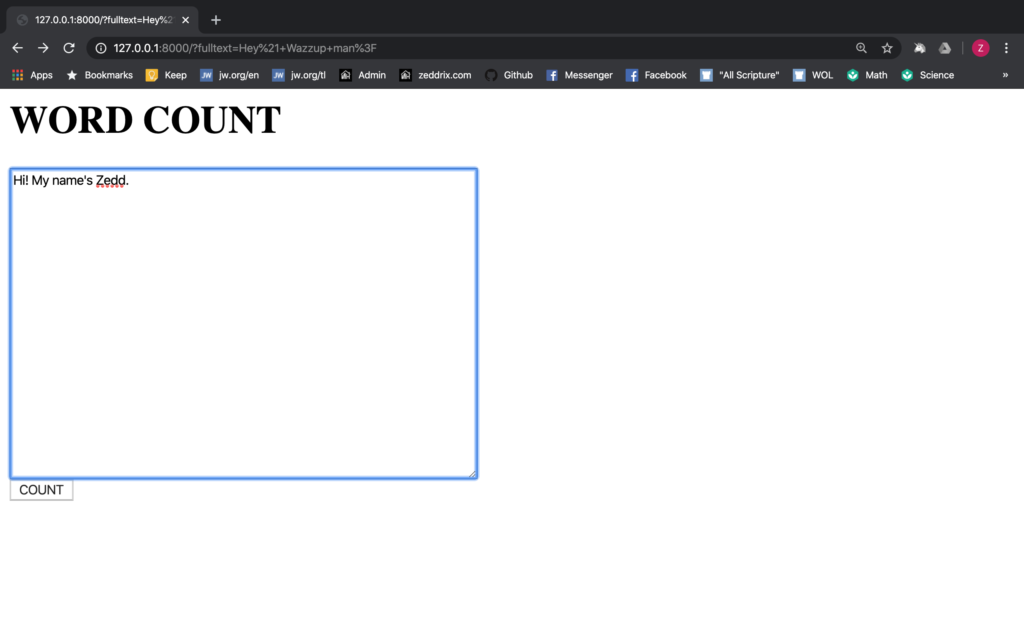
COUNT:

Excellent! The page not found didn’t show because we have a pliable URL. But, I think /countthewords is a bad name so I guess I’ll just stick with count/:
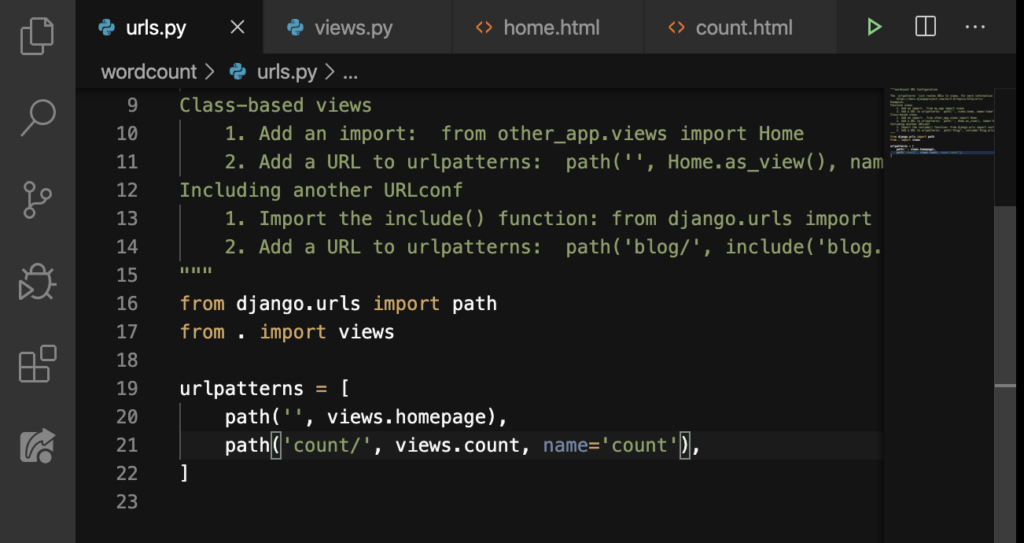
That’s it for HTML forms for Django. Tell me, did you learn a lot? If that’s the case, good, because you’ll have a lot more to learn on the next lesson. Besides, we still have to display the word count of the user’s text.