Previous Lesson: Lesson 12: Let’s Count the User’s Text Using Django!
In the previous lesson, we were able to count the words in our text. But in this lesson, we’ll get the keyword density of our text using Django. And I also showed you an awesome website that’s called: wordcounter.net.
By the way, if you hadn’t seen that website, you should check it out because that website would be pretty much like the website we’re building here. Let’s start! So, here’s the plan:
Our Plan:
- We’ll convert the entered text into a list.
- Every word that’s in that list will be the keys for our dictionary.
- We’re gonna define a function that will count the occurrence of a certain word.
- If this function confirms that a word hasn’t occurred yet (e.g. ‘bacon’), that word will be a new key inside our dictionary, having a value of 1. (so ‘bacon’ will have 1 as its occurrence or keyword density.)
- If another word (another ‘bacon’) appears in the text, our function will add 1 to the occurrence of that word.
- Hence, that word will have 2 as its density or occurrence. (so ‘bacon’ will now have 2.)
- This cycle gets implemented on all the other words in the text. Finally, every keyword’s density gets displayed.
- Let’s do it on our code and get the keyword density using Django!
Execute Our Plan!
Let’s go to our views.py:
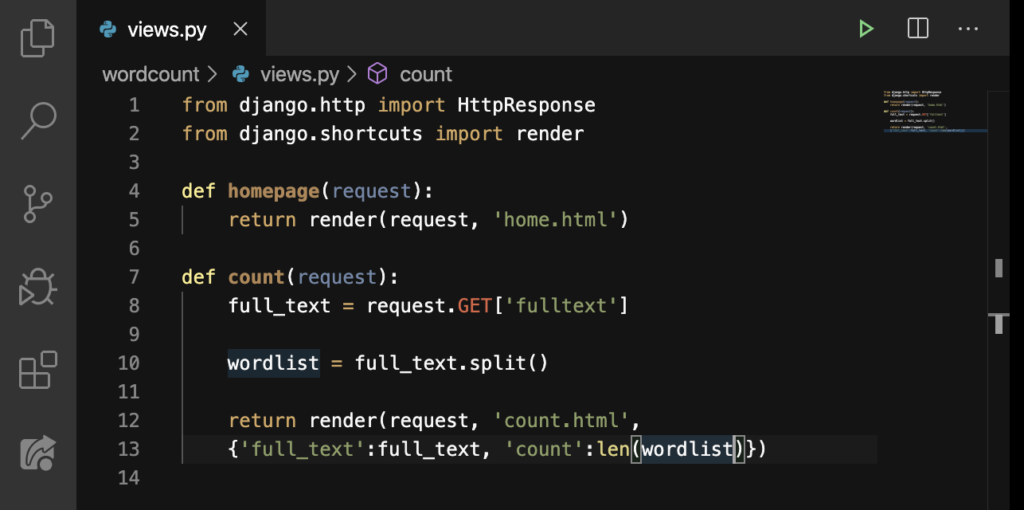
Now, let’s add these code from Line 12 down to Line 21:
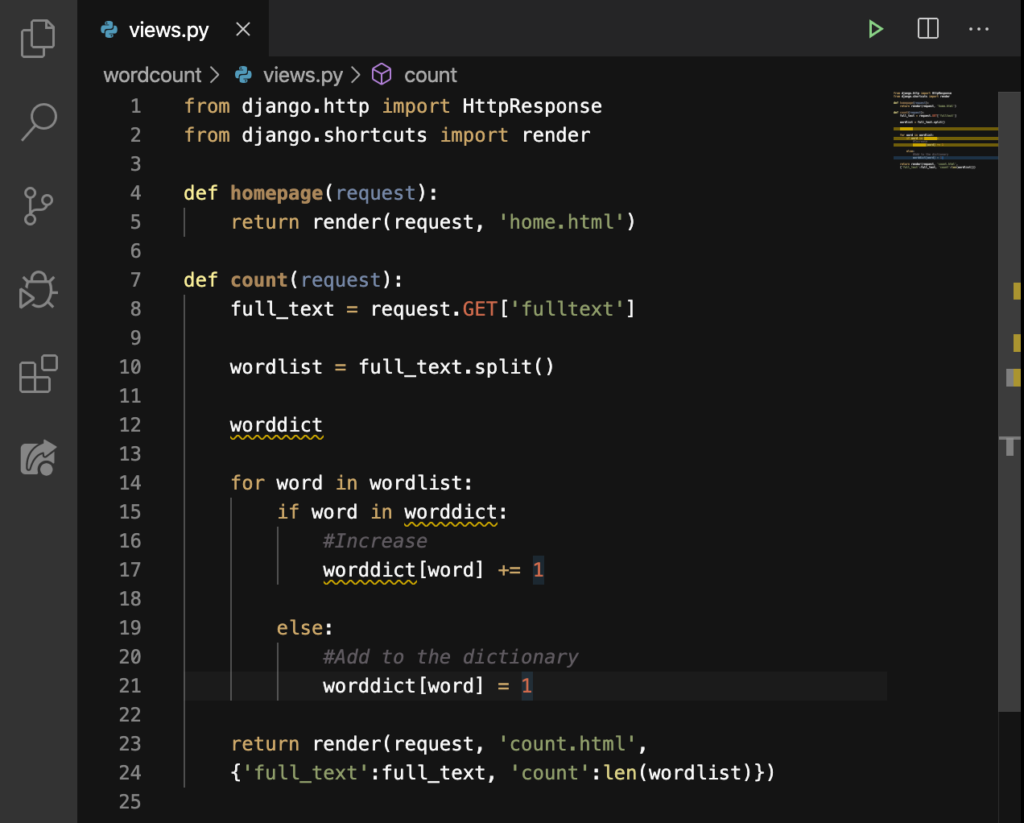
Alright. First, we’ve created a dictionary, worddict on Line 12. Then, we made a for loop for that dictionary. This for loop will loop through all the words inside our wordlist. If a word is in our wordlist already, Line 17 executes. What does it do?
Well, this line commands Django to add 1 to the value of the key. While on the else block, if a new word is seen, this will be a new pair of key and value in our worddict dictionary. Now, let’s add a new pair of key and value to our dictionary on Line 24:
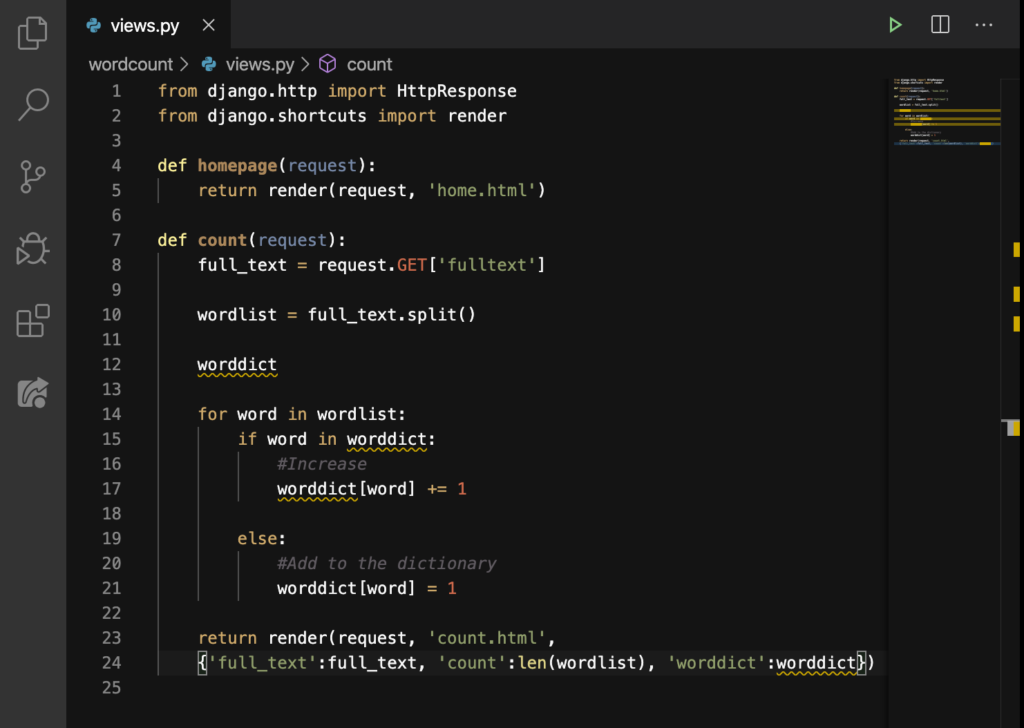
This will display a whole dictionary of words and their own density or occurrence. But, we should also use this here on our count.html:
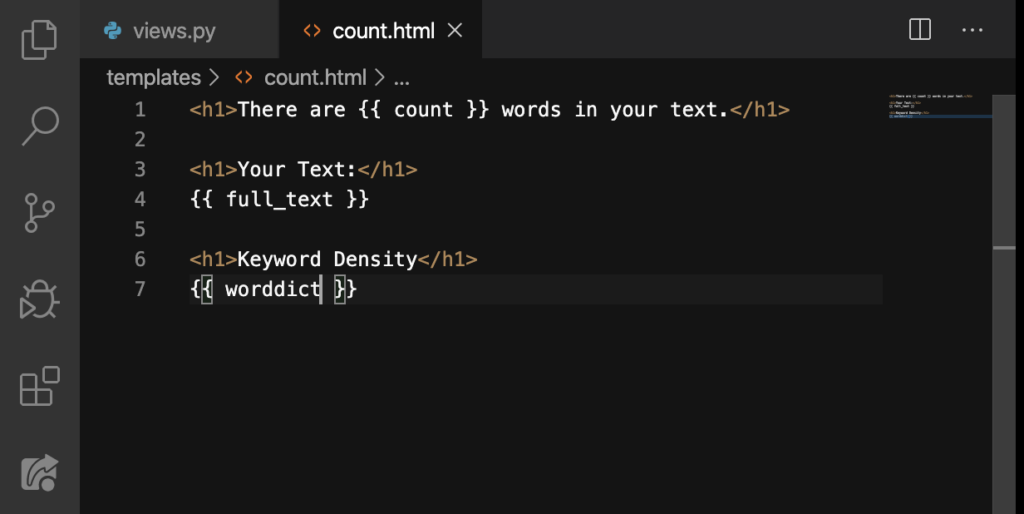
You know, I think we should put these dictionary keys inside a pair of paragraph tags, like this:
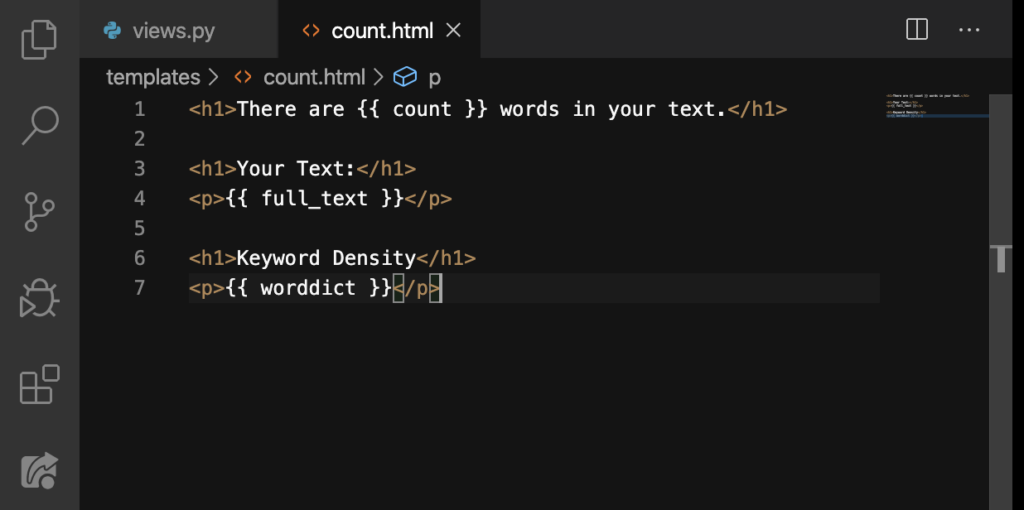
Ah, that’s better. After that, let’s go back to our website:
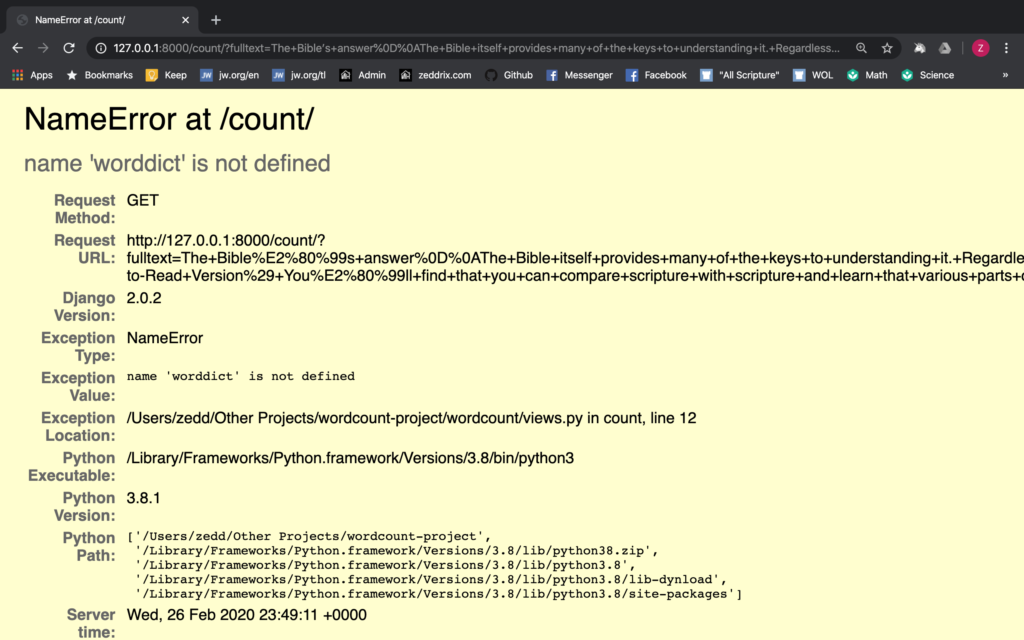
Woah! Why? If you didn’t have this same page like mine because you’ve found out the error a while ago, good job!
Spotting the Error
Did you spot the error in our code? This is a little pop quiz for you. Particularly, this yellow page says: “name ‘worddict’ is not defined“. Looking at the Exception Location will really help. It says that the error occurred on Line 12 in our views.py.
So let’s take a look at that line inside our views.py:
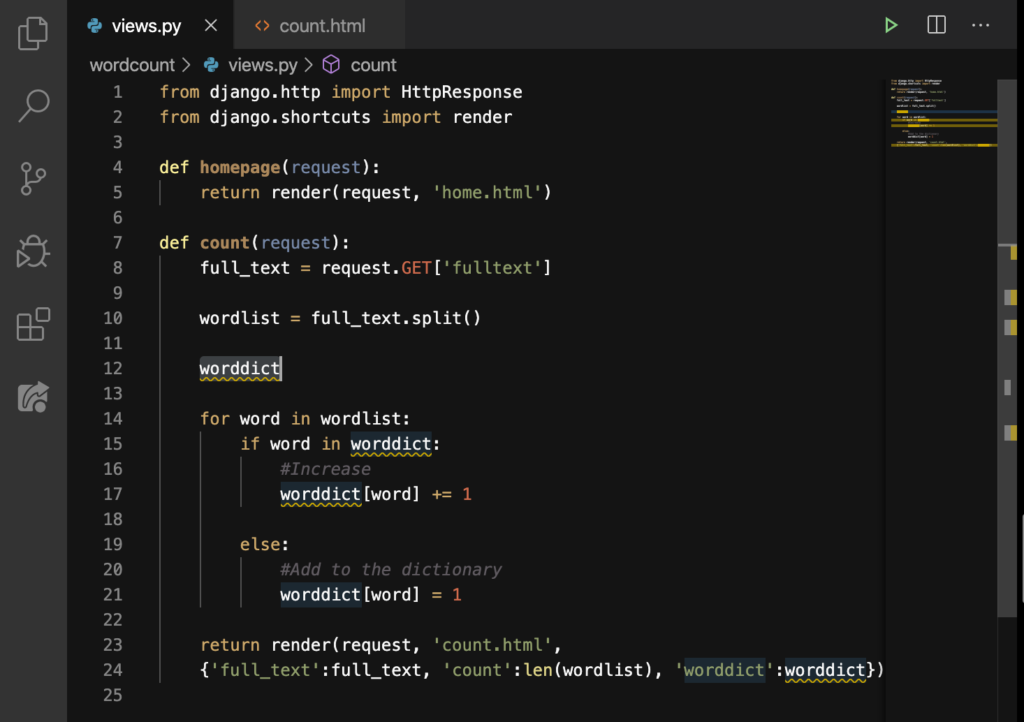
Did you see it? Additionally, do you understand why this line is an error? In fact, we can point our mouse at that worddict because it looks like it wants to say something to us:

So, do you understand? It is an undefined variable. Namely, we want to say that this is a dictionary variable. But for Python, it doesn’t know if it is a tuple, a list, a dictionary, a normal variable, etc. So we’ll do this:

That’s a lot nicer. Now let’s see what displays:
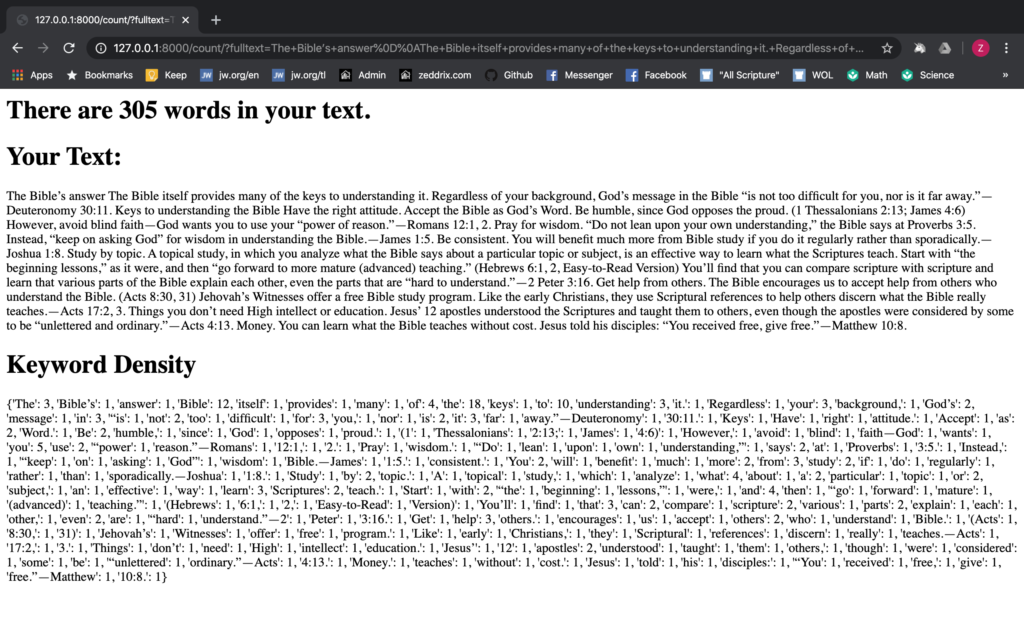
Good. You can take a look at all of these if you want. The way it works is just easy. The key is the word; the value is the density. You know, if this is enough for you, (to have a dictionary), you can settle with it.
But I think it would be better if everything is enumerated. Besides, there are a lot of other things that I want to teach you, so I suggest you go on. Let’s get the keyword density using Django, but enumerated this time.
Enumerated
Let’s go to our views.py:
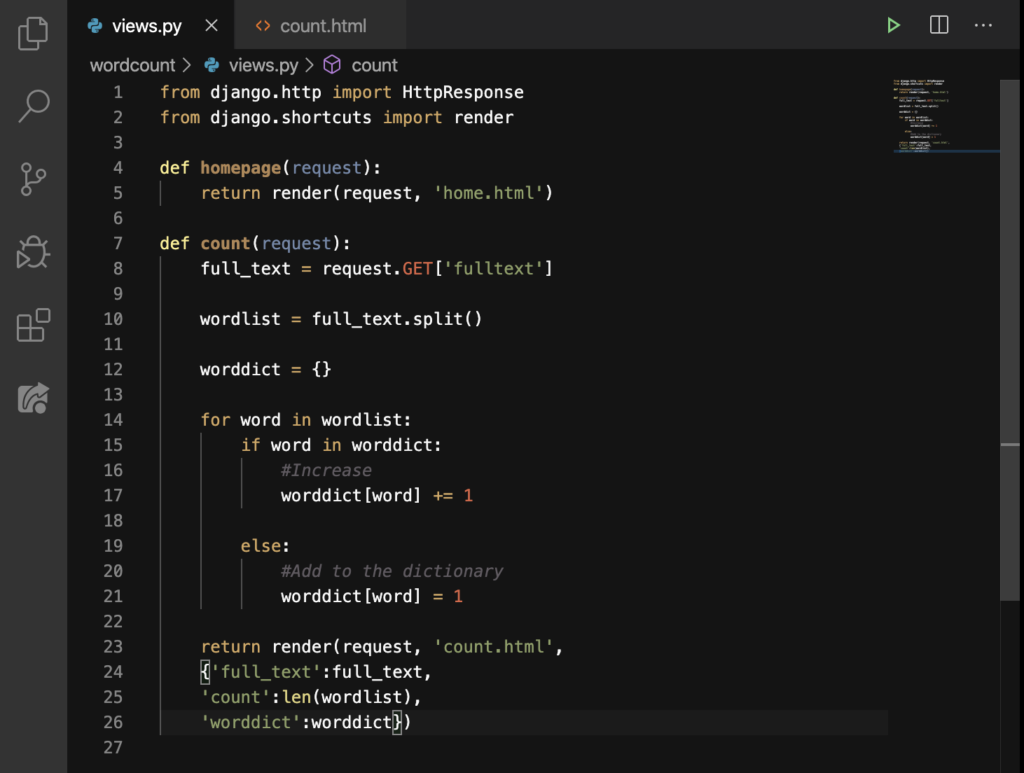
Now let’s put .items inside our dictionary as shown here on Line 26:
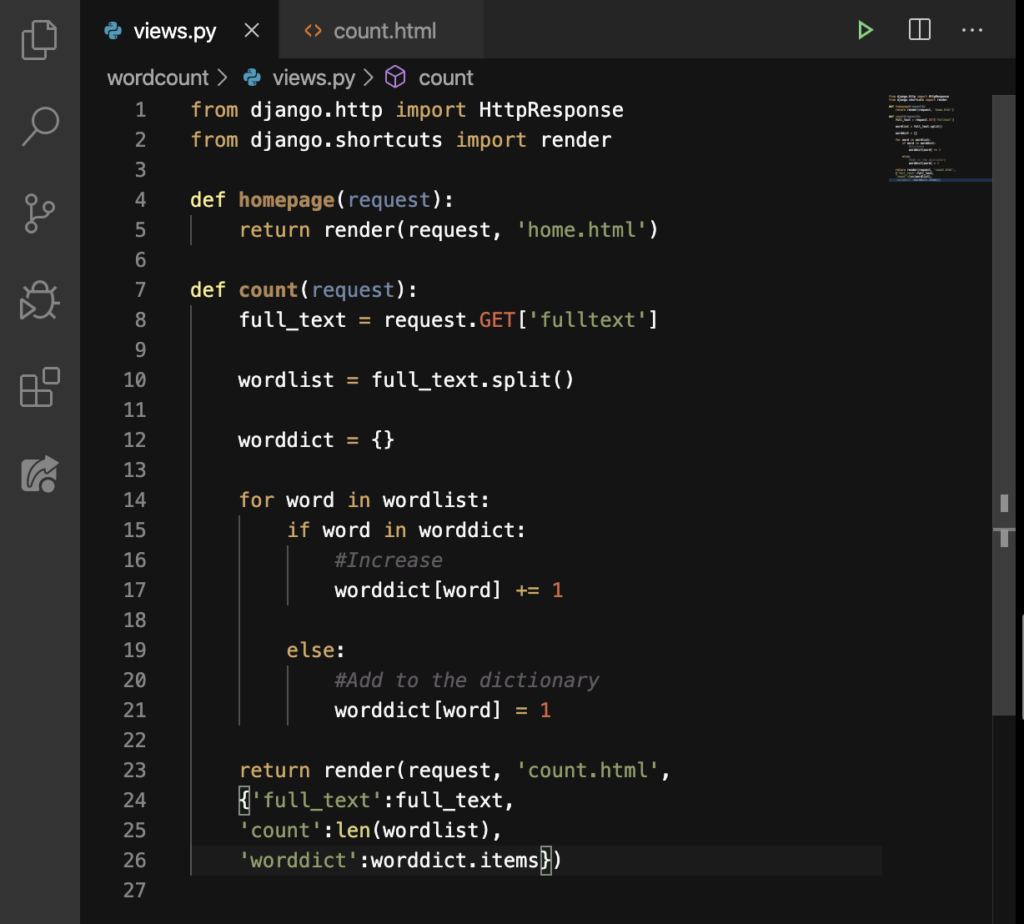
By adding that .items, we turned the worddict into a list. Alright. After that, let’s go inside our count.html and delete this line:
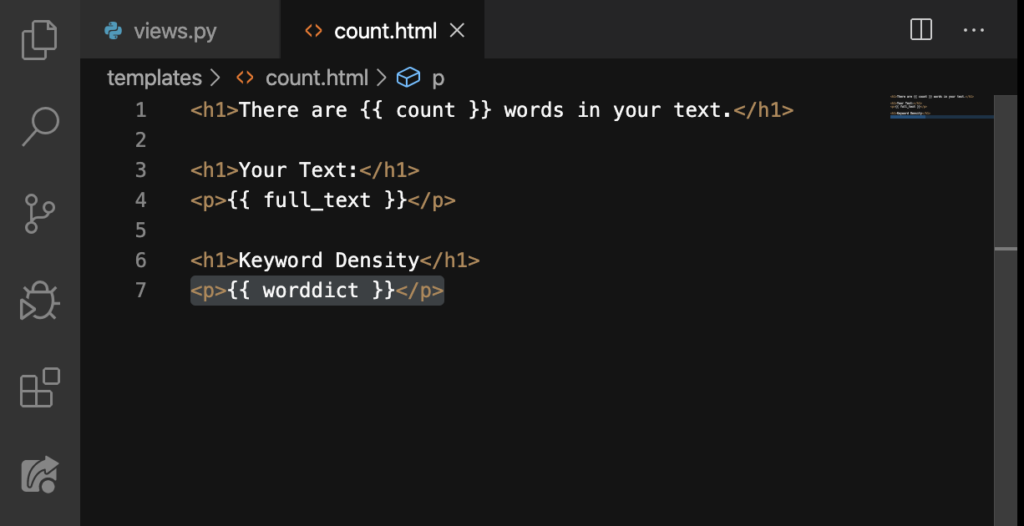
DELETE. And then let’s make a for loop:
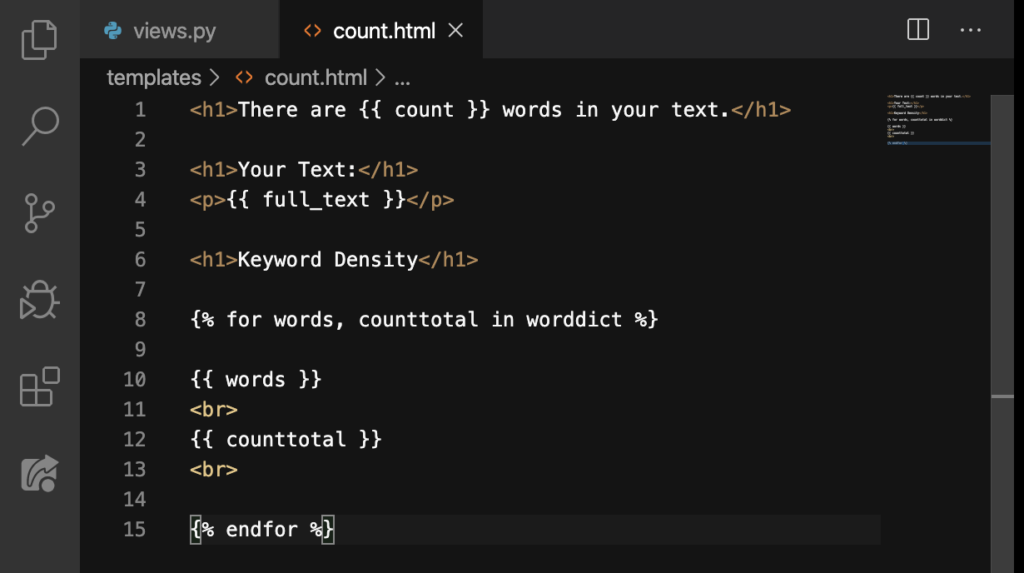
Now let’s see what happens here:
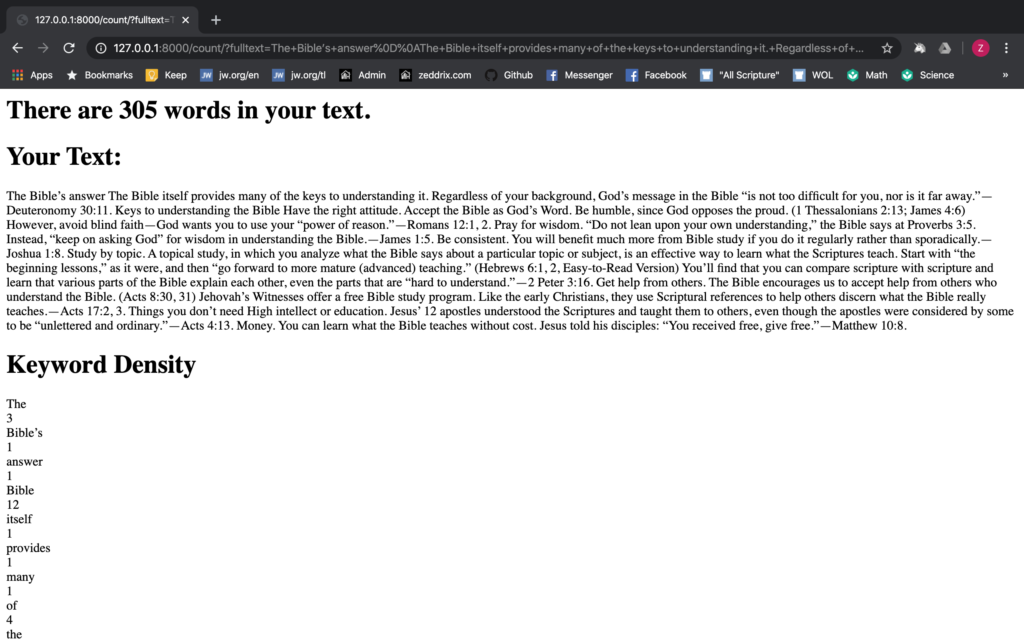
Legit! But this seems a little random. So let’s get the keyword density using Django in a sorted manner. Later, you’ll know what I mean by “sorted.”
Sorted Words
Let’s get back to views.py:
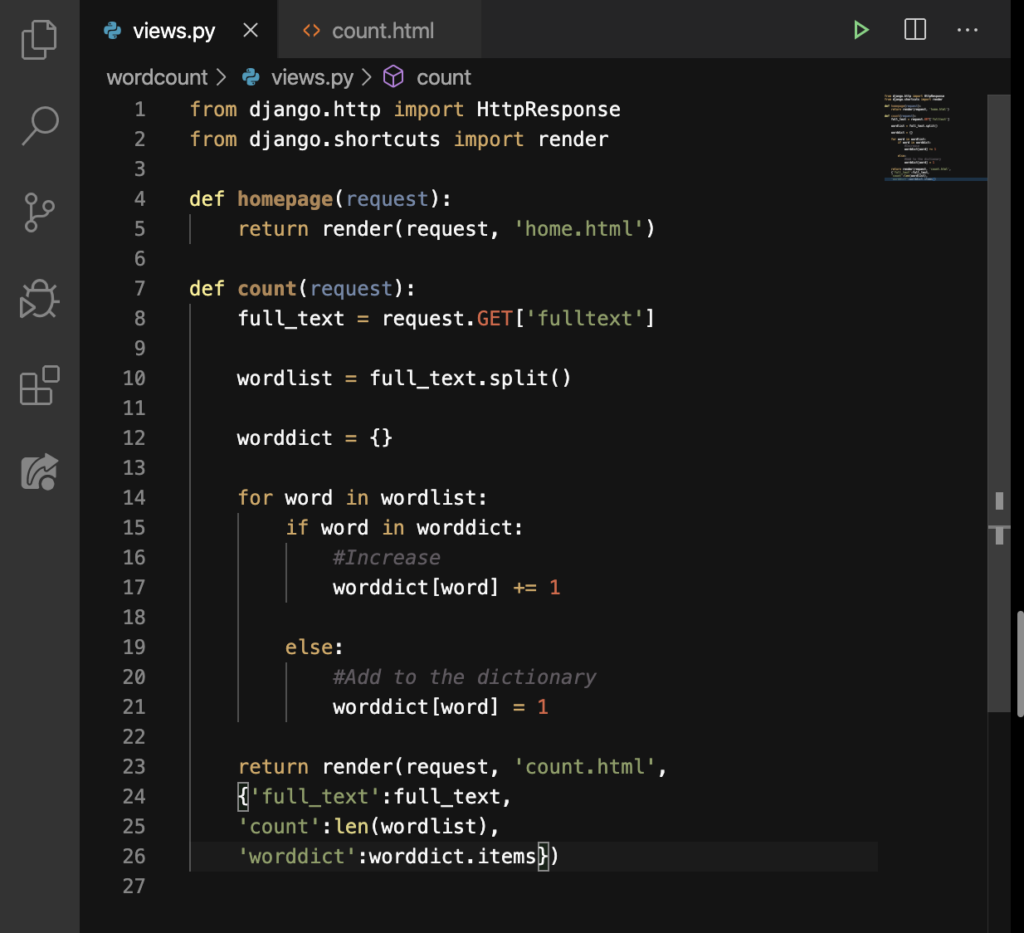
And add the one on Line 23:
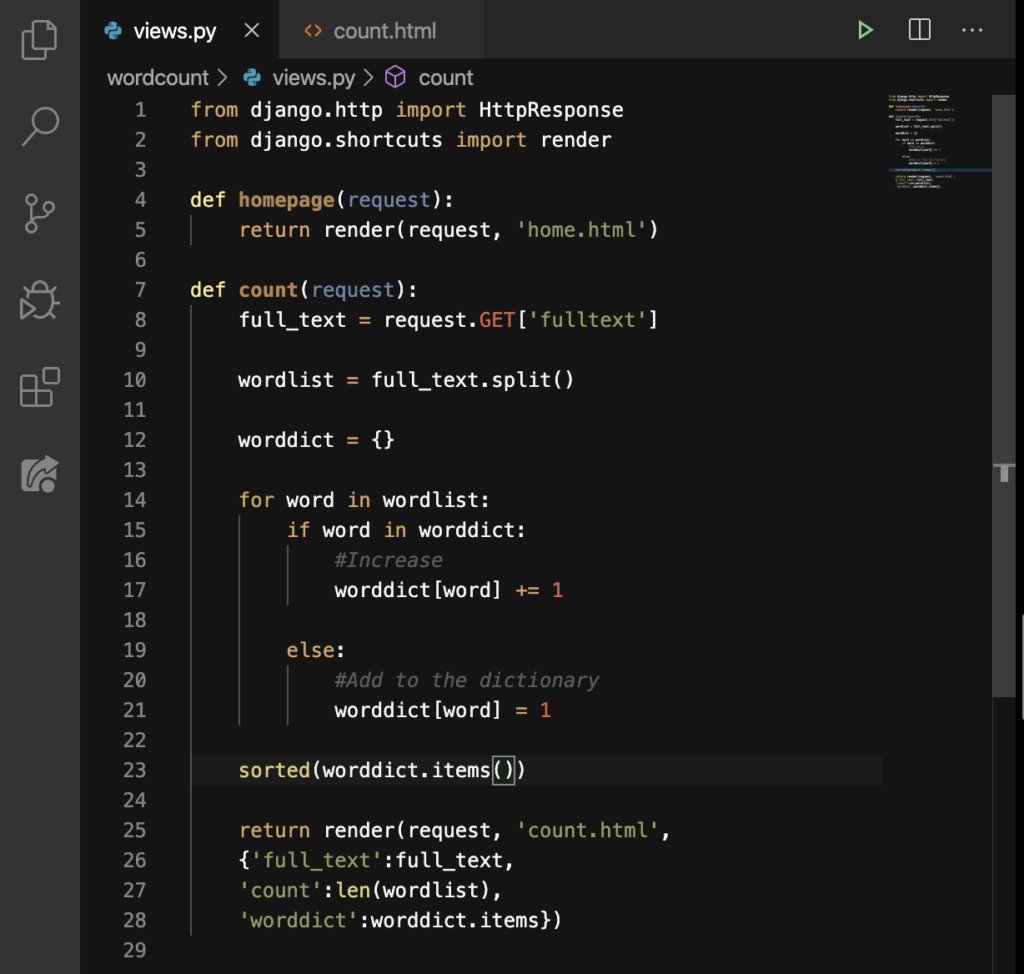
The sorted function, from the word itself, sorts out our items. But we’re not done here yet! We need to do these on Line 3, Line 24, and on Line 29:
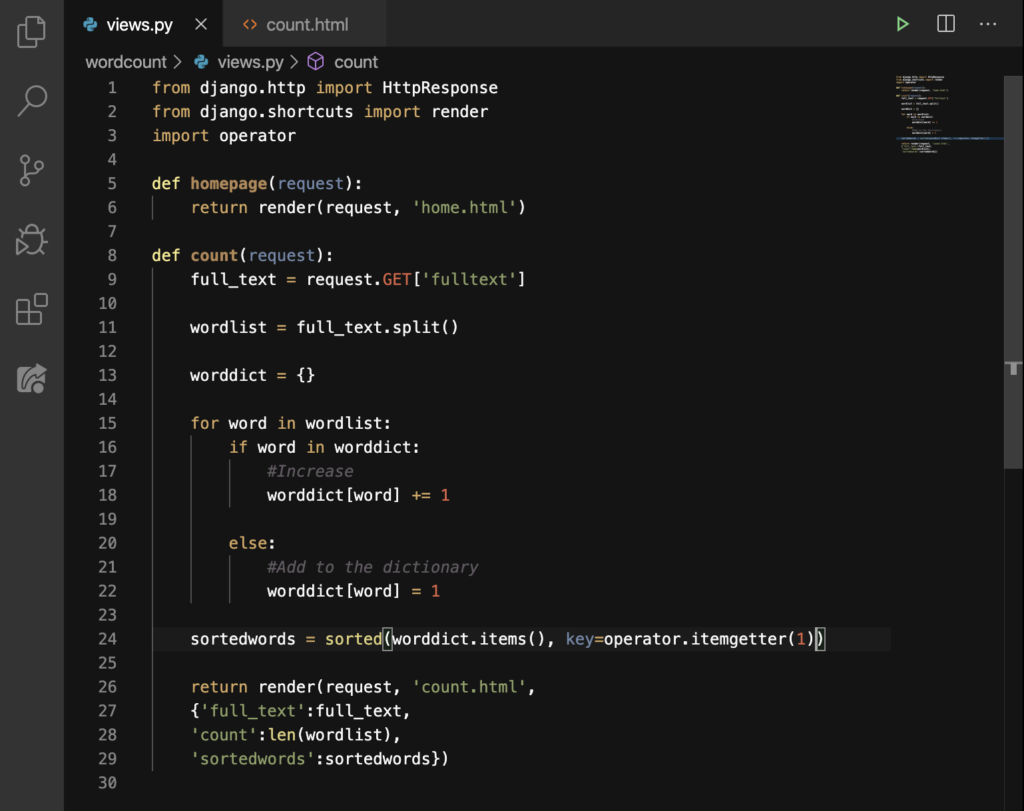
On Line 3, we imported operator. Then on Line 24, we added some things inside the parentheses, and then assigned all this action in one variable, sortedwords. Finally on Line 29, we changed the key to ‘sortedwords’, and its value, sortedwords.
One last step in our count.html:
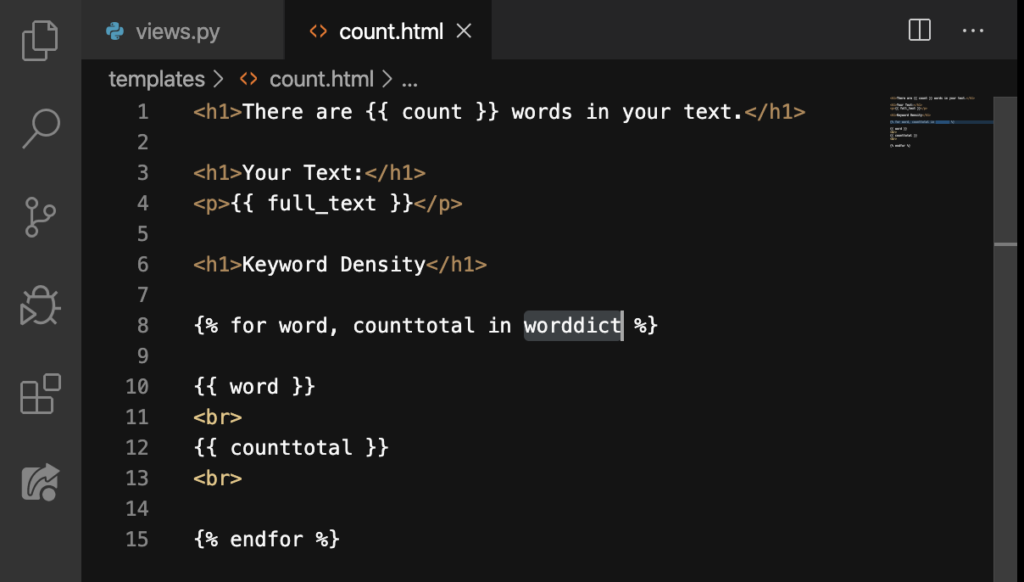
Let’s change that worddict to sortedwords:
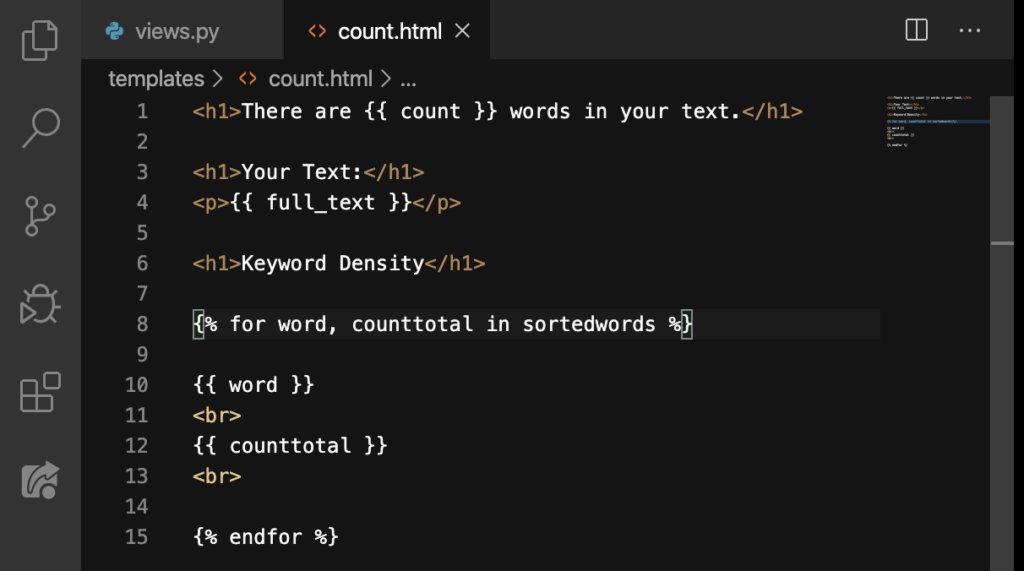
Good. Refresh:
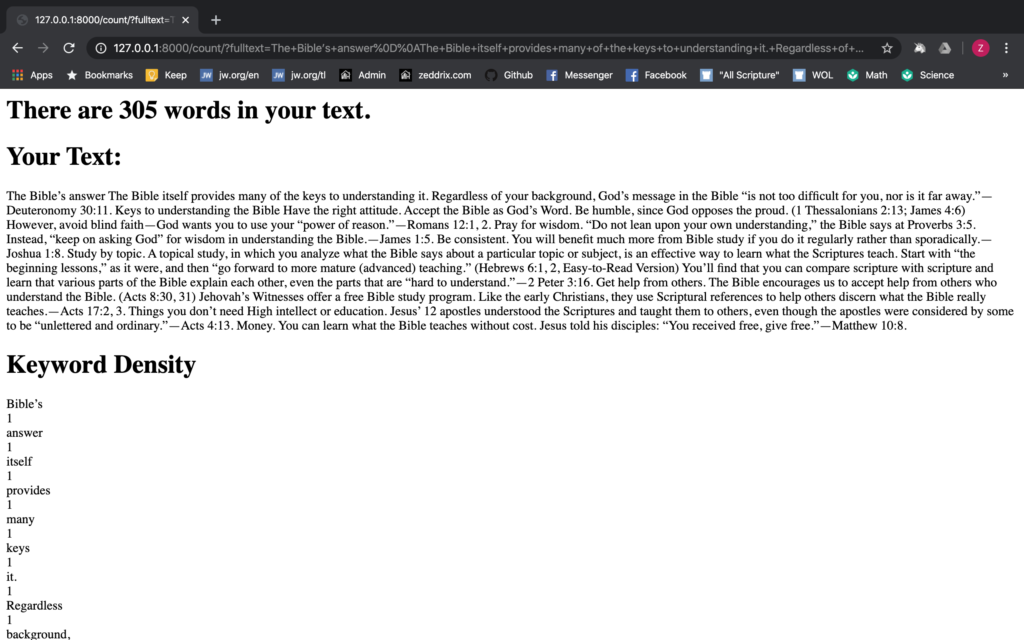
Nice. Now do you know what I mean by “sorted”? Didn’t you notice? All the words with the lowest density were now at the top! And all those words with higher density are now found at the bottom!
But what if I wanted the other way around? What if I want the words with higher density at the top first, before the other words? Let’s add something here on Line 24:
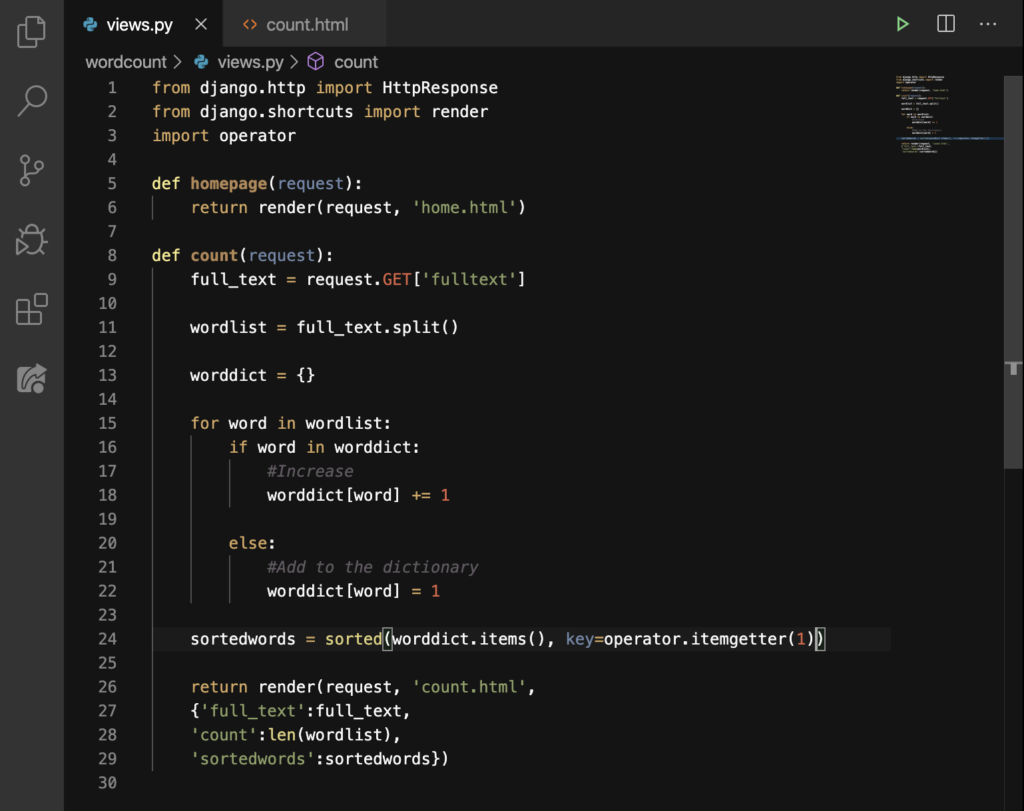
But, actually, before we do, I think it would be more readable to have a newline here:
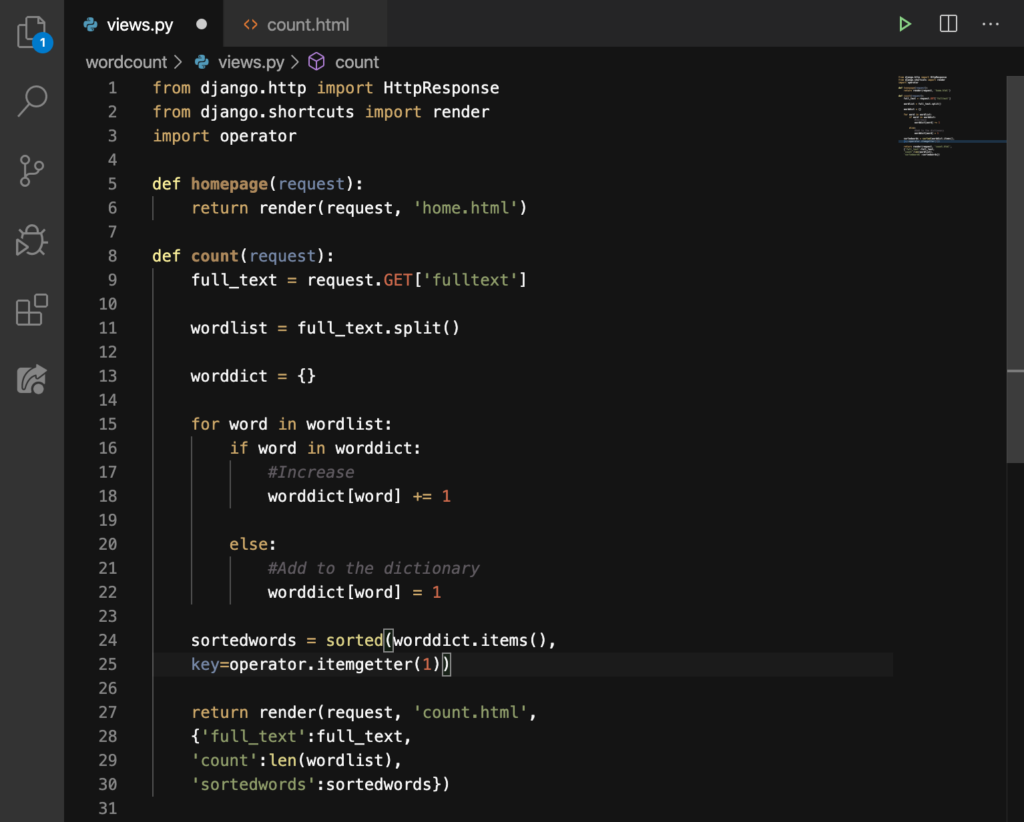
Because this line is about to get longer, we’ll add this code right under it, on Line 26:
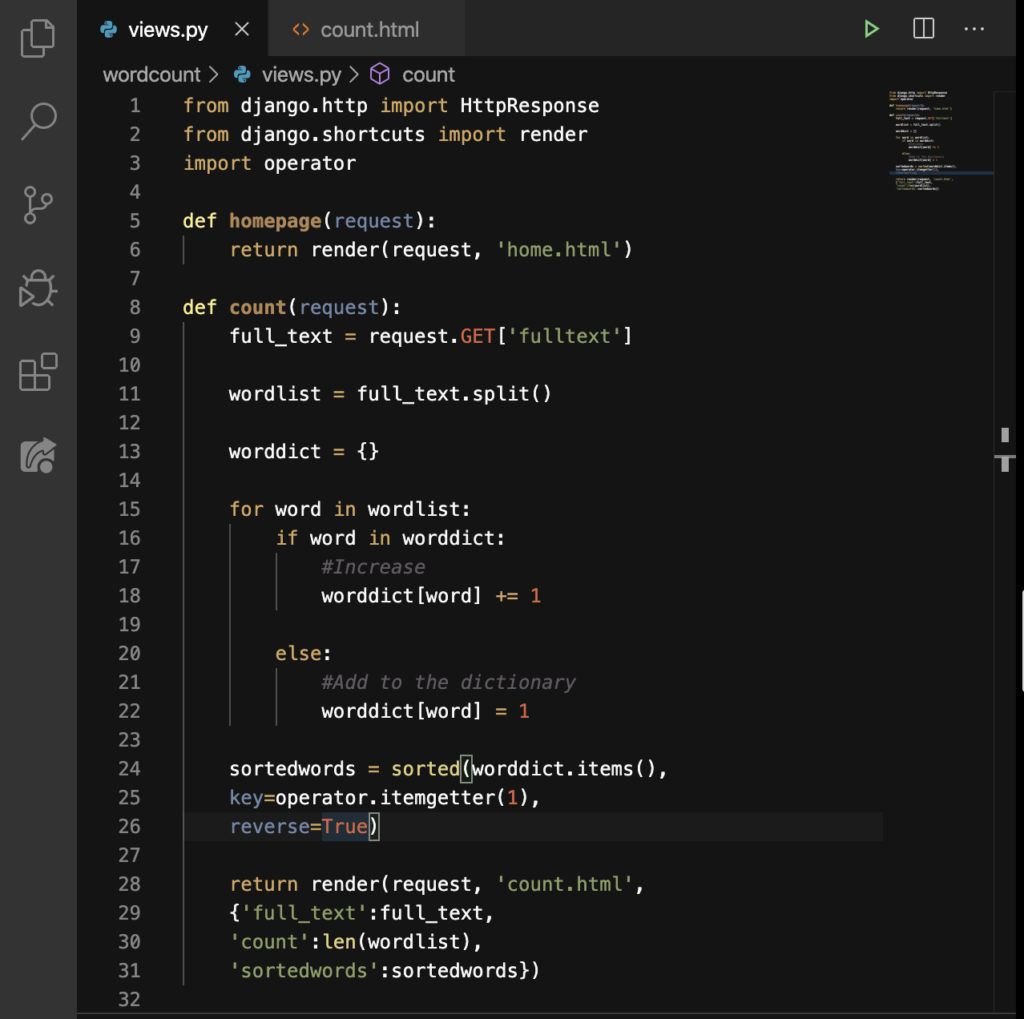
Good. It’s that simple! Add a reverse=True and everything gets reversed! Refresh our site:
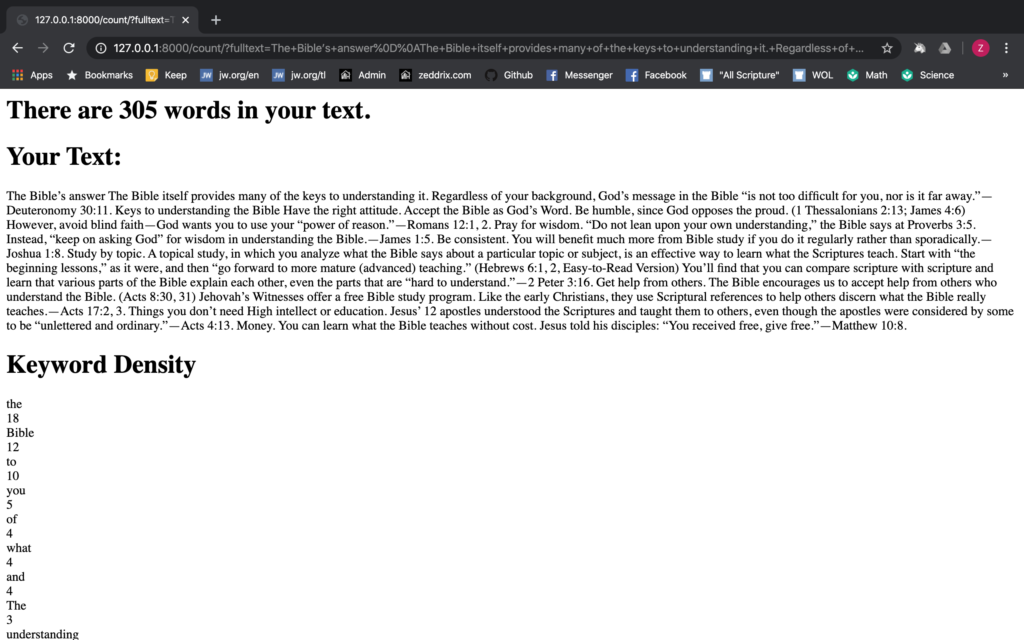
Very nice! Let’s add some readability features here as well.
Using the Dash (-)
On the count.html:
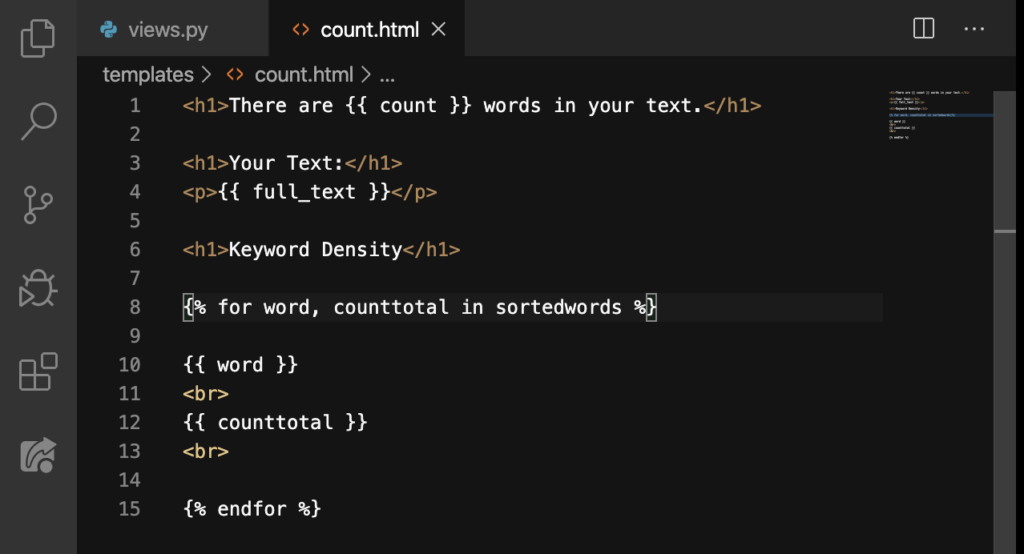
Do this on Line 10:
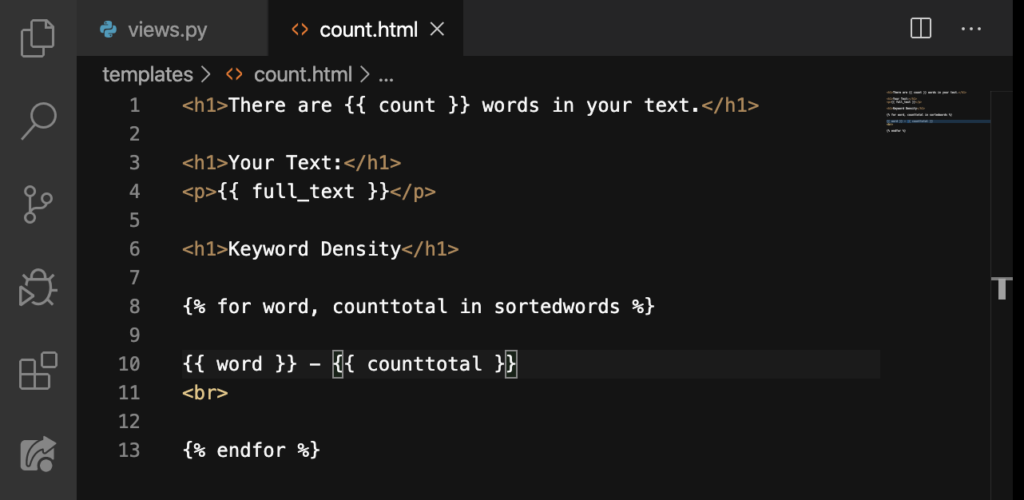
Let’s see why we did that:
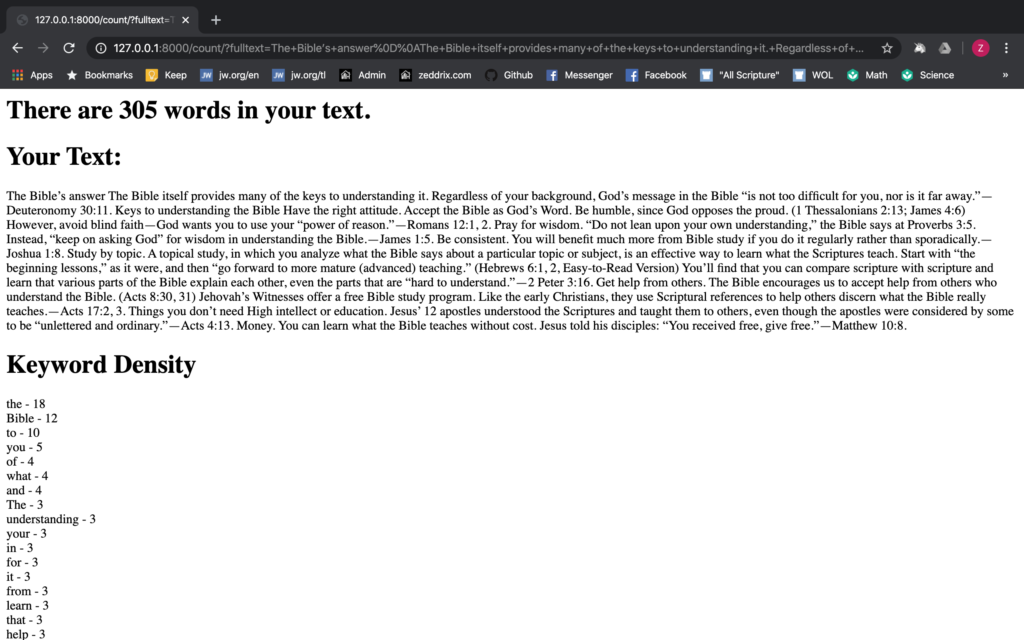
That’s so much better! Now, we’re done here. But, to be honest, it’s not a perfect website. Although we can do something more complex with Django and Python in order to achieve a very ultimate analysis like that of wordcounter.net.
For example, we don’t want to include the word “the” on our Keyword Density. You can do that on your own. However, I’ll have to remind you that we made this word counter website just to learn the basics of Django with Python.
But on the next lesson, I’m gonna teach you something that’s going to improve the functionality of this website. By the way, you did a good job. Keep it up!