Previous Lesson: Lesson 14: Boolean Logic in Python
In the previous lessons, we’ve learned a lot about lists. But what if you want to have a list up to ten million? Range in Python to the rescue!
Mac time! See if you can do this: I want you to have numbers 1 to 9 printed out in the Terminal as a list. Now how would you do it? Do it in ex12.py. Probably, you’ll do it like this:
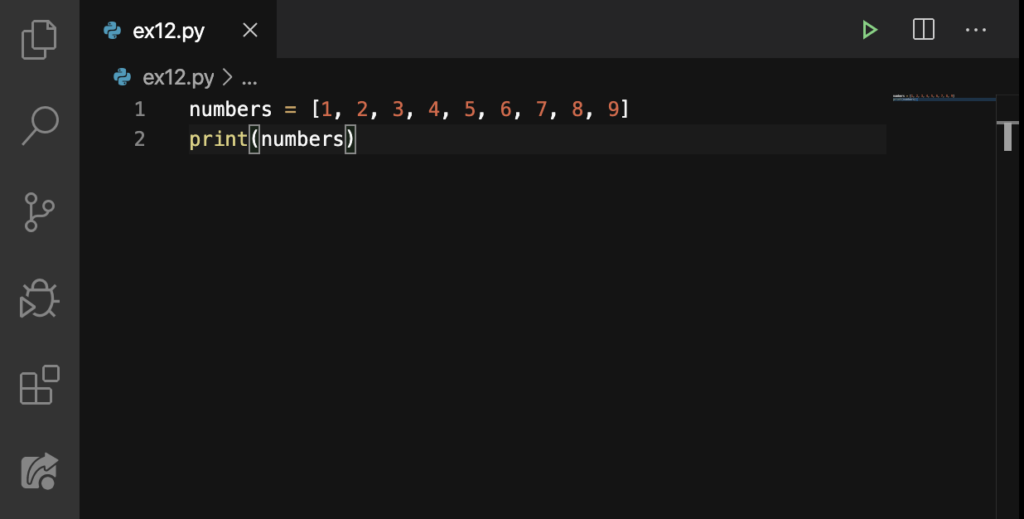
Let’s try this:
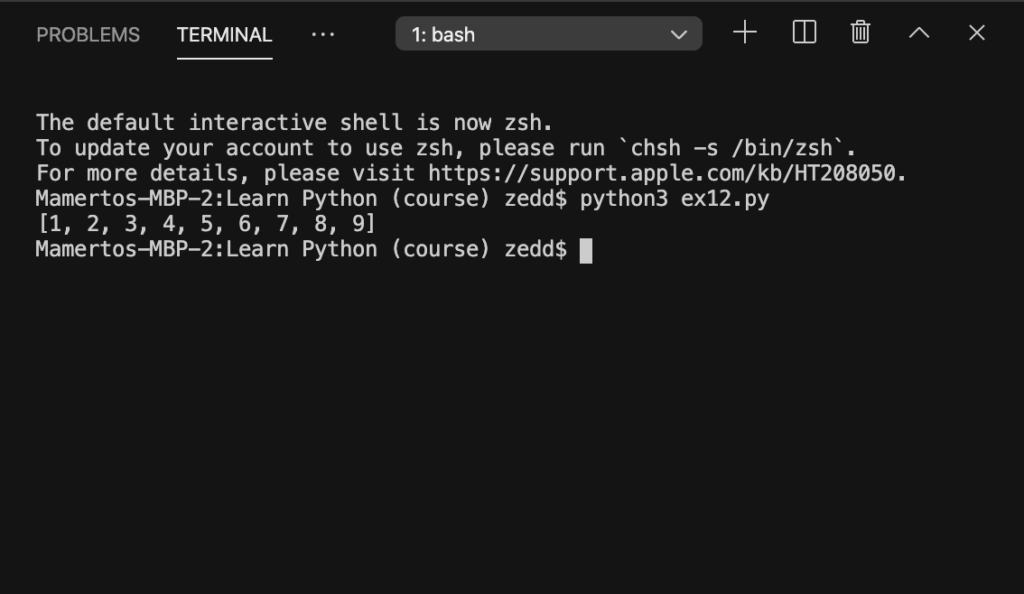
If you did the same thing, you did a great job! But, can you do what I will request next? I want you to make your Terminal return numbers, not just 1 to 9, but 1 to 1,000 in a list. Would you type every single number until you get to number 1,000? You wouldn’t do that, would you? I wouldn’t either.
So let’s get rid of this code and I’ll teach you how we can do this. And this would be a lot easier:
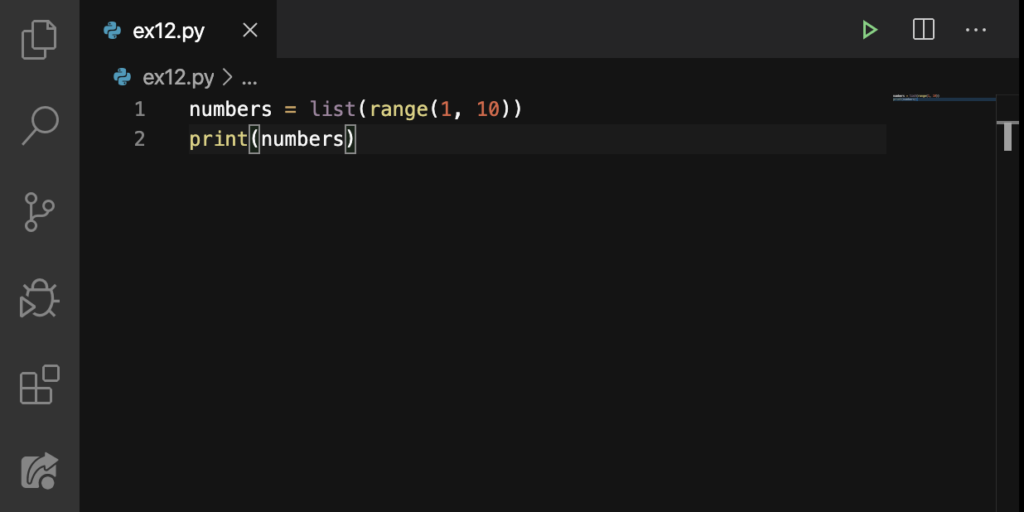
I introduce to you: range. How does this work? The first argument the number that you want to start. And, as you have guessed, the second argument is your last (plus one) number.
But, what is that list beside the range for? You wanna know what happens if we remove this? Then let’s try removing it:
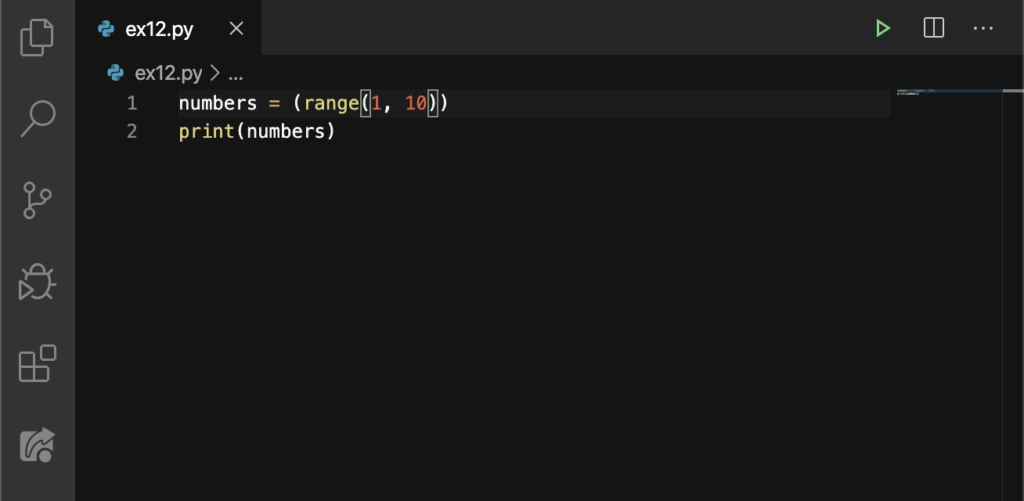
Alright. Before you run this on the Terminal, I want you to click on that plus sign (+) to create a new separate Terminal. It’s more like a new sheet of paper but our first Terminal is not gone yet.
Anyways, let’s see what happens now. But I’ll tell you that the result will not we are expecting:
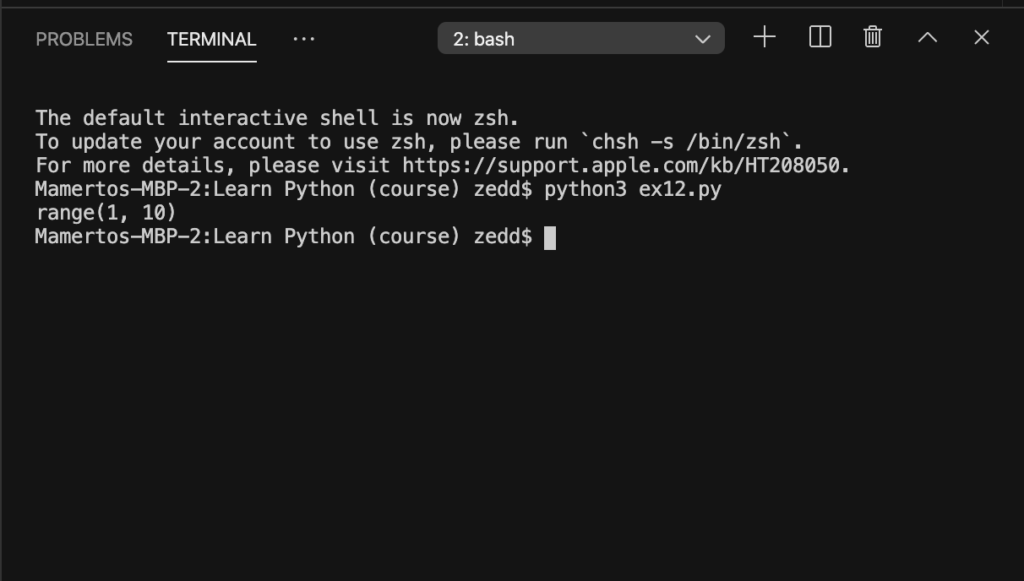
This sucks right. So that’s why that list is important. That’s because it will not print out the numbers we want as a list; it will just print the range(1, 10). But, let’s type that list back.
Before you run this again, click on that trash can icon to get rid of our 2nd Terminal. Then let’s run this to see that we got it all right:

Good! You might have noticed that we’ve typed 10 as the second argument for our range, and yet it didn’t show up on our list. Why is that? Just as we have just learned about lists, it is because they are zero-based.
But if you don’t remember that we’ve talked about that, then I’ll ask you this to get a refresh: What number or index will you use to access or get the first element in a list? That’s right, we use [0]. In the same way, we take the tenth element from the list by using [9]. So to have 9 printed out on our Terminal using the range, we are gonna use 10.
With that in mind, to have a list of numbers 1 to 10, what number should we have as our second argument to have 10, too? Right again–we should type in 11 as our range’s second argument. Let’s do it:
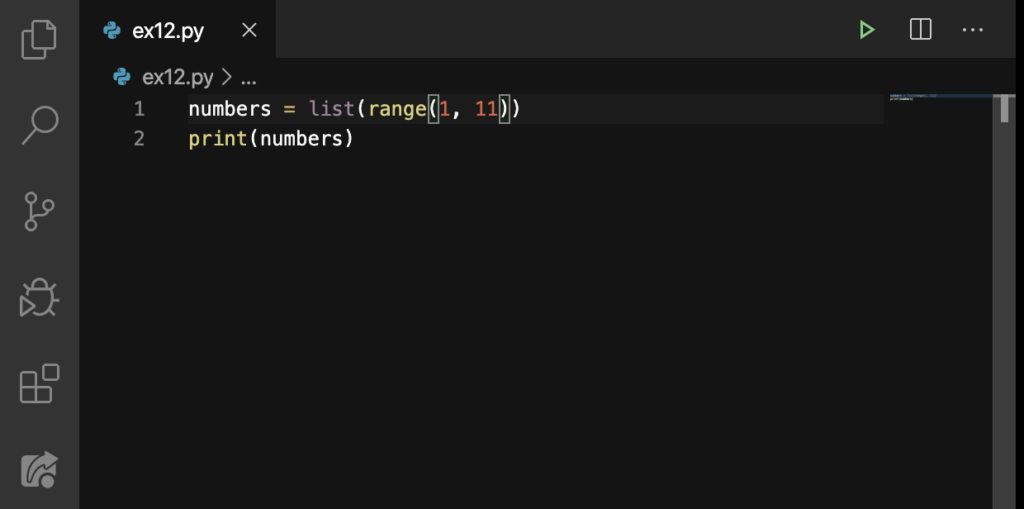
Let’s see if it’s really 10 that gets printed out here using 11:
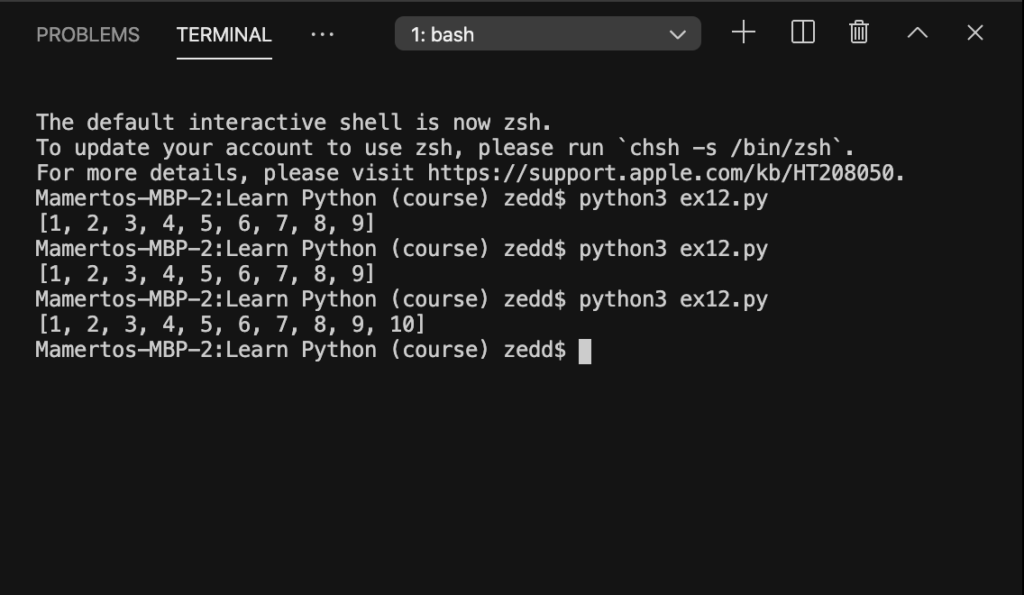
There you go! So let’s do what I wanted, my challenge: Make a list from 1 to 1,000. Just take note that I want the last element of the list to be 1,000, savvy? I want you to do this first before you look at what I would do. Only by then you could look at my solution.
Note: You can’t do 1,001 as the second argument. The Terminal will return “invalid syntax.“
Here’s how I did it:
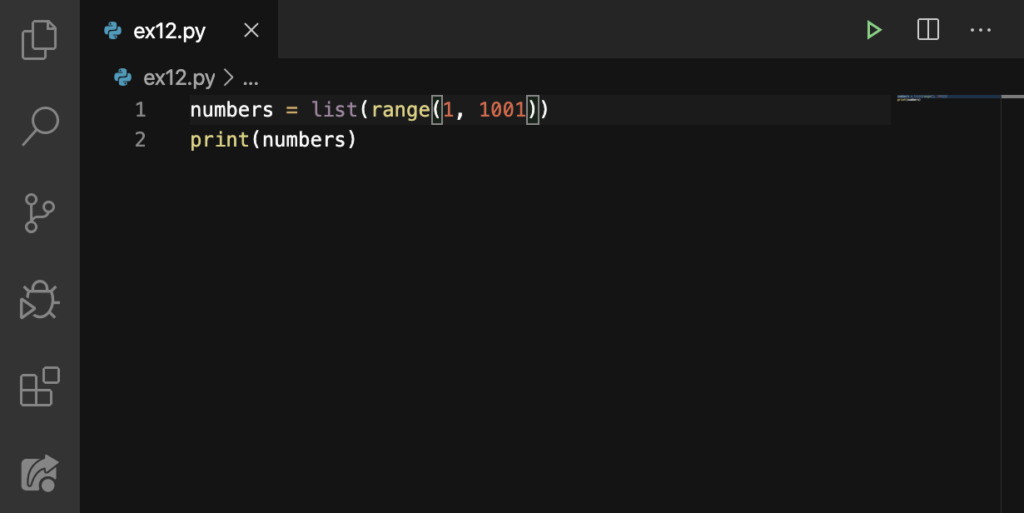
Additional Note: You can’t use 1,001 as the second argument because Python will think that 1 is our second argument; 001 as our third argument.
Tricky note, eh? Okay, let’s run this:
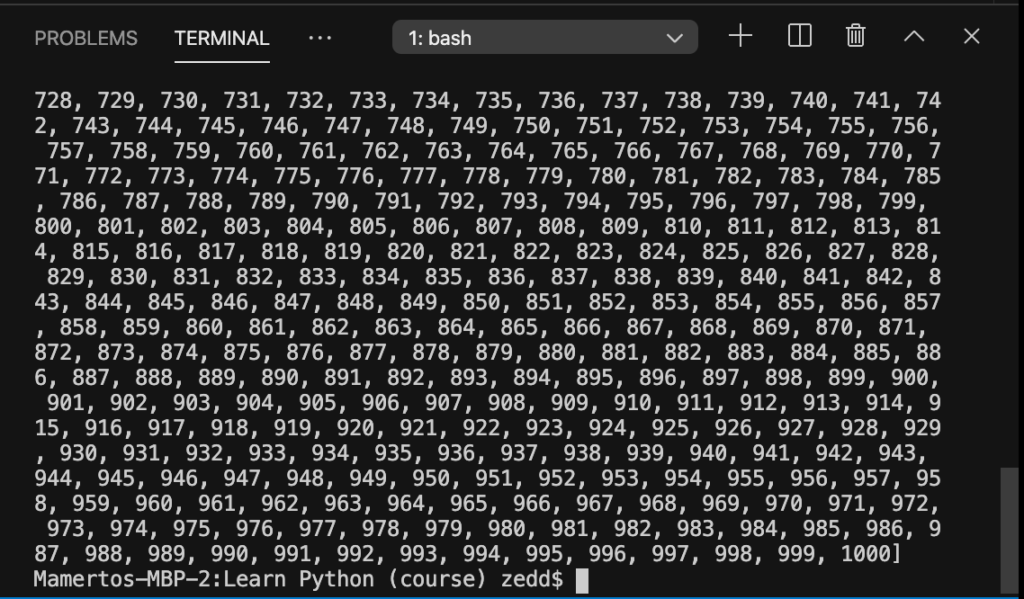
We’re thankful that this is possible with just a small chunk of code. Or else, we would be typing all of these all day, one by one. So now, I want you to play around this code; try removing things; try adding things; and try changing some things. See what other things you can do with this knowledge. It would be delight if you’ll share it with me down on the Comments if you want!
But what if you want to have a list that skips count by 2, or 3, or 10? Is that even possible?
The 3rd Argument in Range in Python
Let’s try it. In the example below, we want to skip count by 10 up to 1,001:

Let’s see how this looks:
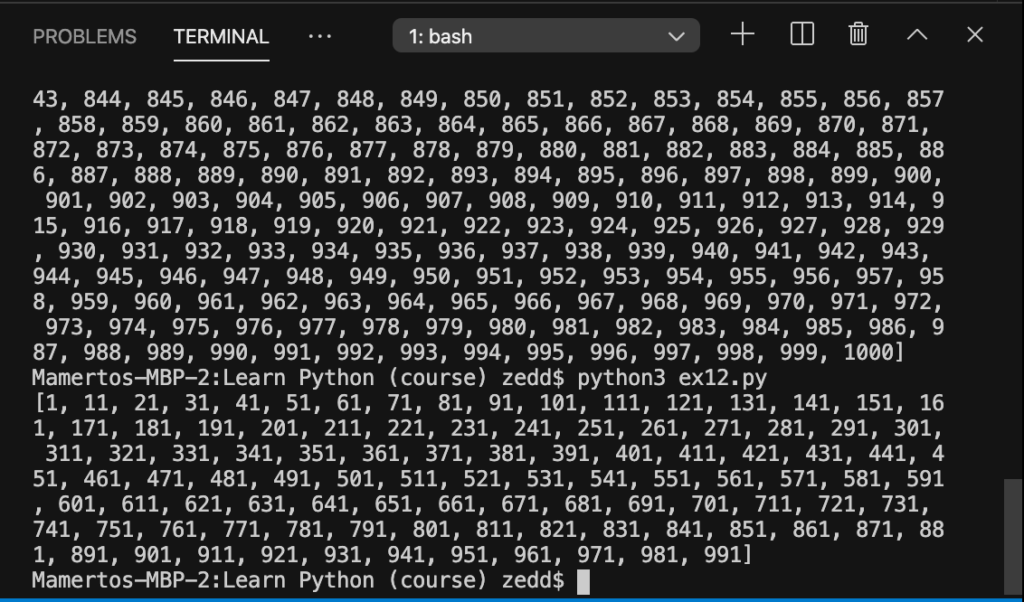
Remember, you can always experiment and play around your code. But just don’t try having a second argument of a billion. I warned you. It really froze my Terminal to the max; unless if you have a third argument of a million, yeah it would work out fine.
But there is something interesting that you still don’t know. You could reverse your lists. We’ll talk about that in the List Slices lesson. But before we head on to that, I just want to talk about some things that is very similar to lists: Dictionaries.