Previous Lesson: Lesson 6: If Elif Else Statements in Python
Operators and delimiters in Python play an essential part in coding. In this lesson, we’ll see what many of them can do and how we’ll use each one of them. We’ll try all of the following examples on a new file: ex5.py.
Additionally, I just want you to know that this is your time to experiment whatever you’d like to experiment with each operators. So this means that you don’t have to copy everything that I do exactly. Instead, you can have your own versions.
By the way, what are Operators and Delimiters in Python? Operators are operations that we use in mathematics. While Delimiters are symbols that we use to compare two or more values. Let’s start!
Plus/Add Operator (+)
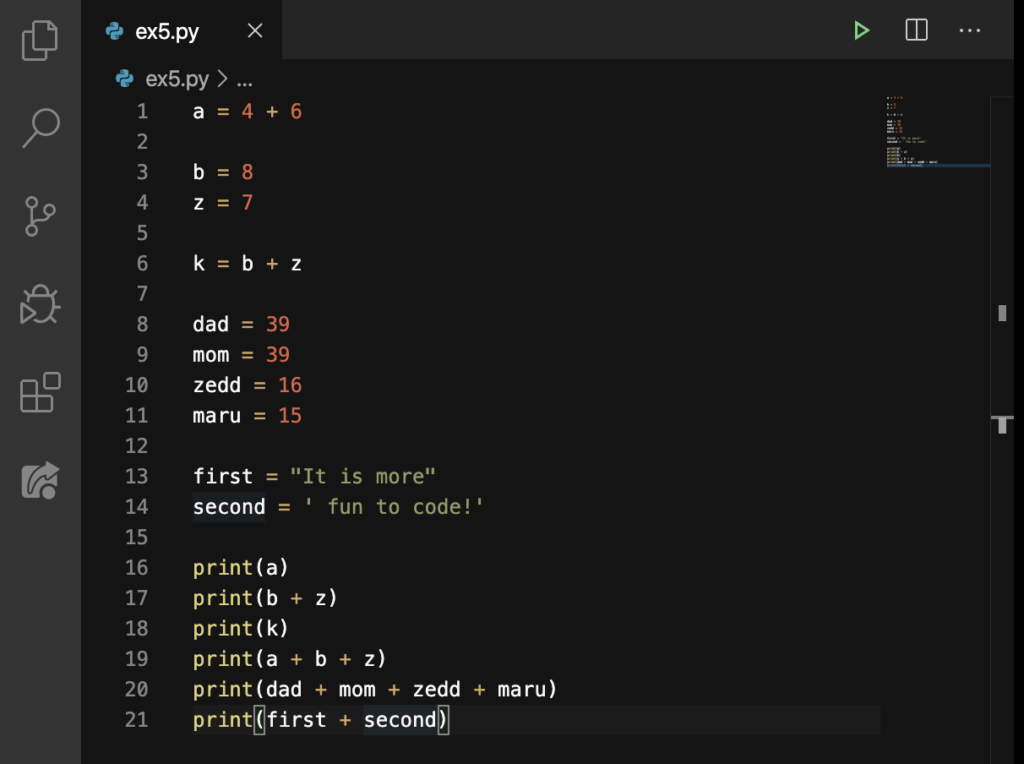
- Line 1 assigns variable a to a mathematical statement of addition. Then Line 16 prints the sum of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the sum of these on Line 17.
- Line 18 assigns the output of Line 17 to the k variable.
- Line 19 just adds up the value of the variables a, b, and z.
- Line 20 just adds up the value of the variables dad, mom, zedd, and maru.
- We assigned a string to each of the variables on Line 13 and Line 14. Then on Line 21, we added up or united both strings using the plus symbol (+).

Minus/Subtract Operator (-)

- Line 1 assigns variable a to a mathematical statement of subtraction. Then Line 13 prints the difference of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the difference of these on Line 14.
- Line 15 assigns the output of Line 14 to the k variable.
- Line 16 just subtracts the value of the variables a, b, and z.
- Line 17 just subtracts the value of the variables dad, mom, zedd, and maru.
- Unlike the plus (+), we can’t add up or unite both strings using the minus symbol (–).
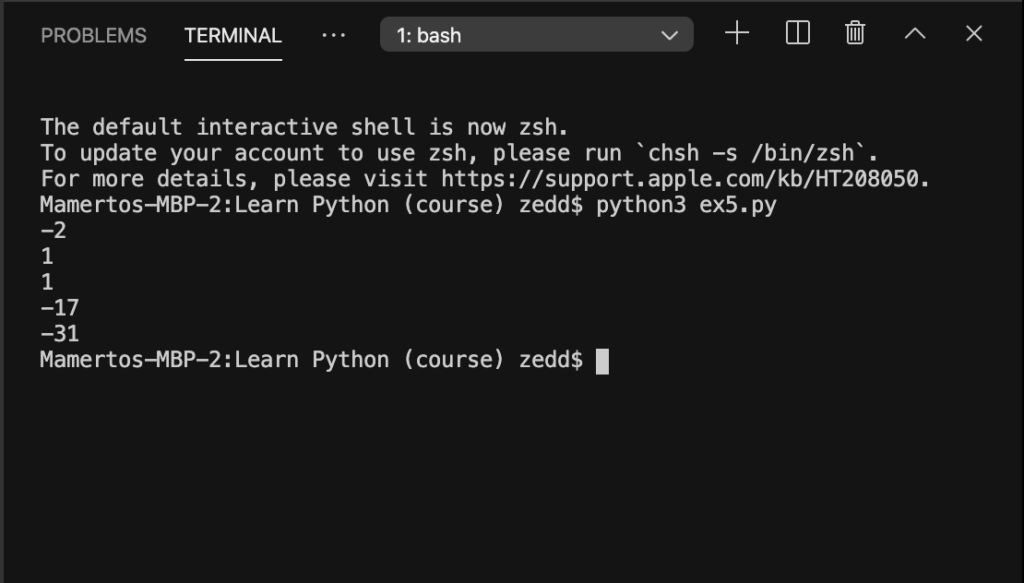
Times/Multiply Operator (*)

- Line 1 assigns variable a to a mathematical statement of multiplication. Then Line 15 prints the product of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the product of these on Line 16.
- Line 17 assigns the output of Line 16 to the k variable.
- Line 18 just multiplies the value of the variables a, b, and z.
- Line 20 just multiplies the value of the variables dad, mom, zedd, and maru.
- We assigned a string to a variable on Line 13. Then on Line 20, we multiplied or repeated the same string three times over. Which means that we can repeat this as many as we want!

Exponentiation (**)

- Line 1 assigns variable a to a mathematical statement of exponentiation. Then Line 11 prints the result of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the result of these on Line 12.
- Line 12 just exponentiates the value of the variables b and z.
- Line 13 assigns the output of Line 12 to the k variable.
- Line 15 just exponentiates the value of the variables zedd and maru.
- Unlike multiplication, you can’t apply exponentiation or repeat a string as many as you want with **! Rather, it only works with one *. Additionally, when you exponentiate, both should be an int or a variable that is equal to an integer.

Divide (/) Operator

- Line 1 assigns variable a to a mathematical statement of division. Then Line 13 prints the quotient of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the quotient of these on Line 14.
- Line 15 assigns the quotient of Line 14 to the k variable.
- Line 16 just divides the value of the variables a, b, and z.
- Line 17 just divides the value of the variables dad, mom, zedd, and maru.
- You can’t divide a string as many as you want with a slash (/)! Additionally, when you divide, the divisor and dividends should be an int or at least a variable that is equal to an integer.

Modulo (%) Operator

- This kinda something new to our beginners. Modulo first does the operation of division, but it will not return the quotient. Instead, it will return the remainder. So it’s still like operation of division.
- Line 1 assigns variable a to a mathematical statement of division. Then Line 13 prints the remainder of that mathematical statement.
- Line 3 and Line 4 assigns a value to each variable (b and z) and then prints out the remainder of these on Line 14.
- Line 15 assigns the remainder of Line 14 to the k variable.
- Line 16 just divides the value of the variables a, b, and z.
- Line 17 just divides the value of the variables dad, mom, zedd, and maru.
- You can’t divide a string as many as you want with a slash (/)! Additionally, when you divide, the divisor and dividends should be an int or at least a variable that is equal to an integer.

Less Than (<) and Greater Than (>) Delimiters

- The results for the delimiters on the Terminal can either be TRUE or FALSE, depending on the equation trueness.
- Line 1 states that 4 is less than (<) 6. If this is really true, then the Terminal will say TRUE once we try to print it on Line 14.
- Line 3 and Line 4 each assigns a value to a variable. Down to Line 15, these variables (b and z) are compared. If this equation is true, then the Terminal will print TRUE; if not, FALSE. But it surely is true because 8 is really greater than 7.
- Line 6 has k equal to b < z. Referencing to the values of b and z, what is the answer: TRUE or FALSE?
- Line 8 and Line 9 each assigns a value to a variable. Down to Line 18, these variables (mom and dad) are compared. If this equation is true, then the Terminal will print TRUE; if not, FALSE.
- Line 11 and Line 12 each assigns a str (string) to a variable. Down to Line 19, the length of these two strings are compared. If the length of code1 is really greater than code2, then the Terminal will print TRUE.

Equal To (==) and Not Equal To (!=) Delimiters

- This a different example of where you can use == and !=. But, as you can remember, on the previous lesson, we’ve talked about these conditionals.
- Here, == means “equal to“.
- While the != means “not equal to“.
- On Line 1, we have a pretty intermediate mathematical equation. But, as we can remember from our Math class, we can use the MDAS (Multiplication, Division, Addition, Subtraction). But don’t worry; we’ll have our computer take care of this one.
- On Line 3, if a is equal to 0, then “The answer is 0” from Line 4 prints out on the Terminal.
- On Line 5, if a is not equal to -3.5, then “The answer is not equal to -3.5” from Line 6 prints.
- On Line 7, if a is equal to -3.5, then “-3.5 is the answer” from Line 8 prints.
- If a doesn’t meet the conditions, then “Dunno. Sorry.”

greater than-equal (>=) or less than-equal (<=) delimiters

- This a different example of where you can use == and !=. But, as you can remember, on the previous lesson, we’ve talked about these conditionals.
- Here, == still means “equal to“.
- The >= means “greater than or equal to“.
- While <= means “less than or equal to“.
- On Line 1, we have a pretty intermediate mathematical equation. But, as we can remember from our Math class, we can use the MDAS (Multiplication, Division, Addition, Subtraction). But don’t worry; we’ll have our computer take care of this one.
- On Line 3, if a is equal to 0, then “The answer is 0” from Line 4 prints out on the Terminal.
- On Line 5, if a is greater than or equal to 0, “The answer is higher than or equal to 0” from Line 6 prints. So whether the answer for our equation (in Line 1) is greater than 0, or the answer is equal to 0 (put simply, if the answer is 0), then the string on Line 6 prints out.
- On Line 7, if a is less than or equal to 0, “The answer is lower than or equal to 0” from Line 8 prints. So whether the answer for our equation (in Line 1) is less than 0, or the answer is equal to 0 (put simply, if the answer is 0), then the string on Line 8 prints out.
- If a doesn’t meet any of these conditions, then I “Dunno. Sorry.”

Plus-Equal (+=) and Minus-Equal (-=) Delimiters

- On Line 1, we have the variable age assigned with the value 16. On Line 2, we are saying that whatever the value of age, just add this 10 to it. So the age now have the value 26. That’s what += does. It’s like the shortened form of this code:
age + 10 = age (add the value of age to 10 and then assign the sum to the same variable) - Same thing happens with the strings on name. And for this one, the result or the value of name will now be: “My name is Zedd.”

Alright! These are just some of the many other operators and delimiters out there. But I just gave you the ones that programmers really use 90%; the others are more likely 5% used–very rare.
I’ll give you time to memorize all these. Give yourself a time to memorize these. After memorizing, write every operator and delimiter you’ve learned on an index card or on your notebook without looking back to this post. That way, we can remember these and use them on our daily coding when we need them.
Only once you’ve done this, can you move on to our next lesson. But I’ll tell you that I can’t stop you because I ain’t your mama. Still, do it.