Previous Lesson: Lesson 25: Create Your Own Game Using Python
In this lesson, learn the different kinds of errors that we might encounter on our coding everyday. And know the reasons why we need exceptions in Python.
Just to give you a background, exceptions occur when something goes wrong, due to incorrect code or input. And, when an exception occurs, the program immediately stops. Type this in ex22.py:
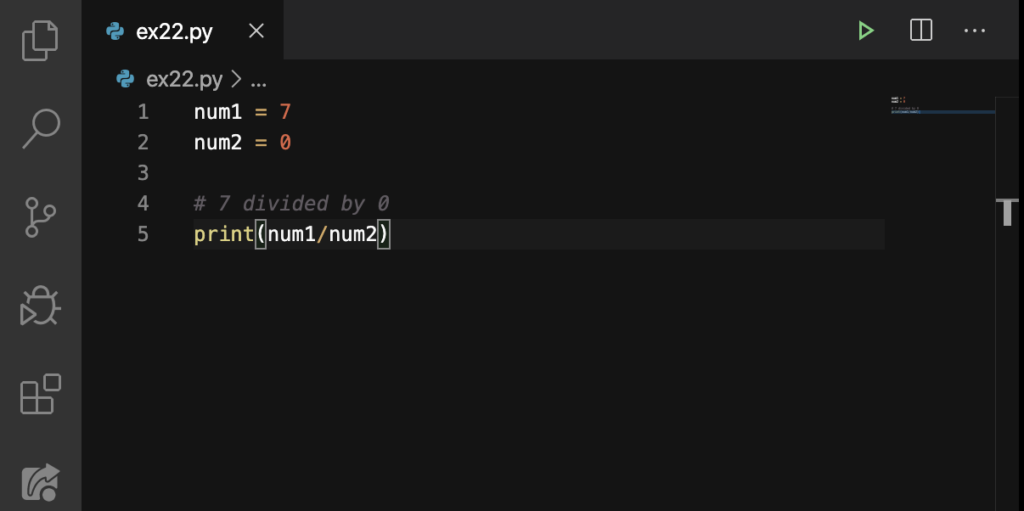
Of course, run this:
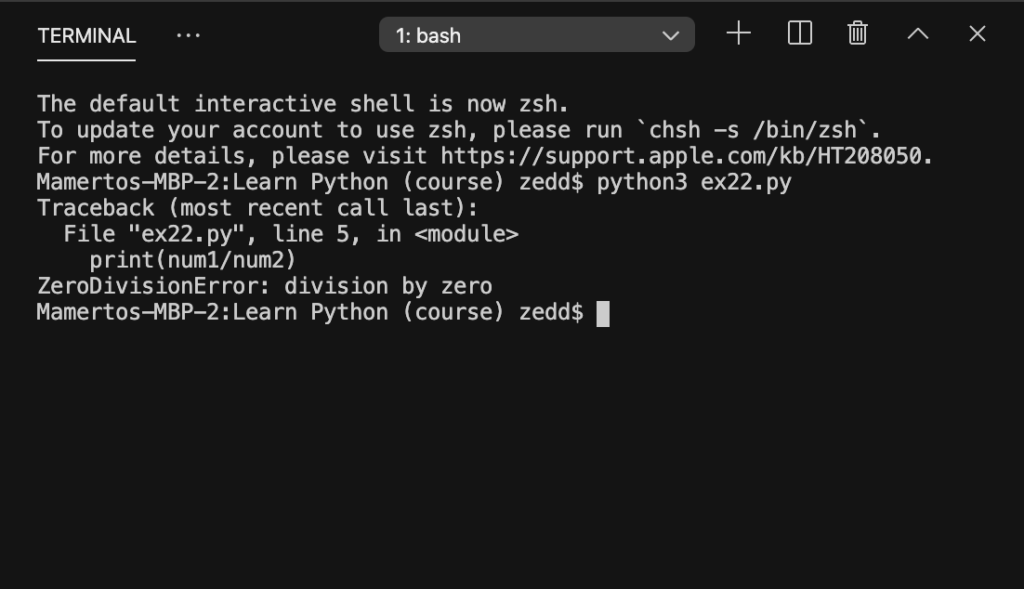
Good. Subsequently, our code produced a ZeroDivisionError exception by trying to divide 7 by 0. Later, I’ll explain why we cannot do this in division. But this time, we’ll do it backwards; let’s divided 0 by 7.
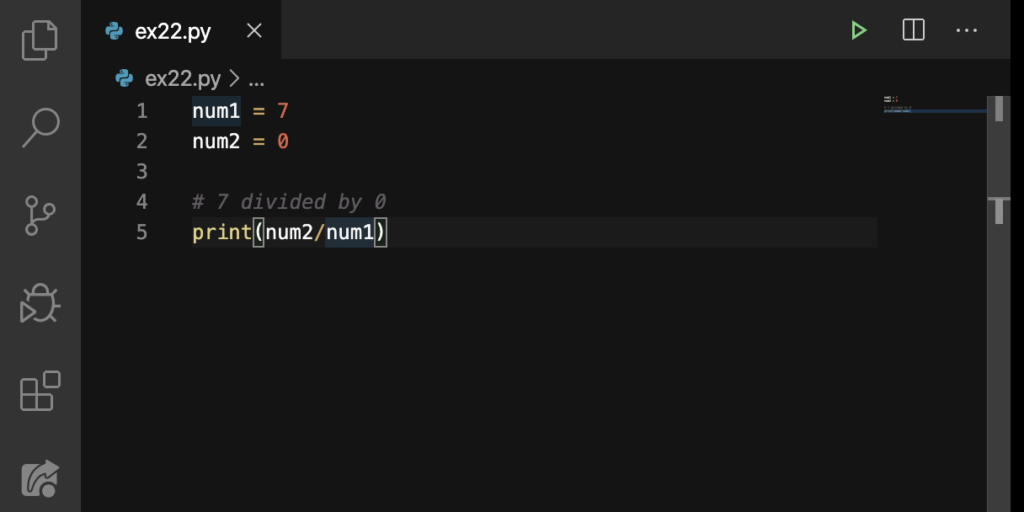
Let’s see what happens now:
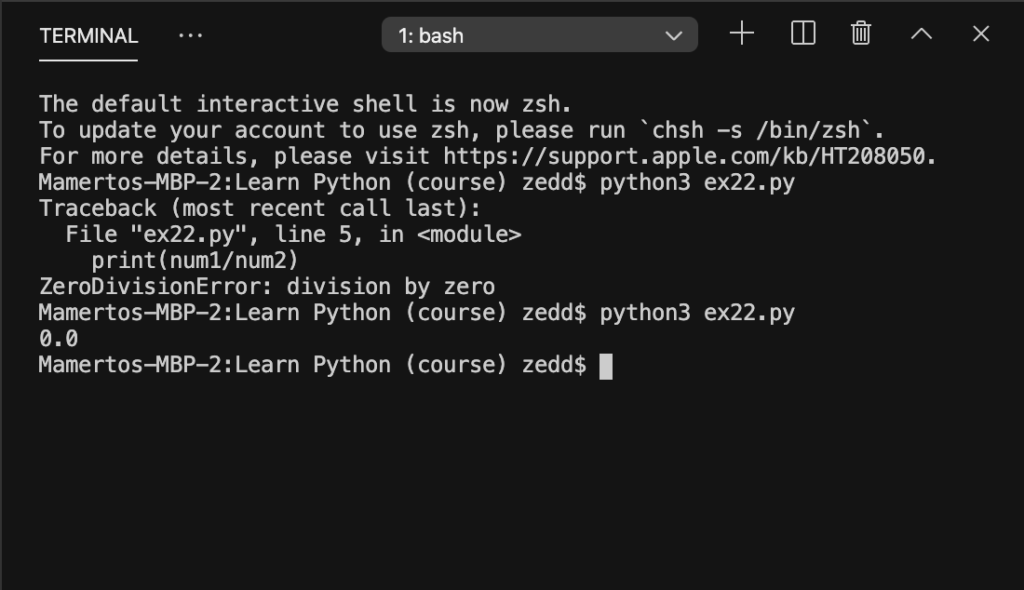
Boom! It worked! There were no problems. But how come 7 divided by 0 was impossible, but 0 divided by 7 was acceptable?
In Math, multiplication is the reverse of division, and vice versa. Thus, when you hvae this equation in division:
7 / 0 = 0.
When we reverse this equation, it will look like this:
0 * 0 = 7.
In math’s terms, multiplying 0 by 0 will be equal to 0. But, can multiplying 0 by 0 be equal to 7? Of course, it won’t work. Therefore, our computer will produce a ZeroDivisionError.
Exceptions in Python Errors
As you well know, different exceptions are raised for different reasons. And these are the common exceptions:
ImportError | an import fails. |
IndexError | a list is indexed with an out-of-range number. |
NameError | an unknown variable is used. |
SyntaxError | the code can’t be parsed properly. |
TypeError | a function is called on a value of an inappropriate type. |
ValueError | a function is called on a value of the correct type, but with an inappropriate value. |
In addition to kinds of errors, there are many other different kinds of errors. And you can check them out in this reference.
Significance of Exceptions in Python
“Why do we have these exceptions anyways? It will just give me a headache seeing that I keep on having errors on everything I do.” Actually, it will ease you from headaches. Why do I say so?
Come to think of it: When our Terminal says ImportError, we immediately know that the error was related to the importing, not on anything else. In that case, we would not look like idiots that will look all day for the cause of the error.
Now, after discussing all these errors, and after knowing their significance to our code, let’s have a short quiz.
Quiz for Exceptions in Python
Analyzing the code below, which exception is going to be raised?
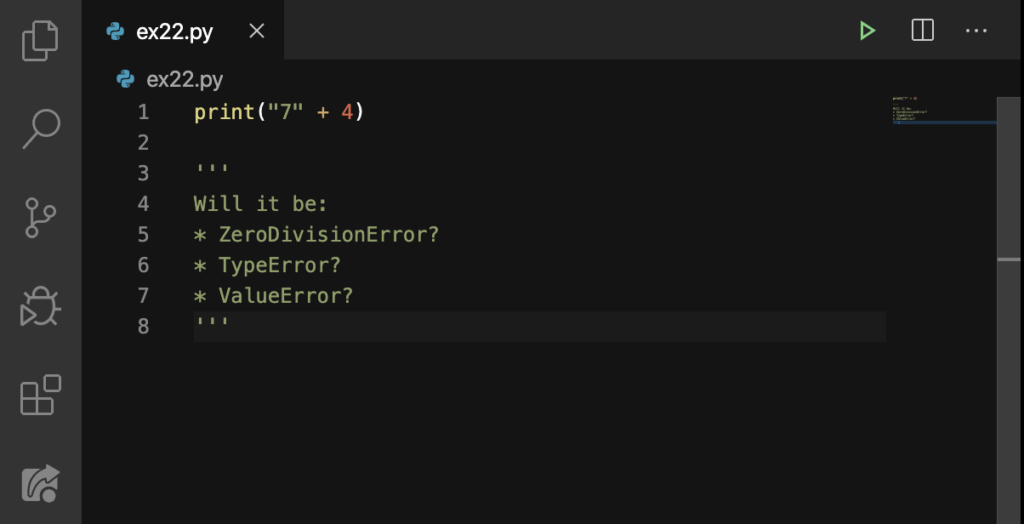
Do not type this on your editor yet. And do not run this until you have an answer. That would be cheating. Share your answers with us on the Comments section below.
That’s it with exceptions in Python. In the next lesson, we’ll discuss how we can use exceptions to have a powerful code. See you there!